How to Print String and Variable in Python
- Use Commas in Python 2.7 to Print String and Variable
-
Use
%
Operator to Print a String and Variable in Python 2.7 - Use Concatenation to Print a String and Variable in Python
-
Use
f-strings
Method to Print a String and Variable in Python 3.6 and Above
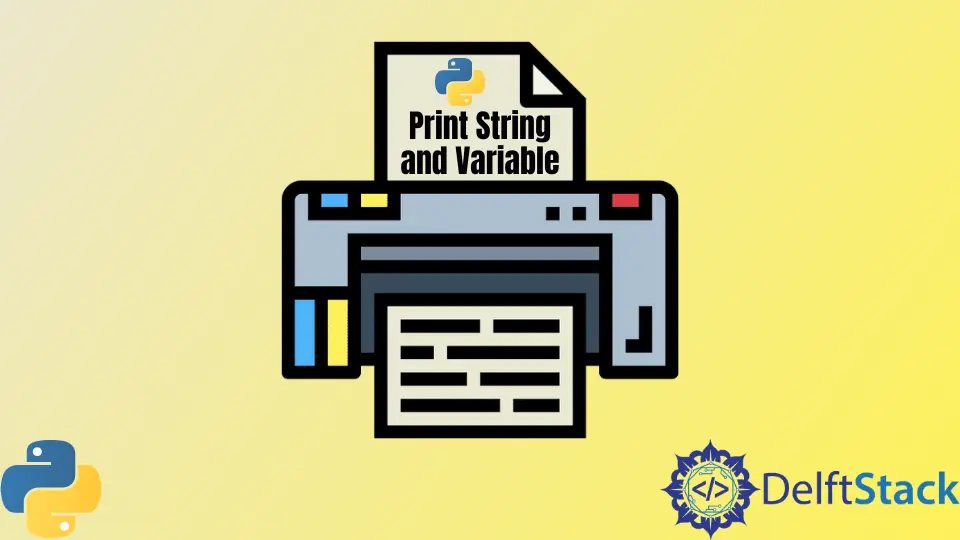
This tutorial will enlist different methods to print strings and variables in one line in Python. The ways of printing a string and variable vary as per the Python versions. For example, a string and variable can be printed by using concatenation, using f-strings
, and so on. So the tutorial will provide a deep insight into the different methods by providing relevant example codes to explain the concept.
Use Commas in Python 2.7 to Print String and Variable
The print
statement in Python 2.7 provides the functionality to print string and variable. The print statement takes the message to be printed in quotations. A comma is used to print the variable along with the message. The print statement evaluates each expression which is separated with a comma. If an expression is not a string, it will be converted into a string and then displayed. And the print
statement is always followed by a newline unless it ends with a comma.
An example code to illustrate the concept of how to print string and variable in Python is given below.
amount = 100
print " The amount i have is:", amount
Output:
The amount i have is: 100
Use %
Operator to Print a String and Variable in Python 2.7
Another method of printing a string and variable in Python 2.7 is by using string formatting operators. In this method, the print
statement uses the %
operator in the message. It defines the message along with a special %
character.
The syntax of the %
operator is shown below.
" %[s/d] " % (value1, vlaue2, ...)
The %
operator defines the data type of the variable. Different letters are used to define different data types. For example, if the variable is a decimal, we will use the %d
operator. If it is a string, we will use the %s
operator, and so on.
Below is an example code to explain the concept of using a string formatting operator to print a string and variable in Python.
grade = "A"
marks = 90
print("John doe obtained %s grade with %d marks." % (grade, marks))
Output:
John doe obtained A grade with 90 marks.
Use Concatenation to Print a String and Variable in Python
The concatenation operator is denoted with the +
sign. It takes two expressions and concatenates them. The expressions must be strings because the concatenation operator works only with strings. The concatenation operator evaluates each expression, and if an expression is not a string, it gives an error. So we need to explicitly cast an expression that is not of the string data type. We can type cast it by using the str(var)
method. The var
is the variable that is not a string.
An example code is given below to elaborate on how to print a string and variable in Python.
grade = "A"
marks = 90
print("John doe obtained " + grade + " grade with " + str(marks) + " marks.")
Output:
John doe obtained A grade with 90 marks.
Use f-strings
Method to Print a String and Variable in Python 3.6 and Above
If you are using Python 3.6 and above, f-strings
method can be used. The f
letter indicates that the string is used for the purpose of formatting. It is the same as the simple print
method in Python. However, in this method, we will use curly braces to indicate our variables. The variable we want to print will be added to the curly braces.
The expression given in the print
method is evaluated at run time, and the formatting is done using the __format__
protocol. An example code is given below to explain how to use f-strings
to print a string and variable in Python.
grade = "A"
marks = 90
print(f"John doe obtained {grade} grade with {marks} marks.")
Output:
John doe obtained A grade with 90 marks.
Moreover, you can also put arithmetic expressions in the f-strings
, and they can evaluate them because f-strings
are evaluated at run time. An example code is given below.
grade = "A"
marks = 45
print(f"John doe obtained {grade} grade with {marks*2} marks.")
Output:
John doe obtained A grade with 90 marks.
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn