在 Python 中替換檔案中的一行
-
在 Python 中使用
for
迴圈和replace()
函式替換檔案中的一行 - 在 Python 中用更新的內容建立一個新檔案並替換原始檔案
-
在 Python 中使用
fileinput.input()
函式替換一行中的文字 -
在 Python 中使用
re
模組替換一行中的文字
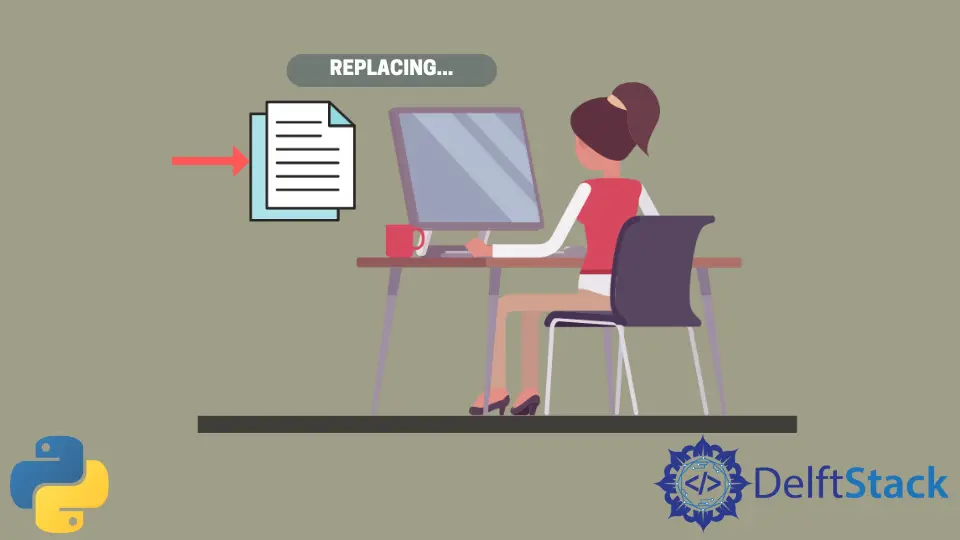
檔案處理被認為是任何 Web 應用程式的重要方面。與大多數程式語言類似,Python 完全能夠支援檔案處理。它允許你處理不同型別的檔案,本質上是執行一些基本操作,例如讀取和寫入。它還提供了一些其他檔案處理選項來操作檔案。
本教程演示了可用於在 Python 中替換檔案中的行的不同方法。
為了演示在本文中替換檔案中一行的不同方法,我們將使用一個文字檔案 motivation.txt
。
文字檔案(motivation.txt
):
There are always second chances in life. Anybody can bounce back from hardships. The hardships that we face are just a test to check our will. A strong will is all it takes to bounce back from a loss.
該檔案的儲存目錄路徑可能在讀者電腦上有所不同;因此,建議進行相應調整。
在 Python 中使用 for
迴圈和 replace()
函式替換檔案中的一行
open()
函式用於在 Python 中開啟檔案。該檔案可以以文字或二進位制格式開啟,這取決於程式設計師。open()
函式有多種模式,所有這些模式都提供了不同的可訪問性選項來開啟檔案。
簡單的 for
迴圈是遍歷給定文字檔案中的每一行並找到我們要替換的行的傳統方法。然後,可以使用 replace()
函式替換所需的行。所有這些都是在閱讀
模式下完成的。最後,以 write
模式開啟檔案,並將替換的內容寫入給定檔案中。
以下程式碼使用 for
迴圈和 replace()
函式。
# opening the file in read mode
file = open("motivation.txt", "r")
replacement = ""
# using the for loop
for line in file:
line = line.strip()
changes = line.replace("hardships", "situations")
replacement = replacement + changes + "\n"
file.close()
# opening the file in write mode
fout = open("motivation.txt", "w")
fout.write(replacement)
fout.close()
在 Python 中用更新的內容建立一個新檔案並替換原始檔案
有幾個函式正在工作來實現這個過程。要成功執行此方法,必須瞭解所有這些函式的過程。我們需要將三個模組中的幾個函式匯入到 Python 程式碼中。
- 首先,我們需要從
tempfile
模組匯入mkstemp()
函式。此函式用於返回一個元組作為輸出以及路徑和檔案描述符。 - 然後,我們需要從
shutil
模組匯入兩個函式。第一個函式是copymode()
函式,用於將許可權位從源路徑複製到目標路徑。第二個函式是move()
函式,它允許將檔案從一個地方移動到另一個地方。 - 最後,我們需要從
OS
模組匯入remove()
函式。此功能允許刪除路徑。
所有這些模組都需要匯入到當前的 Python 程式碼中才能執行程式碼而不會出現任何錯誤。實現這種方式的示例程式碼如下。
from tempfile import mkstemp
from shutil import move, copymode
from os import fdopen, remove
# storing the path where the file is saved on your device as a variable
path = "C:\\Users\Admin\Desktop\python\test\motivation.txt"
def replacement(filepath, hardships, situations):
# Creating a temp file
fd, abspath = mkstemp()
with fdopen(fd, "w") as file1:
with open(filepath, "r") as file0:
for line in file0:
file1.write(line.replace(hardships, situations))
copymode(filepath, abspath)
remove(filepath)
move(abspath, filepath)
replacement(path, "hardships", "situations")
在 Python 中使用 fileinput.input()
函式替換一行中的文字
fileinput.input()
方法逐行獲取檔案作為輸入,主要用於追加和更新給定檔案中的資料。
fileinput
和 sys
模組需要被匯入到當前的 Python 程式碼中,以便在沒有任何錯誤的情況下執行程式碼。以下程式碼使用 fileinput.input()
函式替換 Python 中一行中的文字。
import fileinput
import sys
def replacement(file, previousw, nextw):
for line in fileinput.input(file, inplace=1):
line = line.replace(previousw, nextw)
sys.stdout.write(line)
var1 = "hardships"
var2 = "situations"
file = "motivation.txt"
replacement(file, var1, var2)
在 Python 中使用 re
模組替換一行中的文字
re
模組是 Python 提供給處理正規表示式的程式設計師的內建模組,需要將其匯入到程式碼中。它有助於執行在給定的特定字串中搜尋模式的任務。
在這個方法中,我們使用了來自 RegEx
模組的兩個函式,即 re.compile()
和 re.escape()
。re.compile()
函式用於從正規表示式模式生成正規表示式物件,然後用於匹配。re.compile()
函式用於忽略或轉義 Python 中的特殊字元。
以下程式碼使用 Regex
模組中的幾個函式來替換 Python 中一行中的文字。
import re
def replacement(Path, text, subs, flags=0):
with open(filepath, "r+") as f1:
contents = f1.read()
pattern = re.compile(re.escape(text), flags)
contents = pattern.sub(subs, contents)
f1.seek(0)
f1.truncate()
f1.write(file_contents)
filepath = "motivation.txt"
text = "hardships"
subs = "situations"
replacement(filepath, text, subs)
在這裡,我們還使用了 sub()
函式來用字串或指定函式的結果替換給定的模式。
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn