How to Get Range of Floating Numbers in Python
-
Get Range of Floating-Point Numbers Using the
numpy.arange()
Method in Python - Get Range of Floating-Point Numbers Using the List Comprehension in Python
- Get Range of Floating-Point Numbers Using the Generator Comprehension in Python
- Get Range of Floating-Point Numbers Using the Generator Function in Python
-
Get Range of Floating-Point Numbers Using the
numpy.linspace()
Method in Python
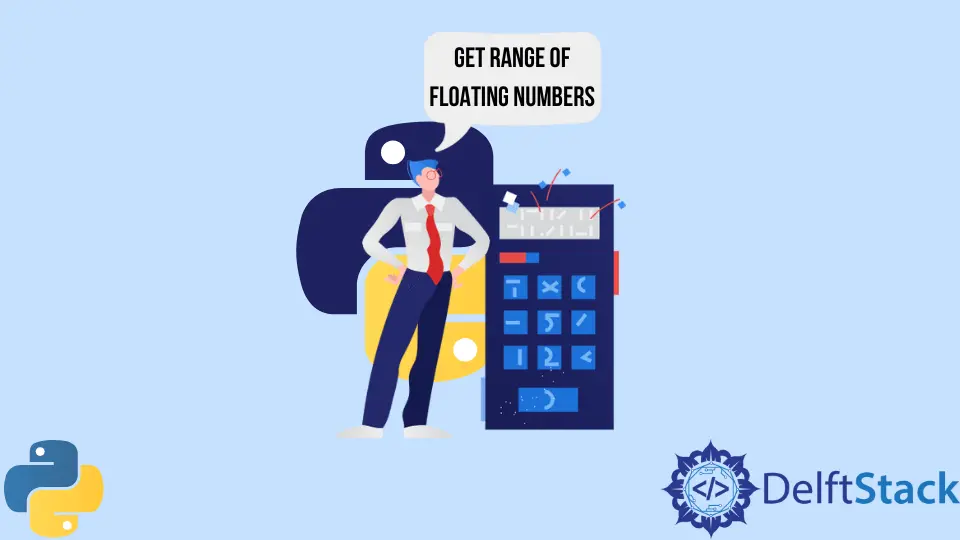
In this tutorial, we will look into various methods to get a sequence of float values in Python. Suppose we need a series of numbers with a step size of 0.1
, but the problem is that the range()
function does not accept a float as input and returns a TypeError
instead.
We can generate a sequence of float values with a uniform step size in Python using the methods explained below.
Get Range of Floating-Point Numbers Using the numpy.arange()
Method in Python
The numpy.arange(start, stop, step)
method returns a sequence of values within start
and stop
values with the step size equal to the step
value. The default value of the start
and step
arguments are 0 and 1, respectively.
The advantage of using the numpy.arange()
method is that it can generate the range of the float values. But it is not a default function of Python, and the NumPy
library is needed to use it.
The below example code demonstrates how to use the numpy.arange()
method to get the sequence of values from 0
to 1
with the step size equal to 0.1
.
import numpy as np
seq = np.arange(0, 1, 0.1)
print(seq)
Output:
[0. 0.1 0.2 0.3 0.4 0.5 0.6 0.7 0.8 0.9]
Get Range of Floating-Point Numbers Using the List Comprehension in Python
List comprehension is the syntactic method to create a new list from an existing list in Python. We can generate the range or sequence of float values by creating a new list from the sequence of values returned by the range()
method.
Since the range()
method can only generate the sequence of integers, we will have to multiply or divide each value of the sequence by the required float value to get the desired sequence. Since multiplying an integer, i.e., 3
with the float value 0.1
returns 0.30000000000000004
, that is why dividing the integer with 10
must be preferred to generate the sequence of float values.
The below example code demonstrates how to get the range or sequence of float values in Python using the list comprehension method.
seq = [x / 10 for x in range(0, 10)]
print(seq)
Output:
[0.0, 0.1, 0.2, 0.3, 0.4, 0.5, 0.6, 0.7, 0.8, 0.9]
Get Range of Floating-Point Numbers Using the Generator Comprehension in Python
Generator comprehension is a syntactic approach similar to list comprehension that creates an iterable generator object in Python. We can access the new value from the generator by using the next()
method or iterate through the whole sequence using the for
loop.
The below example code demonstrates how to use the generator comprehension to get the range of floats in Python.
seq = (x / 10 for x in range(0, 10))
for x in seq:
print(x, end=" ")
Output:
0.0 0.1 0.2 0.3 0.4 0.5 0.6 0.7 0.8 0.9
Get Range of Floating-Point Numbers Using the Generator Function in Python
Although the generator comprehension is a concise and more straightforward way to get a generator object in Python, the generator function is a reusable and better way to get a range of float values using the start
, stop
, and step
values of our choice.
The below example code demonstrates how to create a generator function using the yield
keyword, similar to the range()
function in Python.
def myrange(start, stop, step):
while start < stop:
yield start
start = start + step
seq = myrange(0.0, 1.0, 0.1)
for x in seq:
print(x)
Get Range of Floating-Point Numbers Using the numpy.linspace()
Method in Python
The numpy.linspace()
method like the numpy.arange()
method returns the sequence of values from the start
value to the stop
value. Instead of step
argument, the numpy.linspace()
method takes num
argument, which specifies the number of values to generate from start
to stop
value.
Therefore we will have to provide the number of values num
to the numpy.linspace()
method; the following formula can calculate the value of num
using start
, stop
, and step
values.
num = (stop - start)/step
The below example code demonstrates how to use the numpy.linspace()
to generate the range of floats from 0
to 1
with the step
equal to 0.1
. The num
value for the required sequence will be (0 - 1)/0.1 = 10
.
seq = np.linspace(0, 1, 10, endpoint=0)
print(seq)
Output:
array([0. , 0.1, 0.2, 0.3, 0.4, 0.5, 0.6, 0.7, 0.8, 0.9]
The endpoint
argument specifies whether to include the last value of the sequence or not, as the sequence starts from 0
by default.