How to Read File Into Dictionary in Python
-
Use the
split()
Function to Read a File Into a Dictionary in Python -
Use the
strip()
Function Along With thesplit()
Function to Read a File Into a Dictionary in Python - Use Dictionary Comprehension to Read a File Into a Dictionary in Python
-
Use
pandas
Library to Read a File Into a Dictionary in Python
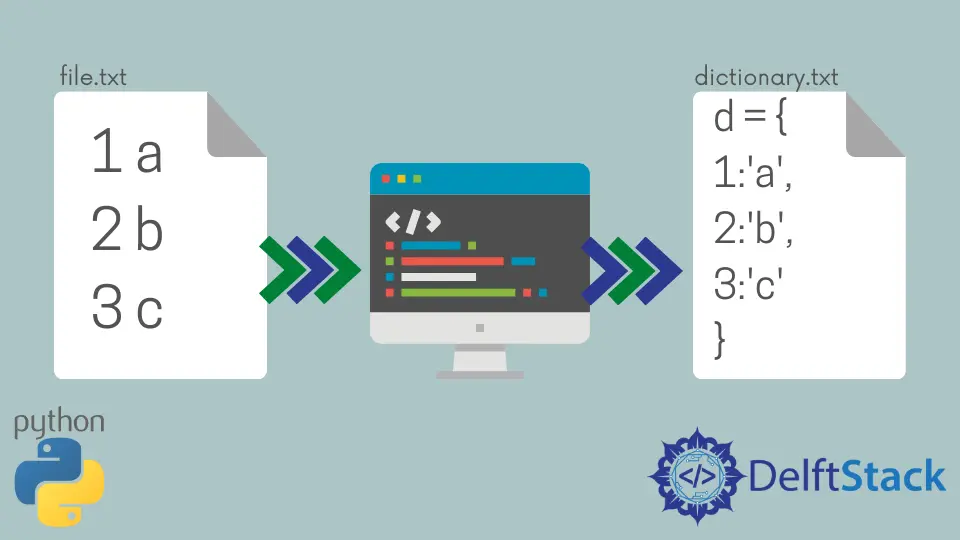
File handling is a vital part of the development and maintenance of any web application. Like other popular programming languages, Python is perfectly capable of supporting file handling. It allows the users to operate on different types of files while carrying some basic operations like reading and writing along with the other mainstream operations.
This tutorial demonstrates the different ways to read a file into a dictionary in Python.
For reference, we will be using a text file in the code to explain the different methods used in the article.
Contents of the file File1.txt
:
4 x
5 y
6 z
Use the split()
Function to Read a File Into a Dictionary in Python
The split()
function is generally utilized to chop a given string into a list.
The following code uses the split()
function to read a file into a dictionary in Python.
a = {}
with open("File1.txt") as f:
for line in f:
(k, v) = line.split()
a[int(k)] = v
print(a)
The above code provides the following output:
{4: 'x', 5: 'y', 6: 'z'}
Explanation:
- An empty dictionary
a
is created first. - The
open()
function is utilized to open and read from the given fileFile1.txt
- The contents of the file are read line by line.
- The line contents are then chopped using the
split()
function at space character. The character before the space is taken as the key while the character after the space is taken as the value of the dictionary. - The
for
loop is utilized for the iteration purpose and for reaching the end of the file.
Use the strip()
Function Along With the split()
Function to Read a File Into a Dictionary in Python
The strip()
function in Python removes any particularly specified characters or blank spaces at the beginning and the end of a string. The function returns a new string instead of making changes to the original one.
The following code uses the strip()
function and the split()
function to read a file into a dictionary in Python.
with open("File1.txt") as f:
a = dict(i.rstrip().split(None, 1) for i in f)
print(a)
The above code provides the following output:
{4: 'x', 5: 'y', 6: 'z'}
Explanation:
- An empty dictionary
a
is created first. - The
open()
function is utilized to open and read from the given fileFile1.txt
- The contents of the file are read line by line.
- The line contents are then chopped using the
split()
function at space character. Thestrip()
function is also utilized within the same to remove mentioned characters. - The
for
loop is utilized for the iteration purpose and for reaching the end of the file.
Use Dictionary Comprehension to Read a File Into a Dictionary in Python
The dictionary comprehension is a syntactical extension of the much popular and used list comprehension.
While dictionary comprehension is syntactically deployed similarly to list comprehension in the Python code, it has a big difference as the former produces the output as a dictionary, unlike the latter, which provides a list
as an output.
The following code uses the dictionary comprehension to read a file into a dictionary in Python.
with open("File1.txt") as f:
a = {int(k): v for line in f for (k, v) in [line.strip().split(None, 1)]}
print(a)
The above code provides the following output:
{4: 'x', 5: 'y', 6: 'z'}
Use pandas
Library to Read a File Into a Dictionary in Python
Pandas is a library provided by Python that is utilized for data analysis and manipulation. Pandas is an open-source, easy-to-use, and flexible library.
The following code uses the pandas
library to read a file into a dictionary in Python.
import pandas as pd
a = pd.read_csv("File1.txt", delimiter=" ", header=None).to_dict()[0]
print(a)
The above code provides the following output:
{4: 'x', 5: 'y', 6: 'z'}
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedInRelated Article - Python File
- How to Get All the Files of a Directory
- How to Append Text to a File in Python
- How to Check if a File Exists in Python
- How to Find Files With a Certain Extension Only in Python
- How to Read Specific Lines From a File in Python
- How to Check if File Is Empty in Python