How to Add Selenium Web Driver Wait in Python
- Python Selenium Web Driver Wait
- Implicit Wait in Selenium Web Driver
- Explicit Wait in Selenium Web Driver
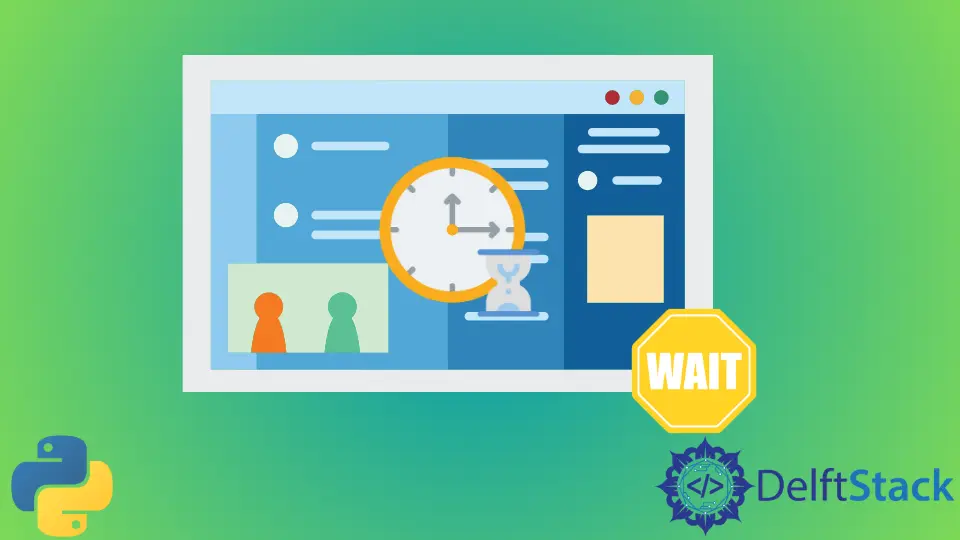
This article will introduce examples of adding a wait in Selenium web driver in Python.
Python Selenium Web Driver Wait
Most web applications use AJAX techniques. Due to this, different elements present on a web page take different time intervals to get completely uploaded since selenium cannot find any text until it is present on the web page.
We may find that particular text is not present on a web page of some application before it gets uploaded. This creates errors while testing an application.
This issue can get solved by involving waits. These waits offer flexibility while searching for an element or any other operation on a web page.
The selenium web driver provides two types of waits. The first one is implicit wait, and the second one is explicit wait.
The detail of these two types of waits is discussed in this article.
Implicit Wait in Selenium Web Driver
Due to slow internet or slow response from a website, there may be situations where we have to wait for a few seconds to get all the results. For this case, the implicit wait works best.
An implicit wait tells the web driver to poll the DOM for a specific time while finding elements to make all the elements available when our web driver fetches the element. The default setting for the process is 0
.
Once we have set the implicit wait, it will be for the lifespan of the web driver object.
It will be for the same amount as long as we run the same web driver without closing or restarting and changing the wait value.
Let’s go through an example in which we will go to a website and wait for 10 seconds, and after that, we will click on the About Us
page link, as shown below.
Example code:
# python
from selenium import webdriver
ChromeDriver = webdriver.Chrome()
ChromeDriver.implicitly_wait(10)
ChromeDriver.get("https://www.inventicosolutions.com/")
getElemByLink = ChromeDriver.find_element_by_link_text("About Us")
getElemByLink.click()
Output:
As you can see from the above example, it waits for exactly 10 seconds after loading the page before going to the About Us
page.
Explicit Wait in Selenium Web Driver
An explicit wait is a type of code defined to wait for a specific condition to occur and proceed further in the code. The extreme case of explicit wait is time.sleep()
.
This sets the condition, for the wait, to an exact time period.
Some appropriate methods help us to write an explicit code. Explicit waits are achieved using the web driver wait class and combined with expected_conditions
.
As shown below, let’s go through an example in which we will use an explicit wait instead of an implicit wait.
Example code:
# python
from selenium import webdriver
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.common.by import By
from selenium.webdriver.support import expected_conditions as ExpectedCond
chromeDriver = webdriver.Chrome()
chromeDriver.get("https://inventicosolutions.com")
getElembyLinkText = WebDriverWait(chromeDriver, 10).until(
ExpectedCond.presence_of_element_located((By.LINK_TEXT, "About Us"))
)
getElembyLinkText.click()
Output:
The timeout value is 10 seconds for the above-written code. The web driver will wait 10 seconds before throwing a Timeout Exception
.
The application’s web page address (URL) and text element can be written in the given code as per requirement.
The web driver wait (one of the tools being used in explicit waits) has this built-in quality to check for the Expected Condition
after every 500 milliseconds until the text is found successfully.
Expected Conditions for Wait in Python
These conditions are most frequently used while automating the web browser and are pre-defined in web driver. These conditions include:
title_is
title_contains
presence_of_element_located
visibility_of_element_located
visibility_of
presence_of_all_elements_located
text_to_be_present_in_element
text_to_be_present_in_element_value
frame_to_be_available_and_switch_to_it
invisibility_of_element_located
element_to_be_clickable
staleness_of
element_to_be_selected
element_located_to_be_selected
element_selection_state_to_be
element_located_selection_state_to_be
alert_is_present
We can include these expected conditions in our code by importing the following libraries.
# python
from selenium.webdriver.support import expected_conditions as ExpectedCond
Custom Wait Conditions in Python
Custom wait conditions can be created when none of the above methods meets the requirements of a user. A custom condition is created using a class with the call()
method.
As shown below, let’s go through an example in which we will create a custom condition and try to make our program wait for a few seconds.
Example code:
# python
class ElementWithClass(object):
def __init__(self, location, className):
self.location = location
self.className = className
def __call__(self, ChromeDriver):
findElem = ChromeDriver.find_element(*self.location)
if self.className in findElem.get_attribute("class"):
return findElem
else:
return False
wait = WebDriverWait(ChromeDriver, 10)
findElem = wait.until(element_has_css_class((By.ID, "myId"), "myClass"))
This code will give the user a true
if the element is found and returns a false
otherwise.
So we have discussed different methods to add waits in Python with some examples. We hope you enjoyed this topic and learned something from it.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedInRelated Article - Python Selenium
- How to Check if Element Exists Using Selenium Python
- How to Refresh Page in Python Selenium
- WebDriverException: Message: Geckodriver Executable Needs to Be in PATH Error in Python
- How to Install Python Selenium in macOS
- How to Login to a Website Using Selenium Python
- How to Open and Close Tabs in a Browser Using Selenium Python