How to Fix TypeError: Unhashable Type: Slice in Python
-
TypeError: unhashable type: 'slice'
in Python -
Fix
TypeError: unhashable type: 'slice'
in Python - Conclusion
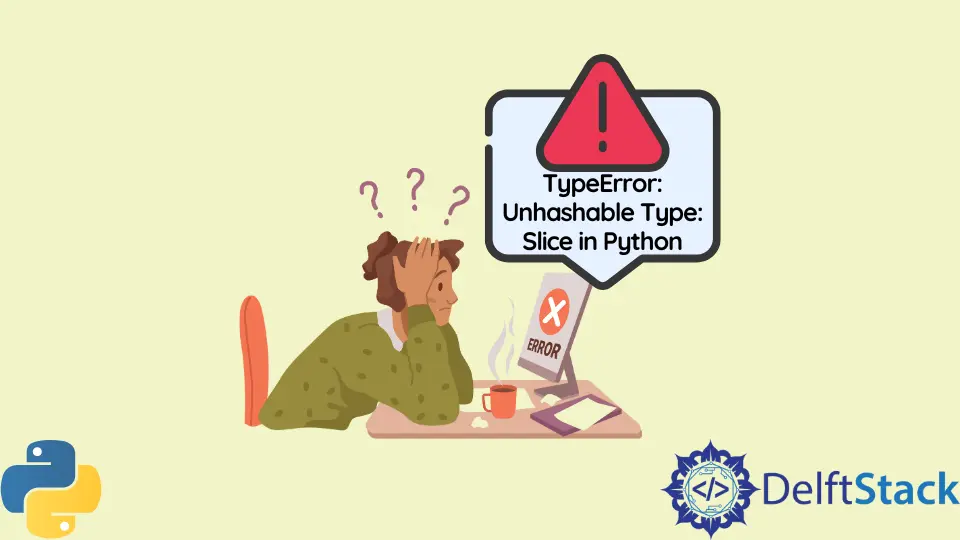
Slicing is a very common technique in Python. It allows us to extract data from a given sequence like a string, list, tuple and more, using the indexes of the elements.
A very simple example of slicing is below.
lst = [2, 5, 6, 7, 3, 1]
print(lst[0:2])
Output:
[2, 5]
The above example extracts a part of the list using the slicing technique. Notice the use of the indexes.
TypeError: unhashable type: 'slice'
in Python
A TypeError
is raised in Python when we try to perform some unsupported operation on a given data type. A TypeError
can also be raised while slicing if we try to use this technique on unsupported data types like a dictionary, DataFrame, and more.
For example:
d = {"a": 10, "b": 11}
d[0:2]
Output:
TypeError: unhashable type: 'slice'
Note the unhashable type: 'slice'
error raised in the above example.
Dictionaries use a hashing function to find values using their respective keys. It does not store the indexes of the values.
Unhashable here means that the slicing operation is not hashable and does not work with dictionaries.
This tutorial will demonstrate how to solve Python’s unhashable type: 'slice'
error.
Fix TypeError: unhashable type: 'slice'
in Python
The most basic fix is to use sequences that support slicing. These include lists, strings, dictionaries, tuples, and other supported sequences.
We can convert the dictionary that does not support slicing to a list and fix this error.
For a dictionary, we can use the values()
and keys()
to get a view object of the values and keys in the dictionary. This object can be converted to a list that supports slicing using the list()
function.
See the code below.
d = {"a": 10, "b": 11}
print(list(d.values())[0:2])
print(list(d.keys())[0:2])
Output:
[10, 11]
['a', 'b']
The above example converts the dictionary into two lists of keys and values. We perform slicing on these lists.
We can also use the items()
function to return a view object of the key and value pairs in a list of tuples.
See the code below.
d = {"a": 10, "b": 11}
print(list(d.items())[0:2])
Output:
[('a', 10), ('b', 11)]
Conclusion
This tutorial demonstrates the unhashable type: 'slice'
error in Python and how to fix it. We first discussed the slicing technique in Python.
We demonstrated how dictionaries and the reason behind them do not support this technique. The fix involves the use of different functions like items()
, keys()
, and values()
that can create a list using the dictionary, which can be further used for slicing.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python