How to Append to Front of a List in Python
- What Is Python List Insert
- Purpose of Python List Insert
- Inserting Element to Specific Index Into the List
- Inserting an Element at the Beginning of the List
- Inserting a Tuple Into the List
-
Python List
insert()
Conclusion
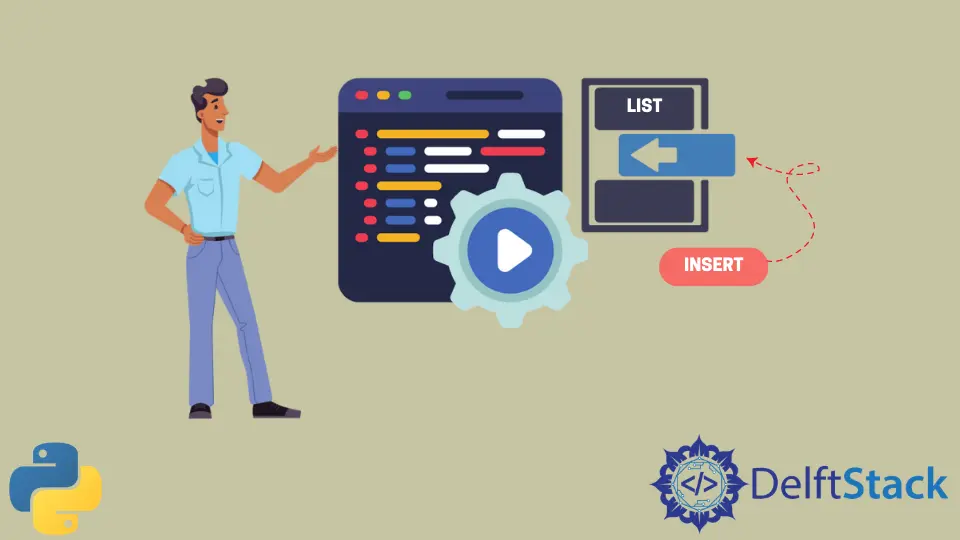
The list
data type provides a flexible way to store and manage collections of items, and you can use a method to insert elements at specific positions within the list. The Python insert into list method is a vital component for developers aiming to precisely manipulate lists in Python programming.
This tutorial will demonstrate different ways on how to insert a given element name into a list in Python.
What Is Python List Insert
In Python, the list insert()
method is a built-in function that allows you to insert an element at a specified position within a list. The method takes two arguments: the index at which you want to insert the element and the inserted element itself.
It’s important to note that the insert()
method does not have a return value; instead, it modifies the list in place.
After insertion, the list’s length increases by 1, and all the elements to the right of the specified index are shifted to accommodate the new element.
Understanding the insert()
Method Syntax
The general syntax for the insert()
method is as follows:
list.insert(index, element)
The list
is the name of the list.
Here’s an overview of the two parameters:
index
: The position at which the element should be inserted. If the index is out of bounds (negative or greater than the length of the list), the element will be inserted at the beginning or the end of the list, respectively.
element
: The value or object to be inserted into the list at the specified index.
Purpose of Python List Insert
The purpose of the Python insert()
method in lists is to allow developers to insert an element at a specific position within the list. This method provides a way to customize the arrangement of elements by specifying the desired index for insertion.
By inserting elements at specific locations, developers can dynamically modify the content of a list of values without the need for complex restructuring. This is particularly useful when working with evolving datasets or when the order of elements is crucial.
Inserting Element to Specific Index Into the List
The insert()
function in Python is used to insert an element at a specified index within a list. This is particularly useful when you want to control the order of elements in the list or insert new data at a specific location.
Example
# Define a list
my_list = [1, 2, 3, 5, 6]
# Specify the index and the element to be inserted
index_to_insert = 3
element_to_insert = 4
# Use the insert() method to insert the element at the specified index
my_list.insert(index_to_insert, element_to_insert)
# Display the modified list
print("Modified List:", my_list)
Step 1: List Definition
Here, we initialize a list named my_list
with some initial elements.
my_list = [1, 2, 3, 5, 6]
Step 2: Index and Element to Insert
We specify the index (index_to_insert
) at which the new element should be inserted and the element itself (element_to_insert
).
index_to_insert = 3
element_to_insert = 4
Step 3: Insertion Using insert()
Method
The insert()
method is then used on the list (my_list
). It takes two arguments: the given index and the element to be inserted. In our example, it inserts the value 4
at index 3
.
my_list.insert(index_to_insert, element_to_insert)
Step 4: Display the Modified List
Finally, we print the modified list to observe the changes.
print("Modified List:", my_list)
Output:
The element 4 has been successfully inserted at index 3
, and the existing elements have shifted accordingly given index three.
Inserting an Element at the Beginning of the List
Inserting an element at the beginning of a Python list is a common operation that can be efficiently accomplished using the list’s insert()
method. This method inserts an element to the first index in the list, and by specifying the index as 0
, you can prepend the element to the list.
Example
# Initialize a list of fruits
fruits = ["apple", "banana", "cherry"]
# New fruit to be added at the beginning
new_fruit = "orange"
# Inserting the new fruit at the beginning of the list
fruits.insert(0, new_fruit)
# Displaying the updated list
print("Updated list of fruits:", fruits)
Step 1: List Initialization
We start by defining a list named fruits
containing a few fruit names.
fruits = ["apple", "banana", "cherry"]
This list currently holds three elements.
Step 2: Defining the New Element
The new_fruit
variable is assigned the value orange
, representing the new fruit we intend to add to the list.
new_fruit = "orange"
Step 3: Inserting at the Beginning
To insert new_fruit
at the beginning of the list, we use the insert()
method. The first argument of insert()
is the index where the element should be inserted, and the second argument is the element itself.
fruits.insert(0, new_fruit)
Here, 0
is the index for the first position in the list. This command places orange
at the start of the list, shifting all other elements one position to the right.
Step 4: Outputting the Result
Finally, we print the updated list to verify our operation.
print("Updated list of fruits:", fruits)
Output:
The output confirms that orange
has been successfully added to the beginning of the list.
Inserting a Tuple Into the List
In Python, the process of inserting a tuple into a list involves using the insert()
method. This technique is valuable when there’s a need to embed a tuple structure within an existing list.
Tuples, being immutable, can encapsulate related data elements. Inserting a tuple into a list allows for the creation of a heterogeneous collection.
Example
# Existing list of employee records
employees = [("John Doe", "Engineering"), ("Jane Smith", "Marketing")]
# New employee record as a tuple
new_employee = ("Emily Davis", "Human Resources")
# Inserting the new employee tuple at a specific index
index = 1
employees.insert(index, new_employee)
# Displaying the updated list
print("Updated Employee List:")
print(employees)
Step 1: List of Tuples Initialization
We start by creating a list named employees
, where each element is a tuple representing an employee record. Each tuple contains the employee’s name and their department.
employees = [("John Doe", "Engineering"), ("Jane Smith", "Marketing")]
This list has two tuples initially.
Step 2: Creating the Tuple to Insert
A new tuple new_employee
is defined, which holds the record for a new employee, Emily Davis
, who works in Human Resources
.
new_employee = ("Emily Davis", "Human Resources")
Step 3: Inserting the Tuple
To insert new_employee
into the employees
list, we use the insert()
method. The index is set to 1
, meaning the new tuple will be placed at the second position in the list (as list indices in Python start from 0
).
employees.insert(index, new_employee)
Step 4: Printing the Updated List
Finally, the updated list is printed, showing that the new employee’s record has been inserted into final list.
print("Updated Employee List:")
print(employees)
Output:
This demonstrates that the tuple ('Emily Davis', 'Human Resources')
has been successfully inserted into the employees
list at the specified index.
Python List insert()
Conclusion
The Python List insert()
method is a crucial tool for developers to manipulate list data structures efficiently and accurately. This article provided a comprehensive guide on using the insert()
method.
It covered the method’s syntax, purpose, and practical examples, including inserting a single element, adding an element to the beginning of the list, and embedding a tuple within a list.
For those exploring more advanced features of the list
data type in or looking to deepen their understanding of Python list manipulation, the next steps involve experimenting with remove an element from a list or append list to another list to achieve more sophisticated data manipulation tasks. Further exploration can also include the integration of these techniques into larger Python projects to appreciate their practical applications in real-world scenarios.
You can also append values to a [2D array]({{relref “/HowTo/Python/append 2d array python.en.md”}}) but it will be covered in another article.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python