How to Print to stderr in Python
-
the Differences between
stdout
andstderr
in Python -
Print to
stderr
in Python 3.x -
Redirecting
print()
tostderr
-
Use the
sys.stderr
to Print tostderr
-
Logging Warnings to
stderr
Usinglogging.warning()
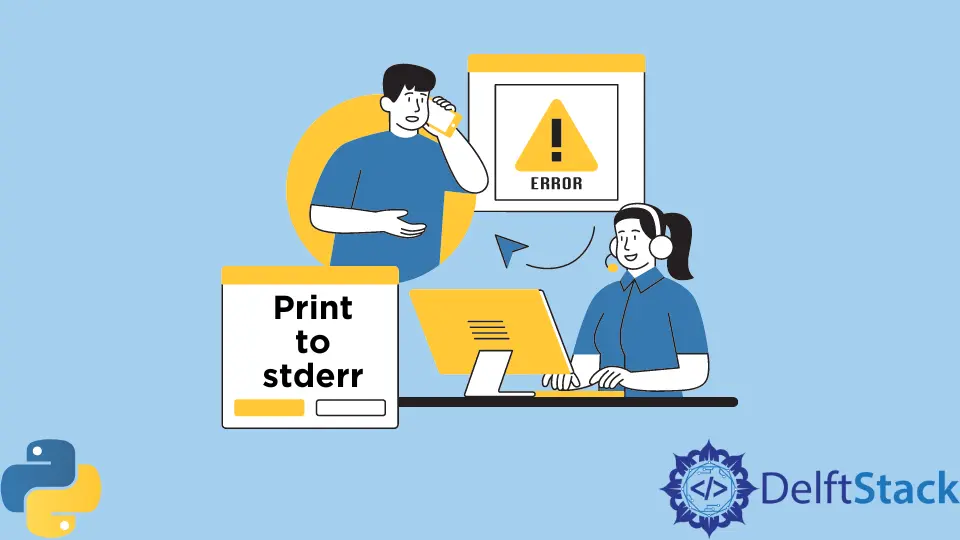
Efficient management of standard input and output streams is essential for debugging, error handling, and logging. Among these, the standard error stream, often referred to as stderr
, plays a pivotal role in reporting errors and exceptional events during program execution. Understanding how to harness stderr effectively can be a valuable skill for any Python developer.
This article delves into the world of stderr in Python, shedding light on its significance alongside its more familiar counterpart, stdout
(standard output). We will explore various methods to print to stderr, including the sys.stderr.write()
function, the print()
function with a file parameter, and the robust capabilities offered by the Python logging
module.
the Differences between stdout
and stderr
in Python
In Python, there are two primary streams for handling output: stdout
(standard output) and stderr
(standard error). While both streams serve the purpose of conveying information, they have distinct roles and should be used for specific types of output. Understanding the differences between these two streams is essential for effective debugging and error handling.
stdout
(Standard Output)
stdout
is the default stream for regular program output. It is used to display information that is not considered an error or an exceptional event. When you use print()
statements without specifying a target stream, Python sends the output to stdout
by default. Here’s an example:
pythonCopy code# Printing to stdout
print("This is regular program output.")
In this example, the text “This is regular program output.” is sent to stdout
. It’s the place where you should direct information, messages, and data that represent the normal flow of your program.
stderr
(Standard Error)
On the other hand, stderr
is reserved for error messages and exceptional conditions. Any issues, errors, or warnings that occur during program execution should be reported to stderr
. You can access stderr
using the sys.stderr
stream, as we will discuss in the next chapter. Here’s an example:
pythonCopy codeimport sys
# Printing an error message to stderr
sys.stderr.write("Error: File not found.\n")
In this example, the error message Error: File not found.
is written to stderr
. This ensures that error messages are separated from regular program output, making it easier to identify and address issues.
Why Separate stdout
and stderr
?
- Clarity: Separating
stdout
andstderr
provides clarity in your program’s output. It distinguishes between regular program output and error messages, making it easier to understand what’s happening. - Error Handling: Redirecting error messages to
stderr
allows for more robust error handling. You can redirectstderr
to a log file or a separate error stream for further analysis, ensuring that error information is not lost. - Debugging: When debugging, you can focus on
stderr
to quickly identify and address issues without being distracted by regular program output.
Print to stderr
in Python 3.x
In Python, the print()
function is a versatile tool for displaying information on the console. By default, print()
directs its output to the standard output stream (sys.stdout
). However, you can also leverage this function to print messages and errors to the standard error stream (stderr
) by specifying the file
parameter. In this chapter, we’ll explore how to use the print()
function to send output to stderr
and discuss scenarios where this approach is beneficial.
