How to Check if List Contains a String in Python
-
Use the
for
Loop to Check a Specific String in a Python List - Use the List Comprehension to Check a Specific String in a Python List
-
Use the
filter()
Function to Get a Specific String in a Python List
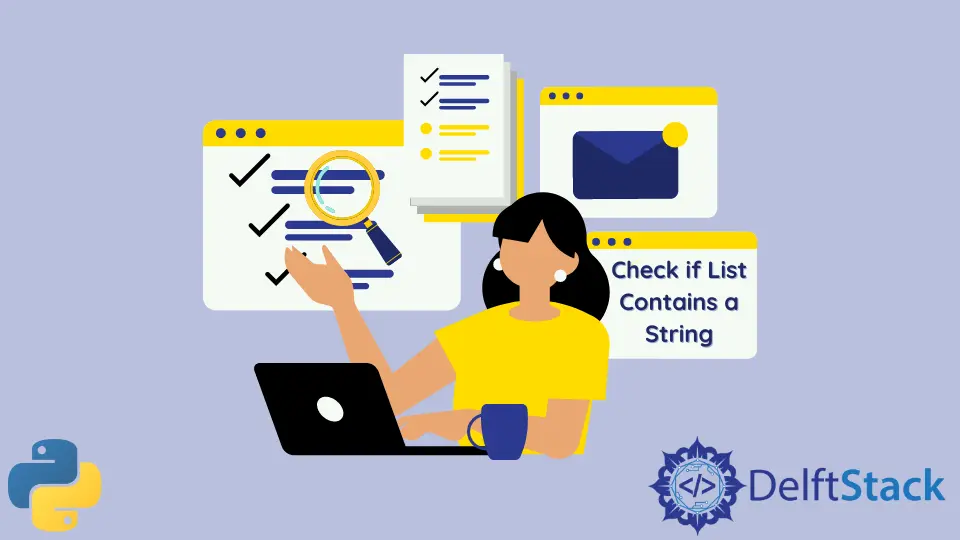
Strings are a sequence of characters. Just like strings store characters at specific positions, we can use lists to store a collection of strings.
In this tutorial, we will get a string with specific values in a Python list.
Use the for
Loop to Check a Specific String in a Python List
The for
is used to iterate over a sequence in Python.
Its implementation for obtaining a string containing specific values is shown in the example below.
py_list = ["a-1", "b-2", "c-3", "a-4"]
new_list = []
for x in py_list:
if "a" in x:
new_list.append(x)
print(new_list)
Output:
['a-1','a-4']
In the above code, the if
statement is used inside the for
loop to search for strings containing a
in the list py_list
. Another list named new_list
is created to store those specific strings.
Use the List Comprehension to Check a Specific String in a Python List
List comprehension is a way to create new lists based on the existing list. It offers a shorter syntax being more compact and faster than the other functions and loops used for creating a list.
For example,
py_list = ["a-1", "b-2", "c-3", "a-4"]
r = [s for s in py_list if "a" in s]
print(r)
Output:
['a-1', 'a-4']
In the above code, list comprehension is used to search for strings having a
in the list py_list
.
Note that writing the same code using other functions or loops would have taken more time, as more code is required for their implementation, but list comprehension solves that problem.
We can also use list comprehension to find out strings containing multiple specific values i.e., we can find strings containing a
and b
in py_list
by combining the two comprehensions.
For example,
py_list = ["a-1", "b-2", "c-3", "a-4", "b-8"]
q = ["a", "b"]
r = [s for s in py_list if any(xs in s for xs in q)]
print(r)
Output:
['a-1', 'b-2', 'a-4','b-8']
Use the filter()
Function to Get a Specific String in a Python List
The filter()
function filters the given iterable with the help of a function that checks whether each element satisfies some condition or not.
It returns an iterator that applies the check for each of the elements in the iterable.
For example,
py_lst = ["a-1", "b-2", "c-3", "a-4"]
filter(lambda x: "a" in x, py_lst)
print(filter(lambda x: "a" in x, py_lst))
Output:
<filter object at 0x7fd36c1905e0>
Note that the above output is a filter-iterator type object since the filter()
function returns an iterator instead of a list.
We can use the list()
function as shown in the code below to obtain a list.
list(filter(lambda x: "a" in x, py_lst))
Output:
['a-1','a-4']
In the above code, we have used filter()
to find a string with specific values in the list py_list
.
Related Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python