Colors in Python
- Understanding Color Representation
- Using Tkinter for Color Representation
- Visualizing Data with Matplotlib
- Creating Games with Pygame
- Conclusion
- FAQ
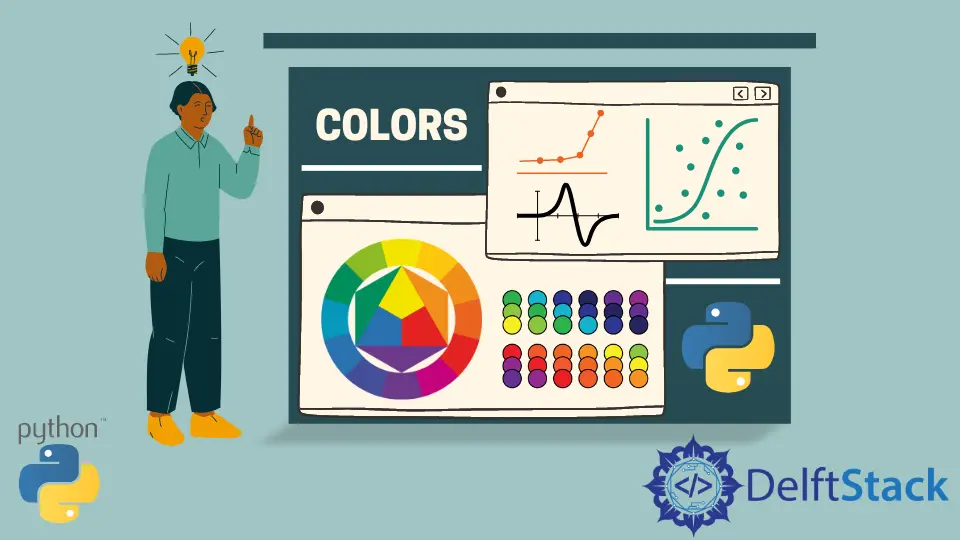
Colors play a significant role in programming, especially when it comes to visual representation in applications. In Python, you can manipulate colors in various ways, whether you’re working with graphics, data visualization, or even simple console output.
This tutorial aims to provide a detailed overview of how colors can be utilized in Python, covering essential libraries and methods. Whether you’re a beginner or an experienced developer, understanding how to work with colors in Python can enhance your projects and make them visually appealing. Let’s dive into the colorful world of Python!
Understanding Color Representation
Before we jump into the practical aspects of working with colors in Python, it’s essential to understand how colors are represented. In programming, colors are often defined using RGB (Red, Green, Blue) values, where each color component can range from 0 to 255. This representation allows for a wide spectrum of colors. For instance, pure red is represented as (255, 0, 0), while pure green is (0, 255, 0).
Python has several libraries that facilitate color manipulation, including Tkinter, Matplotlib, and Pygame. Each library offers unique functionalities for incorporating colors into your projects. Let’s explore how to utilize these libraries effectively.
Using Tkinter for Color Representation
Tkinter is Python’s standard GUI (Graphical User Interface) toolkit. It provides a straightforward way to work with colors in GUI applications. You can create windows, buttons, and other widgets while customizing their colors easily.
Here’s a simple example of how to set the background color of a Tkinter window:
import tkinter as tk
root = tk.Tk()
root.title("Color Example")
root.configure(bg='lightblue')
label = tk.Label(root, text="Hello, World!", bg='lightblue', fg='darkblue')
label.pack(pady=20)
root.mainloop()
Output:
This will create a window with a light blue background and a label that says "Hello, World!" in dark blue.
In this code, we first import the Tkinter library and create a basic window. The configure
method sets the background color of the window to light blue. We then create a label with the same background color and a contrasting foreground color (text color) of dark blue. This combination enhances readability and aesthetics. Tkinter allows you to use color names or RGB values, providing flexibility in color choices.
Visualizing Data with Matplotlib
Matplotlib is a powerful library for creating static, animated, and interactive visualizations in Python. It’s widely used for plotting data and can easily incorporate colors to enhance visual appeal.
Here’s an example of how to create a simple bar chart with different colors using Matplotlib:
import matplotlib.pyplot as plt
categories = ['A', 'B', 'C', 'D']
values = [10, 15, 7, 12]
colors = ['red', 'green', 'blue', 'orange']
plt.bar(categories, values, color=colors)
plt.title("Bar Chart Example")
plt.xlabel("Categories")
plt.ylabel("Values")
plt.show()
In this example, we define categories and their corresponding values. The colors
list specifies the color for each bar in the chart. By passing the color
parameter to the bar
function, we can customize the appearance of our chart. Matplotlib supports a wide range of color options, including named colors, hex codes, and RGB tuples, making it versatile for various visualization needs.
Creating Games with Pygame
Pygame is a popular library for game development in Python. It provides functionalities to handle graphics, sound, and user input, making it ideal for creating interactive applications. Working with colors in Pygame is straightforward and essential for rendering graphics.
Here’s a basic example of how to create a Pygame window with a colored background:
import pygame
pygame.init()
screen = pygame.display.set_mode((400, 300))
pygame.display.set_caption("Pygame Color Example")
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill((0, 128, 255)) # Fill the screen with a blue color
pygame.display.flip()
pygame.quit()
Output:
This will create a blue window that remains open until you close it.
In this code, we initialize Pygame and create a window. The fill
method is used to set the background color of the window. In this case, we fill it with a blue color represented by the RGB value (0, 128, 255). The pygame.display.flip()
method updates the screen, allowing the color change to take effect. Pygame supports RGB values, making it easy to create vibrant graphics for your games.
Conclusion
Colors in Python can greatly enhance the visual aspect of your applications, whether you’re creating a GUI with Tkinter, visualizing data with Matplotlib, or developing games with Pygame. By understanding how to manipulate colors using these libraries, you can create more engaging and visually appealing projects. Experiment with different color combinations and representations to find what works best for your needs. Embrace the colorful possibilities that Python offers!
FAQ
-
What are the common color representations in Python?
RGB (Red, Green, Blue) values are commonly used, along with color names and hex codes. -
Can I use hex color codes in Tkinter?
Yes, Tkinter supports hex color codes, allowing you to specify colors in the format #RRGGBB. -
How do I customize colors in Matplotlib?
You can customize colors in Matplotlib by passing a list of colors to thecolor
parameter in plotting functions. -
Is Pygame suitable for beginners?
Yes, Pygame is beginner-friendly and provides a straightforward way to learn game development in Python. -
Can I create a color gradient in Matplotlib?
Yes, Matplotlib supports color gradients through colormaps, which can be applied to various types of plots.