How to Fix Python TypeError: Must Use Keyword Argument for Key Function
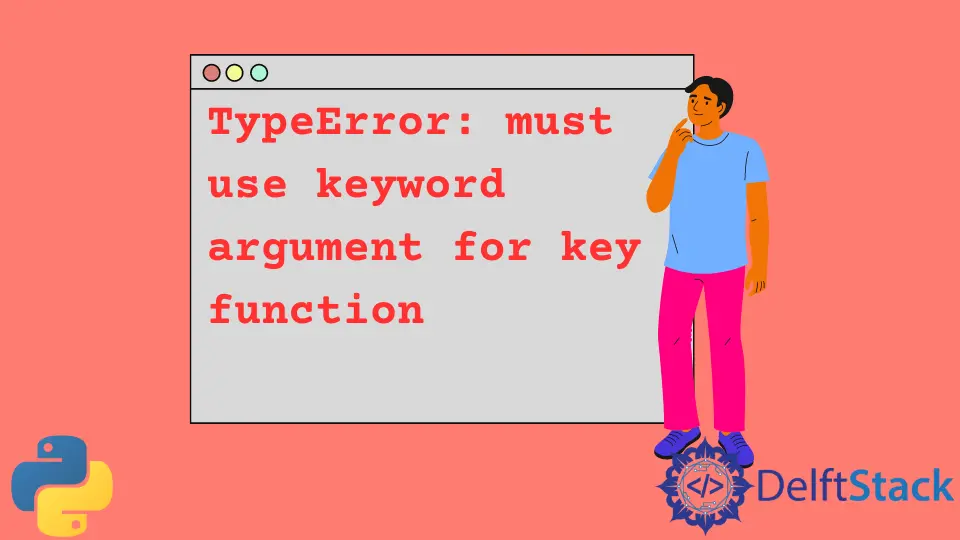
This article tackles the simple and powerful ways to sort a list of items and use the lambda function inside the sort()
method. We also discuss why we failed to execute Python’s sort()
method.
Correct Way to Use Key Argument Inside the sort()
Method in Python
Ordered data lies at the heart of many web services. When we perform a search, the algorithm returns an ordered list of relevant results, and when shopping online, we often want to sort products by price.
When we look at our calendar, we expect our appointments to be sorted in chronological order.
Let’s start with a classic example and sort alphabetically. We’ll use a list of six alkaline earth metals.
They are sorted by atomic number, but what if we wanted to sort them alphabetically? For lists, we call the sort()
method.
The sort()
method assumes you want the data sorted alphabetically, in ascending order. If you want to sort the data in reverse order, you call the sort()
method and specify reverse
equals True
.
Code:
Earth_Metals = ["Berylium", "Magnisium", "Calcium", "strontium", "Barium", "Radium"]
Earth_Metals.sort()
print(Earth_Metals)
If you look at the list, the names are sorted in reverse alphabetical order.
Output:
['Barium', 'Berylium', 'Calcium', 'Magnisium', 'Radium', 'strontium']
Let’s repeat this example, except this time, we store the elements in a tuple rather than a list. When we try to sort a tuple, Python complains and raises an exception.
The reason for this error is that tuples are immutable objects. They cannot be changed, and sorting changes things.
Earth_Metals = ("Berylium", "Magnisium", "Calcium", "strontium", "Barium", "Radium")
Earth_Metals.sort()
print(Earth_Metals)
Output:
AttributeError: 'tuple' object has no attribute 'sort'
Before we see a more complex example, let’s look at the help()
text for the sort
method on lists.
The sort method accepts two keyword arguments, the key
and reverse
. By default, the reverse
is set to false
, which means the data will be sorted in ascending order.
Code:
help(list.sort)
Output:
Let’s focus on the key
argument where the action lies. To use the key argument, you pass in a function that will be used to determine what values to sort by.
Suppose we are building an application for processing orders, and we have this list of order items, and in every item in this list is a tuple with two items the product name and its price. Let’s see what will happen if we sort this list and print the items.
Code:
items = [("product1", 10), ("product2", 9), ("product3", 12)]
items.sort()
print(items)
Output:
[('product1', 10), ('product2', 9), ('product3', 12)]
Nothing changes when we execute the code because Python does not know how to sort this list. In this situation, we need to define Python’s function for sorting lists.
We will make it cleaner by using a lambda expression or an anonymous function, so we do not have to define this function first and then pass it. Instead of this, we add lambda, and the syntax for writing a lambda function is like this we add parameters colon and then expression.
We will have only one parameter; then, after the colon(:
), we are returning an item of one.
Code:
items = [("product1", 10), ("product2", 9), ("product3", 12)]
items.sort(key=lambda item: item[1])
print(items)
Output:
[('product2', 9), ('product1', 10), ('product3', 12)]
Some beginners try to give more than one argument value to the key
argument and get an error because the key
argument only accepts one value. Another reason we failed to execute the code was that we directly passed the function instead of using the key
argument.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python