How to Fix ValueError: Setting an Array Element With a Sequence in Python
- Numpy Array in Python
- Create a Numpy Array in Python
-
Causes of the
ValueError: setting an array element with a sequence
Error in Python -
Fix the
ValueError: setting an array element with a sequence
Error in Python
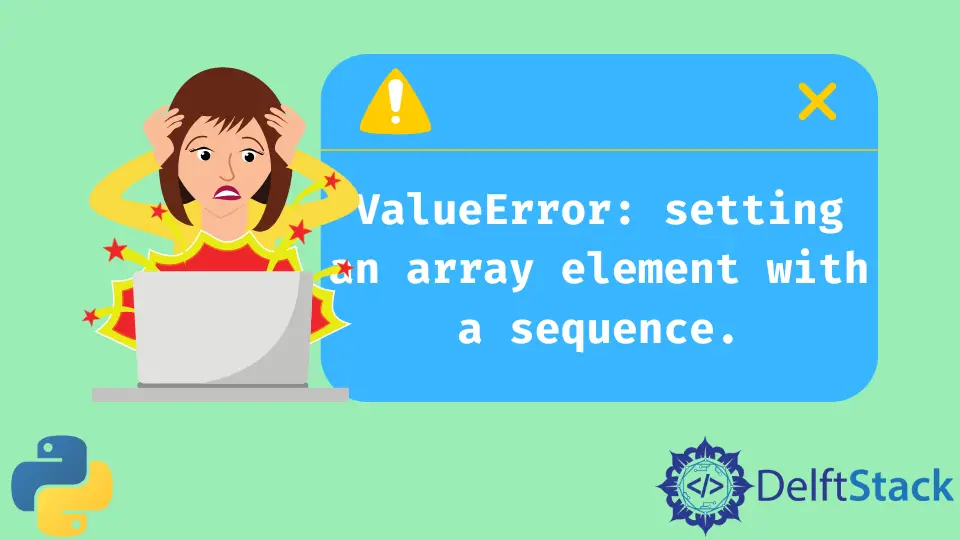
In Python, the array is one of the most common and useful data structures, a collection of more than one value. The elements of the array are accessed through the indices, which are the element’s location.
You might have somehow interacted with the error ValueError: setting an array element with a sequence
at some point. This ValueError mainly occurs in two scenarios; the first is when you try to assign multiple values to a single array index, and the second is when you try to assign an invalid data type to the defined array.
As we know, arrays are homogenous in nature. They accept a single data type throughout the array.
Numpy Array in Python
Numpy is an open source library which is most used for metrics, linear algebra, Fourier transform, etc. It was created by Travis Oliphant in 2005.
In Python, we have Lists that provide us with the purpose of arrays, but the Numpy creators claim that they prove 50x faster arrays than Lists. This is one of the core purposes of using the Numpy array.
Create a Numpy Array in Python
The syntax of the Numpy array is very simple. You must import the numpy
library to your program and use it accordingly.
# import numpy library
import numpy as np
# creating a numpy array
arr = np.array([1, 2, 4, 5, 6])
print(arr)
Output:
[1 2 4 5 6]
We can also convert a conventional array to a Numpy array.
# import numpy library
import numpy as np
conventional_array = [1, 2, 3, 4, 5]
print("The data type conventional array is: ", type(conventional_array))
# converting a conventional array into a numpy array
arr = np.array(conventional_array)
print("The data type of numpy array is: ", type(arr))
print(arr)
Output:
The data type conventional array is: <class 'list'>
The data type of numpy array is: <class 'numpy.ndarray'>
[1 2 3 4 5]
The type of the conventional array belongs to the list
class, whereas after converting it into a Numpy array, it now belongs to the numpy.ndarray
class.
Causes of the ValueError: setting an array element with a sequence
Error in Python
As discussed, the ValueError: setting an array element with a sequence
error occurs in Python when you try assigning more than one value to a single position or improper data types to an array. As we know, arrays are homogenous data structures that cannot store multiple data types on a single array.
Let’s understand both of these scenarios through examples.
# import numpy library
import numpy as np
arr = [1, 2, 3, 4, 5, 6, 7]
numpy_arr = np.array(arr)
# print the element at 0 index, which is the first element
print("The first element of the array: ", numpy_arr[0])
# assign two values (1,2) to the 0th index --> Error
numpy_arr[0] = 1, 2
Output:
The first element of the array: 1
ValueError: setting an array element with a sequence.
As you can see in the last code of the above program, when we try to assign two values 1,2
to the 0
index of the numpy_arr
, we get the error ValueError: setting an array element with a sequence
.
This is because we can only store a single value in an index. However, we can replace it with another value so that a single index will point to an individual value.
Now, let’s have a look at the second scenario.
# import numpy library
import numpy as np
# Creating an array
array1 = [1, 2, [0, 0], 4, 5]
print(array1)
print("The data type of the conventional array is: ", type(array1))
# This causes Value error
np_array = np.array(array1, int)
print("\n", np_array)
print("The data type of of numpy array is: ", type(np_array))
Output:
[1, 2, [0, 0], 4, 5]
The data type of the conventional array is: <class 'list'>
ValueError: setting an array element with a sequence.
In this case, the data type we assigned to the numpy array
is responsible for this error. In this statement np_array = np.array(array1, int)
, the second parameter int is causing this error.
As you can see, the class of the array1
is a list, so it isn’t possible to convert it into int straightforwardly.
Fix the ValueError: setting an array element with a sequence
Error in Python
We have now seen in the above examples what causes the ValueError. Let’s take a look and understand how to fix this ValueError.
The first scenario is very simple. You only assign one value to a single index.
# import numpy library
import numpy as np
# creating a numpy array
numpy_arr = np.array([1, 2, 3, 4, 5])
print(numpy_arr)
# assigning 0 value at the 0th index
numpy_arr[0] = 0
print(numpy_arr)
Output:
[1 2 3 4 5]
[0 2 3 4 5]
In the second scenario, we can use a universal data type accepted in every case, object
. Let’s see it in the example below.
# import numpy library
import numpy as np
# creating an array
arr = [1, 2, [9, 8], 3, 4]
# This causes Value error
np_arr = np.array(arr, object)
print(np_arr)
Output:
[1 2 list([9, 8]) 3 4]
As you can see, after we changed the data type from int to object, the program ran smoothly. Because object
is the universal data type, it treats every piece of the array as an object, whether it is a list or a single int value.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python