How to Convert a String to List in Python
-
Convert String to List in Python Using the
list()
Function -
Convert String to List in Python Using the
split()
Method -
Convert String to List in Python Using the
eval()
Function -
Convert String to List in Python Using the
ast
Method
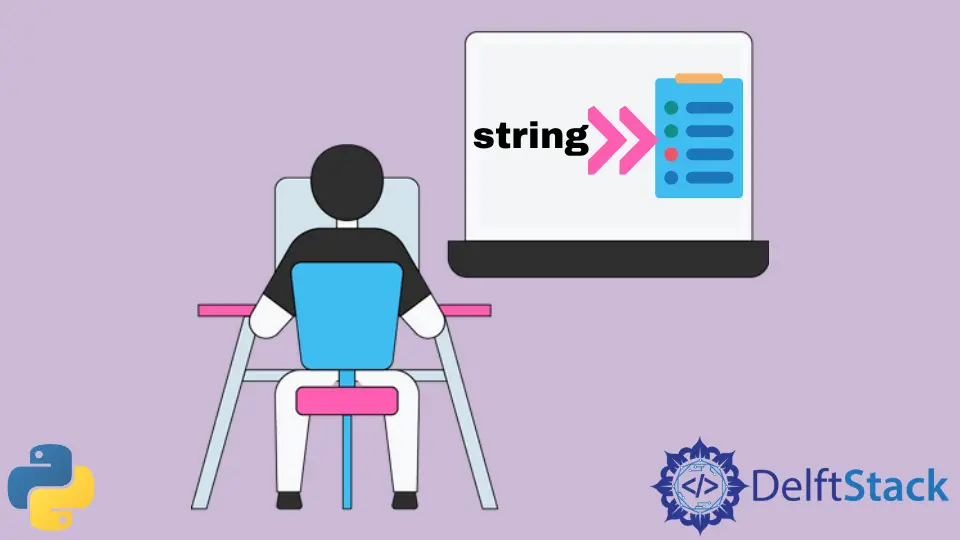
Python list is mutable; so that its elements can be overwritten, modified, and deleted at any time during the program execution. The string is a combination of characters enclosed in single or double quotes, and it is not mutable.
In this article, we will demonstrate different methods to convert a string into a list in Python.
Convert String to List in Python Using the list()
Function
The list()
function converts the string into a list in Python.
Let’s take the below code as an example:
test_str = "Python"
list(test_str)
Output:
['P', 'y', 't', 'h', 'o', 'n']
The list()
function takes a string as an argument and converts it into a list. Every character in the string becomes the individual element in the list.
Convert String to List in Python Using the split()
Method
Let’s take a string in comma-separated values.
string = "x,y,z"
Now we split the string values into the list elements.
The complete example code is as follows:
string = "x,y,z"
output = string.split(",")
print(output)
Output:
['x', 'y', 'z']
The above example splits the string at the given delimiter, ,
, to a list. If the delimiter is not given, it uses the white space as the default value.
Convert String to List in Python Using the eval()
Function
This method will only convert the string into a list when the string is safe; it means that the string in the eval()
function is parsed and determined as a Python expression. If it couldn’t be evaluated as a Python expression, it will generate an error. It is not a pythonic method. We could use split()
or ast
methods instead of this.
Let’s take a specified string for this method.
string = "['xxx','yyy','zzz']"
The complete example code is as follows:
string = "['xxx','yyy','zzz']"
output = eval(string)
print(output)
Output:
['xxx', 'yyy', 'zzz']
Convert String to List in Python Using the ast
Method
This method will use the ast
library (Abstract Syntax Trees). With this library, we will use the eval()
function to convert a string to a list.
The complete example code is as follows:
import ast
string = "['xxx','yyy','zzz']"
output = ast.literal_eval(string)
print(output)
Output:
['xxx', 'yyy', 'zzz']
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python