How to Fix Python TypeError: Function Object Is Not Subscriptable
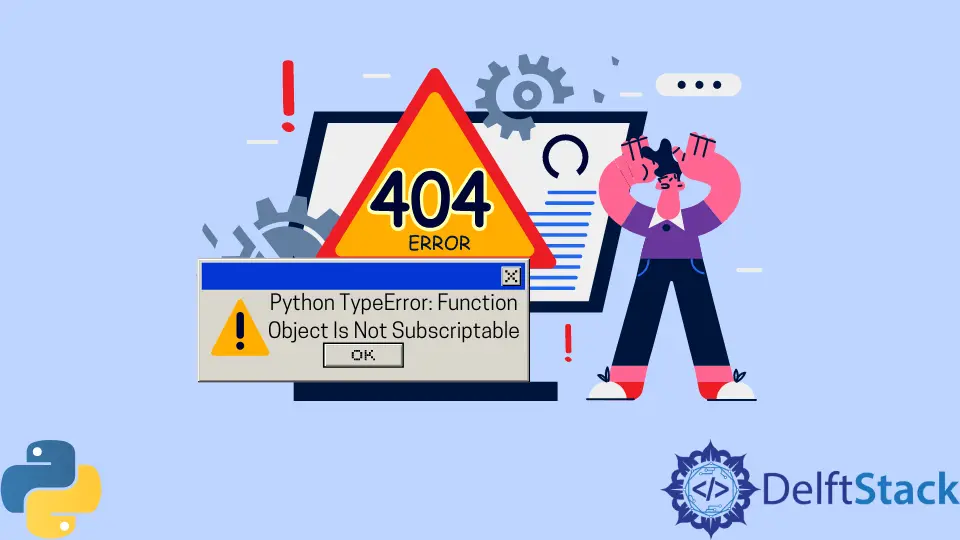
This article discusses why we get an error that is TypeError: 'function' object is not subscriptable
and how we can fix this error in Python.
Fix Function Object TypeError in Python
If you’re a beginner in programming, you may encounter errors in running a program. Sometimes you try to fix this error but find it hard because you are not trying to understand why this error occurs during execution.
For example, suppose we have a list of employees and want to wrap it inside the function to reuse it later inside the class or module. When we call this function, we get an error that says TypeError: 'function' object is not subscriptable
.
Example Code:
Employee_Name = ["Max", "Harry", "Ben", "Simon", "Thomas"]
def Employee_Name(n):
for i in range(5):
print(n[i])
Employee_Name(Employee_Name)
Output:
TypeError: 'function' object is not subscriptable
In the above Python script, we loop the list of items, and the error occurs because we pass a function inside the function instead of a list.
The n
variable contains a function, and inside the loop, when we try to access an index of the n
variable, we get the error because we can not access an index from an object that is not subscriptable or iterable.
Beginners tend to fix it, but others may get a different error, like in the following output.
Example Code:
def Employee_Name(n):
for i in range(5):
print(n[i])
Employee_Name = ["Max", "Harry", "Ben", "Simon", "Thomas"]
Employee_Name(Employee_Name)
Output:
TypeError: 'list' object is not callable
Thinking that the error can be fixed if a list is declared after defining the function even though the function and list have the same name. After running the code, the user gets another error.
The reason is that during a variable declaration, the Employee_Name
function name is overridden with a variable that is a list, and we can not call a list. The simple solution is never to use the same name with another object you have used for a function.
Example Code:
def Employee_Name(n):
for i in range(5):
print(n[i])
Employee_Names = ["Max", "Harry", "Ben", "Simon", "Thomas"]
Employee_Name(Employee_Names)
We changed the variable name Employee_Name
to Employee_Names
.
Output:
Max
Harry
Ben
Simon
Thomas
Fix Function Object TypeError With Empty List in Python
Another reason is to get the TypeError: 'function' object is not subscriptable
when we try to make an empty list of the list for any reason. Let’s look at an example.
Example Code:
Empty_List = []
def Employee_Name(n):
for i in range(5):
Empty_List.append(n[i])
return Empty_List
Employee_Names = ["Max", "Harry", "Ben", "Simon", "Thomas"]
print(Employee_Name[Employee_Names])
Output:
TypeError: 'function' object is not subscriptable
When we call the function, we must put the opening and closing function brackets((function)
) in the function name. Suppose we put square brackets([function]
) in it, which is against the behavior of a function for calling it using square brackets.
Some users make another mistake because they want a list of a list. When they try to fix that inside the opening and closing brackets, they pass a list within square brackets and get another error.
Example Error:
Empty_List = []
def Employee_Name(n):
for i in range(5):
Empty_List.append(n[i])
return Empty_List
Employee_Names = ["Max", "Harry", "Ben", "Simon", "Thomas"]
print(Employee_Name([Employee_Names]))
Output:
IndexError: list index out of range
One reason for getting this error is passing a list of a list with 1
length, and its index goes out of range when we iterate it inside the for
loop. The simple solution is instead of using square brackets inside the function ([Employee_Names]
), we need to put square brackets within the Empty_List
variable where we are returning it.
Example Code:
Empty_List = []
def Employee_Name(n):
for i in range(5):
Empty_List.append(n[i])
return [Empty_List]
Employee_Names = ["Max", "Harry", "Ben", "Simon", "Thomas"]
print(Employee_Name(Employee_Names))
Output:
[['Max', 'Harry', 'Ben', 'Simon', 'Thomas']]
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python