在 Python 中列印字串和變數
- 在 Python 2.7 中使用逗號來列印字串和變數
-
使用
%
運算子在 Python 2.7 中列印字串和變數 - 在 Python 中使用串聯列印字串和變數
-
使用
f-strings
方法在 Python 3.6 及更高版本中列印字串和變數
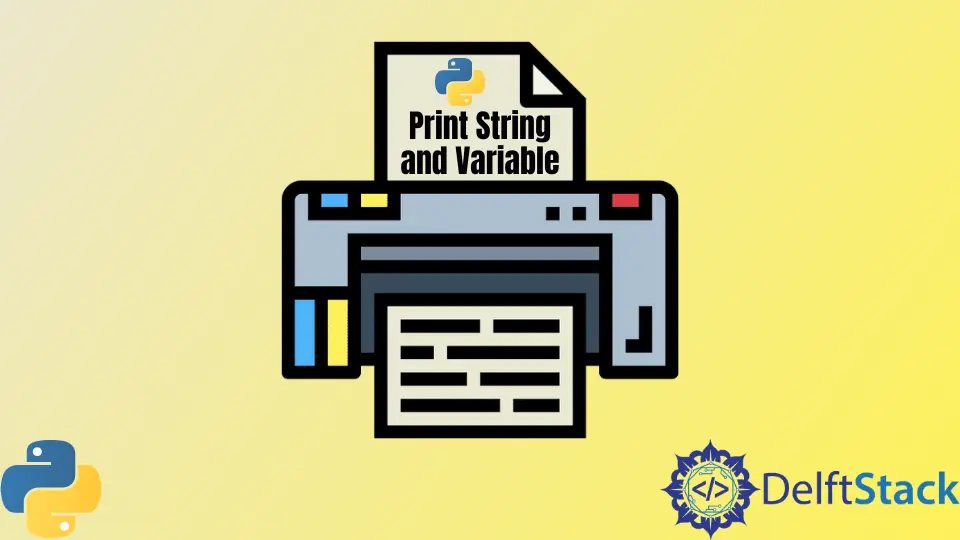
本教程將使用不同的方法在 Python 的一行中列印字串和變數。根據 Python 版本的不同,列印字串和變數的方式也有所不同。例如,可以使用串聯,f-strings
等來列印字串和變數。因此,本教程將通過提供相關的示例程式碼來解釋該概念,從而提供對不同方法的深入瞭解。
在 Python 2.7 中使用逗號來列印字串和變數
Python 2.7 中的 print
語句提供了列印 string
和變數的功能。列印語句將要列印的訊息用引號引起來。逗號用於將變數和訊息一起列印。print 語句評估每個用逗號分隔的表示式。如果表示式不是字串,則將其轉換為字串然後顯示。並且 print
語句之後總是帶有換行符,除非它以逗號結尾。
下面給出了一個示例程式碼,說明了如何在 Python 中列印字串和變數的概念。
amount = 100
print " The amount i have is:", amount
輸出:
The amount i have is: 100
使用%
運算子在 Python 2.7 中列印字串和變數
在 Python 2.7 中列印字串和變數的另一種方法是使用字串格式運算子。在這種方法中,print
語句在訊息中使用%
運算子。它定義了訊息以及特殊的%
字元。
%
運算子的語法如下所示。
" %[s/d] " % (value1, vlaue2, ...)
%
運算子定義變數的資料型別。不同的字母用於定義不同的資料型別。例如,如果變數是小數,我們將使用%d
運算子。如果是字串,我們將使用%s
運算子,依此類推。
以下是一個示例程式碼,解釋了在 Python 中使用字串格式運算子列印字串和變數的概念。
grade = "A"
marks = 90
print("John doe obtained %s grade with %d marks." % (grade, marks))
輸出:
John doe obtained A grade with 90 marks.
在 Python 中使用串聯列印字串和變數
串聯運算子用+
符號表示。它接受兩個表示式並將它們連線起來。表示式必須是字串,因為串聯運算子僅適用於字串。串聯運算子計算每個表示式,如果表示式不是字串,則會產生錯誤。因此,我們需要顯式轉換不是字串資料型別的表示式。我們可以使用 str(var)
方法鍵入強制型別轉換。var
是不是字串的變數。
下面給出了一個示例程式碼,以詳細說明如何在 Python 中列印字串和變數。
grade = "A"
marks = 90
print("John doe obtained " + grade + " grade with " + str(marks) + " marks.")
輸出:
John doe obtained A grade with 90 marks.
使用 f-strings
方法在 Python 3.6 及更高版本中列印字串和變數
如果你使用的是 Python 3.6 及更高版本,則可以使用 f-strings
方法。字母 f
表示該字串用於格式化。它與 Python 中的簡單 print
方法相同。但是,在這種方法中,我們將使用大括號指示變數。我們要列印的變數將被新增到大括號中。
在執行時評估 print
方法中給出的表示式,並使用 __format__
協議進行格式化。以下示例程式碼說明了如何在 Python 中使用 f-strings
來列印字串和變數。
grade = "A"
marks = 90
print(f"John doe obtained {grade} grade with {marks} marks.")
輸出:
John doe obtained A grade with 90 marks.
此外,你還可以將算術表示式放在 f-strings
中,並且可以對它們進行求值,因為在執行時會評估 f-strings
。下面給出了示例程式碼。
grade = "A"
marks = 45
print(f"John doe obtained {grade} grade with {marks*2} marks.")
輸出:
John doe obtained A grade with 90 marks.
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn