How to Save a Dictionary to a File in Python
-
Save a Dictionary to File in Python Using the
dump
Function of thepickle
Module -
Save a Dictionary to File in Python Using the
save
Function ofNumPy
Library -
Save a Dictionary to File in Python Using the
dump
Function of thejson
Module
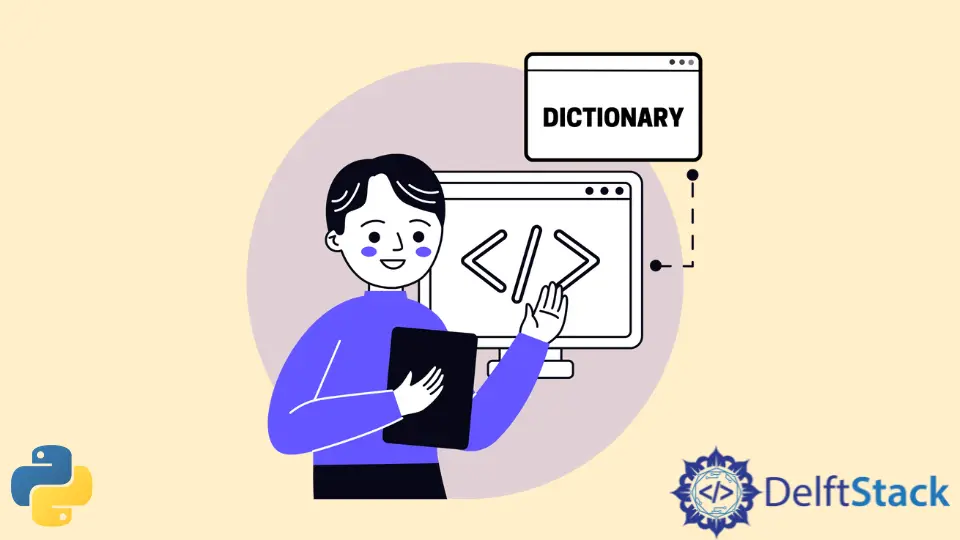
This tutorial explains multiple methods to save a dictionary to a file in Python language. Methods include:
dump()
function ofpickle
module of Pythonsave()
function ofNumPy
librarydump()
function of Pythonjson
module.
Save a Dictionary to File in Python Using the dump
Function of the pickle
Module
The code example below shows how we can use the dump()
function of the pickle
module to save the dictionary and read a dictionary from the saved file using the load()
function. The dump()
function of the pickle
module needs the dictionary which we want to save, and the file object as parameters to save the dictionary as a .pkl
file.
import pickle
my_dict = {"Apple": 4, "Banana": 2, "Orange": 6, "Grapes": 11}
with open("myDictionary.pkl", "wb") as tf:
pickle.dump(my_dict, tf)
The below code example shows how to read the dictionary saved in a file, using the load()
function. The load()
function needs a file object as a parameter to load the dictionary from the .pkl
file.
import pickle
with open("myDictionary.pkl", "wb") as tf:
new_dict = pickle.load(tf)
print(new_dict)
Output:
{ 'Apple': 4, 'Banana': 2, 'Orange': 6, 'Grapes': 11}
Save a Dictionary to File in Python Using the save
Function of NumPy
Library
The save()
function of the NumPy
library can also save a dictionary in a file. In order to save the dictionary as a .npy
file, the save()
function requires the file name and dictionary which we want to save, as parameters to save the dictionary to a file.
Code example:
import numpy as np
my_dict = {"Apple": 4, "Banana": 2, "Orange": 6, "Grapes": 11}
np.save("file.npy", my_dict)
The code example shows how to read the Python dictionary saved as .npy
file. The load()
function of NumPy
library requires the file name and need to set allow_pickle
parameter as True
to load the saved dictionary from .npy
file.
Code example:
import numpy as np
new_dict = np.load("file.npy", allow_pickle="TRUE")
print(new_dict.item())
Save a Dictionary to File in Python Using the dump
Function of the json
Module
Another method to save a dictionary to file in Python is to use the dump()
function of the json
module. It also needs dict
variable which we want to save, and file object as parameters to save the dictionary as .json
file
Example code:
import json
my_dict = {"Apple": 4, "Banana": 2, "Orange": 6, "Grapes": 11}
tf = open("myDictionary.json", "w")
json.dump(my_dict, tf)
tf.close()
Code example to read the dictionary saved as a file using the load
function of the json
module is shown below. The load()
function needs file object as parameter to load the dictionary from the .json
file.
import json
tf = open("myDictionary.json", "r")
new_dict = json.load(tf)
print(new_dict)
Related Article - Python Dictionary
- How to Check if a Key Exists in a Dictionary in Python
- How to Convert a Dictionary to a List in Python
- How to Get All the Files of a Directory
- How to Find Maximum Value in Python Dictionary
- How to Sort a Python Dictionary by Value
- How to Merge Two Dictionaries in Python 2 and 3