How to Replace a Line in a File in Python
-
Use the
for
Loop Along With thereplace()
Function to Replace a Line in a File in Python - Create a New File With the Refreshed Contents and Replace the Original File in Python
-
Use the
fileinput.input()
Function for Replacing the Text in a Line in Python -
Use the
re
Module to Replace the Text in a Line in Python -
Use the
file.seek()
andfile.write()
Functions to Replace the Text in a Line in Python - Conclusion
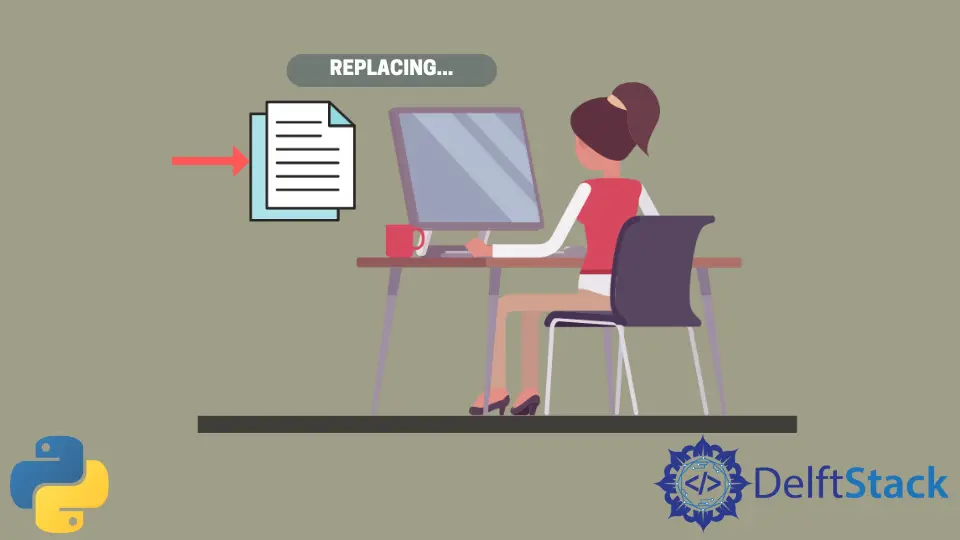
File handling is considered an essential aspect of any web application. Similar to most programming languages, Python is perfectly capable of supporting file handling.
It allows you to deal with files of different types, essentially to perform some basic operations like reading and writing. It also provides some other file handling options to operate the files.
This tutorial demonstrates the different methods available to replace a line in a file in Python.
To demonstrate the different ways to replace a line in a file in this article, we will take a text file, motivation.txt
.
Text file (motivation.txt
):
There are always second chances in life. Anybody can bounce back from hardships. The hardships that we face are just a test to check our will. A strong will is all it takes to bounce back from a loss.
The path of the storage directory of this file might be different in the reader’s computer; therefore, it is advised to adjust accordingly.
Use the for
Loop Along With the replace()
Function to Replace a Line in a File in Python
The open()
function is utilized to open a file in Python. The file can be opened in either the format of text or binary, which depends on the programmer.
The open()
function has various modes, and all of them provide different accessibility options to open a file. This method is particularly useful for targeted text replacements where you know the exact string you wish to change.
The following code uses the for
loop along with the replace()
function.
def replace_text_in_file(filename, old_text, new_text):
with open(filename, "r") as file:
lines = file.readlines()
with open(filename, "w") as file:
for line in lines:
file.write(line.replace(old_text, new_text))
# Replace "hardships" with "situations" in "motivation.txt"
replace_text_in_file("motivation.txt", "hardships", "situations")
In this code, we first open the file in read mode and read all lines into the lines
list. Then, we reopen the file in write mode.
Using a for
loop, we iterate through each line in the lines
list, apply the replace()
function to substitute hardships
with situations
, and write the updated line back to the file. This approach ensures that only the specified word is changed, leaving the rest of the file’s content intact.
Output:
Create a New File With the Refreshed Contents and Replace the Original File in Python
Several functions are at work to implement this process. The knowledge of the process of all these functions is necessary to execute this method successfully.
We need to import several functions from three modules to the Python code.
All these modules need to be imported into the current Python code to run the code without any errors. An example code that implements this way is as follows.
from tempfile import mkstemp
from shutil import move, copymode
from os import fdopen, remove
def replacement(filepath, old_text, new_text):
# Creating a temp file
fd, abspath = mkstemp()
with fdopen(fd, "w") as temp_file:
with open(filepath, "r") as original_file:
for line in original_file:
temp_file.write(line.replace(old_text, new_text))
copymode(filepath, abspath)
remove(filepath)
move(abspath, filepath)
Initially, we invoke mkstemp()
to create a temporary file, which provides us with a file descriptor (fd
) and an absolute path (abspath
) to this new file. Using fdopen(fd, "w")
, we open this temporary file for writing, ensuring that we’re working on a fresh, isolated copy.
