How to Print Colored Text in Python
- Use ANSI Escape Codes to Print Colored Text in Python
-
Use the
colorama
Module to Print Colored Text in Python
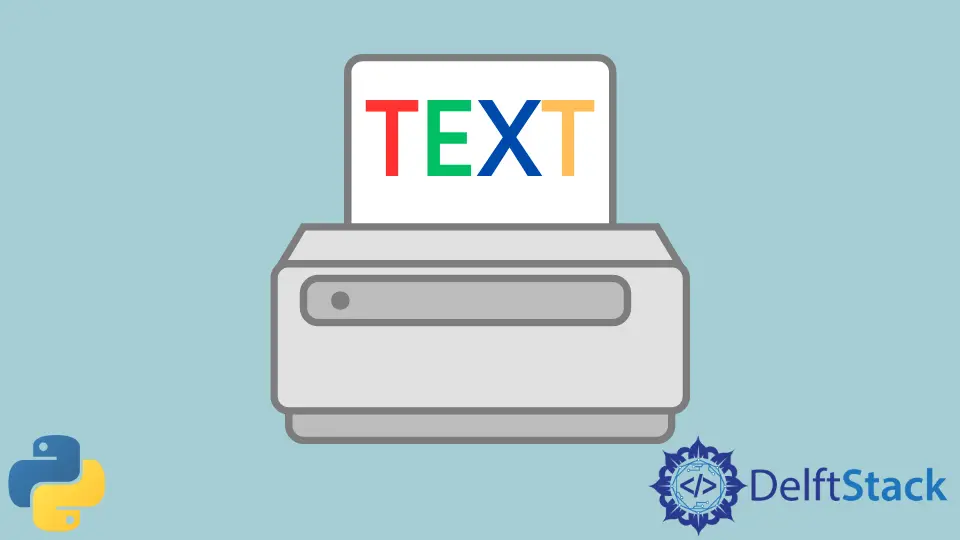
This tutorial shows you how to generate colored text when you print in Python.
The only way to manipulate the command line console using input is by using ANSI Escape Codes. These codes can manipulate console functions, such as text or background color, cursor settings, fonts, and other modifiable elements within the console.
Use ANSI Escape Codes to Print Colored Text in Python
First, let’s declare a Python class that gives us a few ANSI Code that represents colors that we can work with.
class bcolors:
OK = "\033[92m" # GREEN
WARNING = "\033[93m" # YELLOW
FAIL = "\033[91m" # RED
RESET = "\033[0m" # RESET COLOR
3 of these variables are actual ANSI Code for colors, while the variable RESET
is there to set the color back to the default.
The function print()
outputs string argument onto the command line console.
If you want the output of print()
to be colored, you would have to insert ANSI code within the string that can manipulate the command line console.
Using class bcolors
, we’ll print 3 different lines with different colors.
print(bcolors.OK + "File Saved Successfully!" + bcolors.RESET)
print(bcolors.WARNING + "Warning: Are you sure you want to continue?" + bcolors.RESET)
print(bcolors.FAIL + "Unable to delete record." + bcolors.RESET)
We prefix the string with the color you want it to reflect and suffix it with bcolors.RESET
to reset the color to default before the next time you use print()
or the next time you use the terminal.
If you’re using Python 3, you can also format your print()
statement like this:
print(f"{bcolors.OK}File Saved Successfully!{bcolors.RESET}")
print(f"{bcolors.WARNING}Warning: Are you sure you want to continue?{bcolors.RESET}")
print(f"{bcolors.FAIL}Unable to delete record.{bcolors.RESET}")
Output:
After outputting the last line, the terminal will be reset back to its default color because of bcolors.RESET
. If you fail to put it at the end of your lines, the text within the terminal will be colored the last color you set within print()
. In this case, it would be red.
Use the colorama
Module to Print Colored Text in Python
ANSI’s problem is it might not work well with Windows OS, so you would need workarounds to make it work within Windows consoles.
colorama
is a Python module that uses ANSI escape codes. This module also makes it possible for ANSI to be compatible with Windows. The documentation explains how they made it possible to wrap ANSI code for Windows compatibility.
Here’s a list of available colorama
foreground colors:
BLACK, RED, GREEN, YELLOW, BLUE, MAGENTA, CYAN, WHITE, RESET
They also have styles, including a RESET_ALL
that resets all the current ANSI modifications, while Fore.RESET
only resets the latest ANSI.
DIM, NORMAL, BRIGHT, RESET_ALL
First, we need to install the colorama
as it is a community-created library. We can use either pip
or anaconda
to install this dependency.
- For
pip
orpip3
pip install colorama
pip3 install colorama //if you're using python3
- For
anaconda
conda install -c anaconda colorama
We’ll try some of the colors out and output several lines of different colors and styles. We use colorama.init()
to make these settings work for Windows.
import colorama
from colorama import Fore
from colorama import Style
colorama.init()
print(Fore.BLUE + Style.BRIGHT + "This is the color of the sky" + Style.RESET_ALL)
print(Fore.GREEN + "This is the color of grass" + Style.RESET_ALL)
print(Fore.BLUE + Style.DIM + "This is a dimmer version of the sky" + Style.RESET_ALL)
print(Fore.YELLOW + "This is the color of the sun" + Style.RESET_ALL)
Output:
DIM
and BRIGHT
will output different shades of the color when used on the same color. RESET_ALL
resets the color to default to be set to another color or leave it back to the default.
In summary, the only way to print out colored text in Python is by making use of ANSI Escape Codes. It’s the only way for the console to understand instructions from Python’s print()
statement. You can do it manually by including the actual escape code within your print()
, or use colorama
to wrap the ANSI code into a more readable format.
If you want to get more options for ANSI code colors, there are many resources on the internet to provide them, this article is a good start. You can also format text (bold, underline, italicize), change your console background, and much more with ANSI code.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn