How to Fix the ImportError: Cannot Import Name in Python
-
Cause of the
ImportError: cannot import name
Error in Python -
Fix the
ImportError: cannot import name
Error in Python
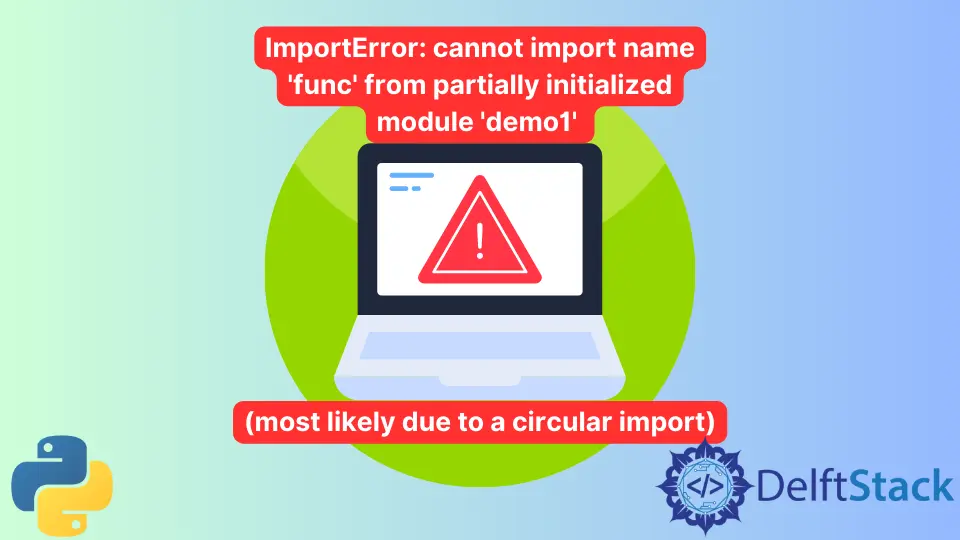
With this explanation, we will learn why we get an error that is ImportError: cannot import name
. We will also learn how to fix this kind of error in Python.
Cause of the ImportError: cannot import name
Error in Python
We can use a couple of ways to fix this error, but before diving into the ways of fixing this error, let’s try to understand why this error occurs and when the ImportError
generally occurs.
An ImportError
in Python occurs if the import
statement within your code experiences difficulty in successfully importing a module.
Such an issue commonly occurs because of a faulty installation of an external library or an invalid path to the module you are trying to import.
The question is raised: when does the ImportError: cannot import name (xyz)
happen? This kind of ImportError
generally occurs when the class you are trying to import is in a circular dependency.
What does that mean? What is a circular dependency? Circular dependency generally occurs when two modules try to import each other simultaneously such that both of them are dependent on each other.
To further explain this, we need to look at an example to understand how and when this error occurs. Let’s say we have a couple of modules: demo1.py
and demo2.py
.
Before moving into the import
statements, let’s look at the contents of these modules and then at the import
statements.
As we can see, the demo2
module has a couple of functions: demo2_func1()
and demo2_func2()
. Similarly, the demo1
module has a single function called demo1_func1()
.
As we can see, within the demo2
module, we are importing demo1_func1
from the module demo1
. Similarly, within the demo1
module, we are trying to import the function called demo2_func2()
, which is a part of the demo2
module.
Let’s focus on the demo2
module.
We can see that the demo2_func1()
function prints some string and then loads the demo1_func1()
function, which we have imported from the demo1
module. When the demo1_func1()
calls, it will print some string.
In the next line, within the demo1_func1()
function, we call the demo2_func2()
method, so we can see the demo2_func2()
method is also part of the demo1
module. We can see both these modules are interdependent.
In this case, when we use a statement like from demo1 import demo1_func1
and from demo2 import demo2_func2
, a circular dependency occurs.
If we execute the demo2
module, then the expected output should be something like this since demo2_func1()
is being called initially, then we move on to the demo1_func1()
function and so on. After execution, we have an ImportError
that says (cannot import name 'demo1_func1'
).
ImportError: cannot import name 'demo1_func1' from partially initialized module 'demo1' (most likely
due to a circular import)
This majorly occurs because we are trying to access the contents of one module from another and vice versa. If we look at the demo2.py
module, we try to import the demo1_func1
function from demo1
before demo1_func1
was initialized; that is when the program begins.
Python is still initializing the contents of the demo1
module, but we are not giving ample time to Python to initialize the demo1_func1
function. We are directly calling it, which is why we encountered this ImportError
.
Fix the ImportError: cannot import name
Error in Python
Now we will see how we can fix this error. We can fix this error in two ways, but we will use the easiest way: to avoid the circular dependency, and Python can do it by itself.
To resolve the problem, we must make certain code changes.
We have to modify the import
statement, and instead of importing the module’s contents, we import the entire module in both files.
The source code of the demo1.py
file:
import demo2
def demo1_func1():
print("demo1_func1")
demo2.demo2_func2()
The code of the demo2.py
file:
import demo1
def demo2_func1():
print("demo2_func1")
demo1.demo1_func1()
def demo2_func2():
print("demo2_func2")
if __name__ == "__main__":
demo2_func1()
Output:
demo2_func1
demo1_func1
demo2_func2
Mostly this error occurs when we are developing the Flask app using the blueprint approach, so if you are creating a Flask structure, you may need to follow this link.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python