How to Log an Error With Debug Information in Python
-
Use the
exception()
Method to Log an Error With Detailed Debug Information in Python -
Use the
exception()
Method Withstack_info
to Log an Error With Detailed Debug Information in Python 3.2+ -
Use the
exception()
Method Withexc_info
to Log an Error With Detailed Debug Information in Python 3.5+
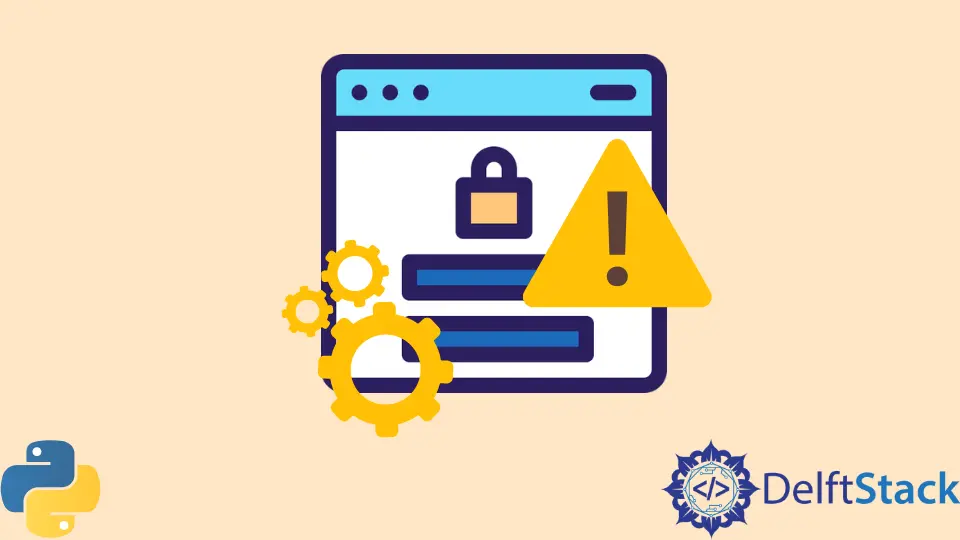
This article explains how to log an error with detailed debug information in Python.
To display detailed debug information, import the logging
library in Python and utilize the logging.exception()
method. It will display the error message and the stack trace.
We know that the exception string will help you understand the exact error that occurred in Python. Apart from this, we can determine detailed information about the exception and the line of code that generated the exception.
Call the logging.exception()
method within the except
code block; this helps display a stack trace with the error message. On this logger, it logs the message with level ERROR
. The exception information is appended to the logging message.
Use the exception()
Method to Log an Error With Detailed Debug Information in Python
Here is an example that demonstrates how you can log an error with detailed debug information in Python.
import logging
def fnc_divide(n):
try:
result = n / 0
print("The result=", result)
except:
print("The except block")
logging.exception("The detailed error message -")
fnc_divide(5)
Output:
The except block
ERROR:root:The detailed error message -
Traceback (most recent call last):
File "C:/Users/../a.py", line 4, in fnc_divide
result=n/0
ZeroDivisionError: division by zero
Notice that in the output, the detailed information is displayed regarding the error as listed below.
- It mentions the module/function where the error occurred. In this example, the output displays that the error occurred within the
fnc_divide()
method. - It mentions the line number where the error occurred. In this example, the output displays that the error occurred on line number 4.
- It mentions the exact error. In this example, it is
ZeroDivisionError: division by zero
.
Use the exception()
Method With stack_info
to Log an Error With Detailed Debug Information in Python 3.2+
From Python 3.2+, you can pass the stack_info
argument as True
. It shows how you got to a particular point in the code; this is also the case when no exceptions are raised.
If stack_info
is True
, the stack information is added to the logging message, plus the actual logging call, and is associated with the stack frames in order. The order is from the bottom of the stack up to the logging call in the current thread.
Look at this example code below.
import logging
def fnc_dividestack(n):
try:
result = n / 0
except Exception:
logging.exception("The detailed error message -", stack_info=True)
fnc_dividestack(4)
Output:
ERROR:root:The detailed error message -
Traceback (most recent call last):
File "C:/Users/Ri..error.py", line 5, in fnc_dividestack
result=n/0
ZeroDivisionError: division by zero
Stack (most recent call last):
File "C:/Users/Ri..error.py", line 9, in <module>
fnc_dividestack(4)
File "C:/Users/Ri..error.py", line 7, in fnc_dividestack
logging.exception("The detailed error message -", stack_info=True)
Use the exception()
Method With exc_info
to Log an Error With Detailed Debug Information in Python 3.5+
From Python 3.5+, you can pass an exception instance in the exc_info
argument. This parameter accepts exception instances.
Note that the exc_info
tuple holds the current exception information if an exception occurs; else, it holds None
. Below is an example that demonstrates this process.
import logging
def fnc_divide(n):
try:
result = n / 0
print("The result=", result)
except Exception as e:
logging.exception("The exc_info - Zero Division error", exc_info=e)
fnc_divide(5)
Output:
ERROR:root:The exc_info - Zero Division error
Traceback (most recent call last):
File "C:/Users/R..ror.py", line 4, in fnc_divide
result=n/0
ZeroDivisionError: division by zero