As we loop through each line of the original file with open(filepath, "r")
, we apply the replace(old_text, new_text)
function to substitute hardships
with situations
. These modified lines are then written directly into our temporary file.
Following the text replacement, copymode(filepath, abspath)
is employed to mirror the original file’s permissions onto the temporary file, preserving important metadata. Finally, we use remove(filepath)
to safely delete the old file and move(abspath, filepath)
to replace it with our updated temporary file, now containing the revised content.
Output:
Use the fileinput.input()
Function for Replacing the Text in a Line in Python
The fileinput.input()
method gets the file as the input line by line and is mainly utilized for appending and updating the data in the given file.
The fileinput
and sys
modules need to be imported to the current Python code in order to run the code without any errors. The following code uses the fileinput.input()
function for replacing the text in a line in Python.
import fileinput
file_path = "motivation.txt"
# Using fileinput to modify the file in-place
for line in fileinput.input(file_path, inplace=True):
# Replace 'hardships' with 'situations' and print the line
print(line.replace("hardships", "situations"), end="")
# Displaying the updated content
with open(file_path, "r") as file:
print(file.read())
In our approach, we first import the fileinput
module. Using fileinput.input(file_path, inplace=True)
, we iterate over each line of the file specified in file_path
.
The key here is the inplace=True
parameter, which allows us to modify the file directly. During this iteration, we apply the replace()
method to each line to substitute hardships
with situations
.
The print()
function with end=''
is used to write back the modified lines to the file. This is because, with inplace=True
, the standard output (print
) is redirected to the file.
After completing the loop, we reopen the file in read mode to display its updated content. This demonstrates how fileinput
simplifies in-place file editing by handling the complexities of file reading and writing seamlessly.
Output:
Use the re
Module to Replace the Text in a Line in Python
The re
module is a built-in module that Python provides to programmers that deal with Regular Expression, and it needs to be imported into the code. It helps in performing the task of searching for a pattern in a given particular string.
In this method, we use two functions, namely, re.compile()
and re.escape()
, from the RegEx
module.
The following code uses several functions from the Regex
module to replace the text in a line in Python.
import re
file_path = "motivation.txt"
# Read the original content
with open(file_path, "r") as file:
content = file.read()
# Replace 'hardships' with 'situations' using re.sub
updated_content = re.sub("hardships", "situations", content)
# Write the updated content back to the file
with open(file_path, "w") as file:
file.write(updated_content)
# Display the updated content
print(updated_content)
In this example, we start by importing the re
module. We then open motivation.txt
and read its content into the variable content
.
The re.sub()
function is used to replace occurrences of hardships
with situations
in content
. The re.sub()
function is powerful because it allows for complex pattern matching and replacement, although in this simple case, it behaves similarly to the string replace()
method.
After the replacement, we open motivation.txt
again in write mode and write the updated content back to it. Finally, we print the updated content to confirm the changes.
Output:
Use the file.seek()
and file.write()
Functions to Replace the Text in a Line in Python
In Python, file.seek()
and file.write()
are fundamental methods for manipulating file content. file.seek()
sets the file’s current position, while file.write()
is used to write data to the file.
This method is particularly useful when you need to update or replace specific parts of a file without rewriting the entire content. It’s often used in situations where the modifications are minor and localized, such as updating configuration files, correcting specific data in log files, or performing in-place text replacements.
file_path = "motivation.txt"
# Read the content and replace the specific string
with open(file_path, "r+") as file:
content = file.read()
updated_content = content.replace("hardships", "situations")
# Move back to the start of the file and write the updated content
file.seek(0)
file.write(updated_content)
file.truncate()
# Display the updated content
with open(file_path, "r") as file:
print(file.read())
In this code, we open motivation.txt
in r+
mode, which allows both reading and writing. We read the entire content of the file and perform the replacement using the replace()
method.
After replacing hardships
with situations
, we use file.seek(0)
to move the file pointer back to the start of the file. Then, file.write(updated_content)
is used to overwrite the file with the new content.
We also call file.truncate()
to remove any remaining part of the old content if the new content is shorter than the old one. Finally, we reopen the file in read mode to print the updated content.
Output:
Conclusion
In this comprehensive guide, we explored various Python techniques for replacing text in a file, specifically targeting the word hardships
in motivation.txt
. Our journey began with the for
loop combined with replace()
, a straightforward method ideal for simple text substitutions.
We then advanced to creating a new file with updated content, a robust approach leveraging modules like tempfile
, shutil
, and os
for intricate file manipulations. Next, the fileinput.input()
function showcased its prowess in in-place text editing, streamlining the update process.
We further delved into the re
module, harnessing the power of regular expressions for more complex pattern-based replacements. Finally, we examined the file.seek()
and file.write()
methods, which excel in precision edits without the need to rewrite entire file contents.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn