How to Fix TypeError: Cannot Convert the Series to <Class 'Float'> in Python
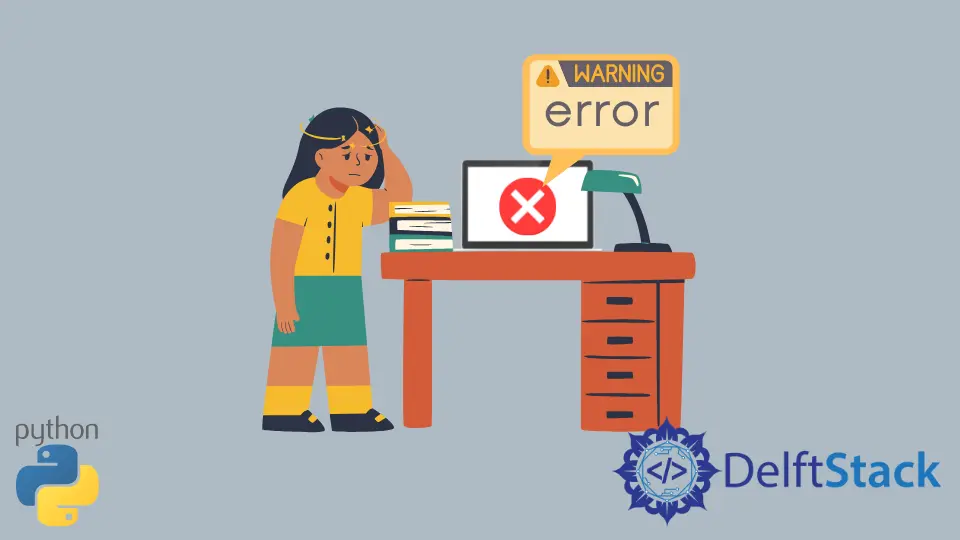
With this explanation, we will learn why we get the error TypeError: cannot convert the series to <class 'float'>
. We will also learn how to fix it and change the data type of a Pandas series in Python.
Convert Data Type of a Pandas Series Without Error in Python
Let’s jump with an example data set. We will import the Pandas library and then the data set; we will start with alcohol consumption by country.
We will declare a variable called Alcohol
and read the CSV file from http://bit.ly/drinksbycountry
.
After executing the following code, we will look at the head()
, and we should see six columns and four rows numeric.
import pandas as pd
Alcohol = pd.read_csv("http://bit.ly/drinksbycountry")
Alcohol.head()
Output:
Let’s look at the data types of these columns. We will use the dtypes
attribute of the Alcohol
data frame to find the data types.
Alcohol.dtypes
Now we will see three of our columns are integer columns, we have one floating-point column, and then two columns are objects, which means country
and continent
are just strings.
Output:
Suppose we want to convert the spirit_servings
column to a floating point rather than an integer. All we have to do is use the series method astype()
and pass it float
, which means we convert it to the floating-point type.
If we want to modify the data frame, you can add a new column or overwrite the existing spirit_servings
column. If we define dtypes
to check the data types of columns, we see that it has changed, and spirit_servings
is now a floating-point column.
Alcohol["spirit_servings"] = Alcohol.spirit_servings.astype(float)
Alcohol.dtypes
Output:
Now you might be wondering what the use of that is. You usually do this when you have a data file where the numbers are stored as strings and have read it into Pandas strings but want to do the Math on them.
To do the Math on a column, it has to be a numeric type, so your column type should be a float which is the more common use case. If you do not convert it into the float type, you will get an error that would be something like this TypeError: cannot convert the series to <class 'float'>
.
One more question arises: how did it find the type of each column before actually reading the CSV file? Now we will change the data types during the CSV reading process; we will have to add one more parameter, dtype
.
We pass it to a dictionary; the dictionary key is spirit_servings
, and the dictionary value is a float. Now, if we check this column’s data type, we see that, once again, spirit_servings
has been converted to a floating point.
Alcohol = pd.read_csv(
"http://bit.ly/drinksbycountry", dtype={"spirit_servings": "float"}
)
Alcohol.dtypes
Output:
The only difference between this and the above is that this method does it during the reading process. In the above, we converted it after the data frame had already been created.
Another Example of Converting Data Type of a Pandas Series Without Error in Python
Now we will show you one more data set and another example using the chip orders data.
CHIP_ORDERS = pd.read_table("http://bit.ly/chiporders")
CHIP_ORDERS.head()
Let’s look at the column. We want to focus on the item_price
column.
CHIP_ORDERS.dtypes
Pandas is storing the item_price
column as an object, meaning a string, because it does not understand that these are numbers.
To do some Math with it, we must remove a character from a series like the $
sign. We can use the str
and replace()
methods to replace the dollar sign with nothing, but it is not enough; if we try to apply a Mathematical operation to the series, it will give you an error.
CHIP_ORDERS.item_price.str.replace("$", "").mean()
Output:
ValueError: could not convert string to float: '2.39 3.39 3.39...
This is because even though we removed the dollar sign, it is still a string, so we must typecast it to a float. After we typecast it to a float, we can do a Mathematical operation on it.
CHIP_ORDERS.item_price.str.replace("$", "").astype(float).mean()
Output:
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python