For Python3.x, the print
function has a keyword argument file
that specifies the printing destination. It is sys.stdout
by default but could be sys.stderr
or even a file path.
In Python, the print()
function is a versatile tool for displaying information on the console. By default, print()
directs its output to the standard output stream (stdout
). However, you can also leverage this function to print messages and errors to the standard error stream (stderr
) by specifying the file
parameter. In this chapter, we’ll explore how to use the print()
function to send output to stderr
and discuss scenarios where this approach is beneficial.
Redirecting print()
to stderr
To redirect the print()
function to stderr
, you simply need to provide sys.stderr
as the value for the file
parameter. Here’s how it’s done:
pythonCopy codeimport sys
# Redirecting print() to stderr
print("This is a regular message.", file=sys.stderr)
In this example, we import the sys
module to access sys.stderr
and then use it as the file
parameter for print()
. This causes the message “This is a regular message.” to be printed to stderr
instead of stdout
.
Benefits of Using print()
with file=sys.stderr
- Clear Separation
By printing tostderr
, you make a clear distinction between regular program output and error messages. This separation enhances the readability of your program’s output, making it easier to identify and address issues.
-
Consistency with Existing Code
If your codebase relies onprint()
for output, usingprint()
withfile=sys.stderr
allows you to integrate error messages seamlessly. You can maintain a consistent code style while effectively handling errors. -
Easy Debugging
When debugging your code, focusing onstderr
can help you quickly spot and resolve errors without being distracted by regular program output.
Custom Error Messages
You can use the print()
function with file=sys.stderr
to create custom error messages, including relevant information about the error or exceptional condition. Here’s an example:
pythonCopy codeimport sys
def divide(a, b):
try:
result = a / b
except ZeroDivisionError as e:
print(f"Error: Division by zero. Details: {e}", file=sys.stderr)
else:
print(f"Result of {a} divided by {b} is {result}")
if __name__ == "__main__":
divide(10, 0)
In this example, the custom error message “Error: Division by zero. Details: division by zero” is printed to stderr
when a division by zero occurs. Meanwhile, the result of the division is printed to stdout
.
Use the sys.stderr
to Print to stderr
The sys
module of Python provides valuable information and key functionalities. sys.stderr.write()
method can be used. sys.stderr.write()
method prints the message as the given parameter to the stderr
. See the example below.
import sys
sys.stderr.write("Error!")
Output:
Error!
In this example, we first import the sys
module. Then, we use sys.stderr.write()
to write an error message to stderr
.
Error Message Formatting
While sys.stderr.write()
is a powerful tool for printing to stderr
, it doesn’t automatically format error messages. You are responsible for crafting informative and clear error messages. It’s a good practice to include relevant information such as error codes, descriptions, and context to help identify and resolve issues efficiently.
pythonCopy codeimport sys
# Custom error message with context
error_message = "Error 404: Resource not found in the 'data/' directory."
# Printing to stderr
sys.stderr.write(error_message + "\n")
In this example, we create a custom error message that includes an error code (404
) and a description. Including such details can greatly assist in debugging and resolving issues.
Logging Warnings to stderr
Using logging.warning()
In Python, the logging
module provides a comprehensive framework for handling various types of log messages, including warnings. While the primary purpose of the logging
module is to manage logs, it can also be used to print warnings and error messages to the standard error stream (stderr
). In this chapter, we’ll explore how to use the logging.warning()
method to log warnings to stderr
and discuss why this approach is beneficial.
Logging Warnings with logging.warning()
The logging.warning()
method is a part of the logging
module and is specifically designed for reporting warnings and exceptional conditions in your Python code. By default, it sends warning messages to the standard error stream (stderr
). Here’s how to use it:
pythonCopy codeimport logging
# Configure the logging module (optional)
logging.basicConfig(level=logging.WARNING)
# Log a warning
logging.warning("This is a warning message.")
In this example, we import the logging
module and configure it with a logging level of WARNING
. Then, we use logging.warning()
to log a warning message, which will be sent to stderr
by default.
Benefits of Using logging.warning()
-
Structured Logging
Thelogging
module provides structured and consistent logging capabilities, making it easier to manage logs and understand the context of warnings. Warnings logged withlogging.warning()
include timestamps, log levels, and the log message itself. -
Fine-Grained Control
With thelogging
module, you can control the verbosity and destination of log messages. You can configure it to send warnings and errors tostderr
while directing other log messages to different locations, such as log files.
- Compatibility with Existing Logging
If your application already uses thelogging
module for logging purposes, usinglogging.warning()
ensures consistency with the existing logging infrastructure. You can integrate warning messages seamlessly into your logging workflow.
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn