How to Remove List Element by Index in Python
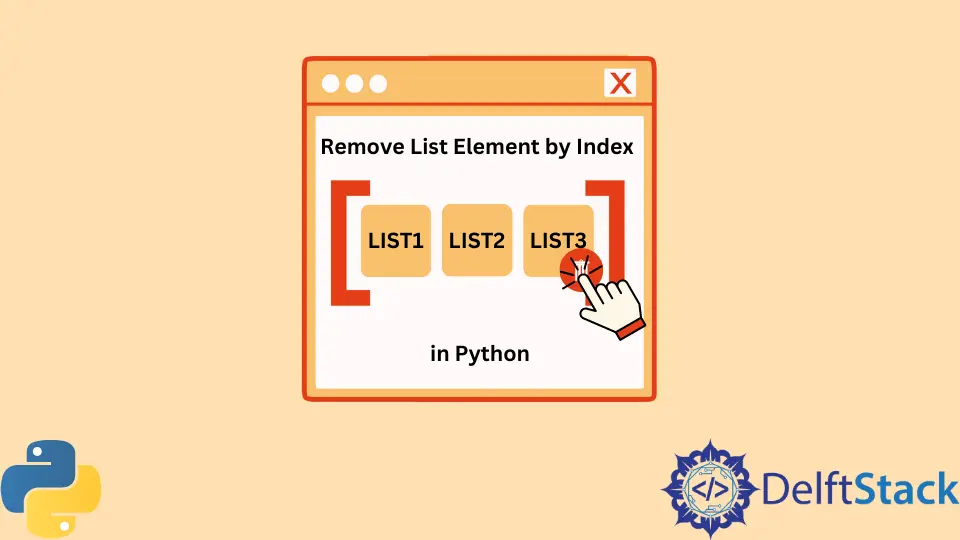
When working with lists in Python, you may find yourself needing to remove elements based on their index. This can be particularly useful when you want to manage the contents of your list dynamically. Fortunately, Python provides two primary methods for this task: the del
keyword and the pop()
function. Both methods are straightforward and efficient, allowing you to manipulate your lists easily.
In this article, we will dive into each method, provide clear examples, and explain how they work. Whether you’re a beginner or looking to refine your skills, understanding how to remove list elements by index is a fundamental part of Python programming.
Using the del Keyword
The del
keyword in Python is a powerful tool that allows you to delete elements from a list by their index. When you use del
, you can remove an element without returning it, which can be useful when you don’t need the value of the removed item. This method directly modifies the original list.
Here’s how you can use the del
keyword to remove an element from a list:
my_list = [10, 20, 30, 40, 50]
del my_list[2]
print(my_list)
Output:
[10, 20, 40, 50]
In this example, we start with a list called my_list
containing five integers. By using del my_list[2]
, we remove the element at index 2, which is the number 30. After executing the del
statement, the list is modified to contain only the remaining elements: 10, 20, 40, and 50.
One of the advantages of using del
is that it allows you to delete multiple items at once by specifying a range. For instance, you could remove items from index 1 to index 3 by using del my_list[1:3]
. However, it’s important to handle potential IndexError
exceptions, which can occur if you try to delete an index that doesn’t exist in the list. Overall, the del
keyword provides a simple and effective way to manage your lists.
Using the pop()
Function
Another method to remove an element from a list by index is the pop()
function. Unlike del
, pop()
not only removes the element but also returns it, which can be useful if you need to use the value after removing it. By default, pop()
removes the last element of the list if no index is specified, but you can also provide a specific index.
Here’s an example of how to use the pop()
function:
my_list = [10, 20, 30, 40, 50]
removed_element = my_list.pop(2)
print(my_list)
print("Removed element:", removed_element)
Output:
[10, 20, 40, 50]
Removed element: 30
In this code snippet, we again start with a list named my_list
. By calling my_list.pop(2)
, we remove the element at index 2, which is 30, and store it in the variable removed_element
. After executing the pop()
function, the modified list now contains 10, 20, 40, and 50. Additionally, we print the removed element, showcasing how pop()
can be beneficial when you need to work with the deleted value.
The pop()
function also allows you to handle situations where you might want to remove and utilize the last element of a list by simply calling my_list.pop()
. If you attempt to pop an index that is out of range, Python will raise an IndexError
, so it’s crucial to ensure the index is valid. This method is particularly handy when you are managing a stack or queue structure in your application.
Conclusion
Removing elements from a list by index in Python is a fundamental skill that can enhance your programming capabilities. By utilizing the del
keyword or the pop()
function, you can efficiently manage and manipulate your lists. Each method has its unique advantages, so understanding when to use one over the other is key to effective list management. Whether you’re cleaning up data, implementing algorithms, or simply organizing information, mastering these techniques will serve you well in your Python journey.
FAQ
-
What is the difference between del and pop() in Python?
del removes an element without returning it, while pop() removes an element and returns its value. -
Can I remove multiple elements from a list using del?
Yes, you can specify a range of indices to remove multiple elements with del. -
What happens if I try to pop from an empty list?
You will receive an IndexError if you attempt to pop from an empty list. -
Are there any performance differences between del and pop()?
Generally, both methods have similar performance, but pop() may be slightly slower due to returning the removed item. -
Can I use del to remove elements from a list of strings?
Yes, del can be used to remove elements from any list type, including lists of strings.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python