How to Get Number of Lines in a File in Python
-
Get Number of Lines in a File in Python Using the
open()
andsum()
Functions -
Get Number of Lines in a File in Python Using the
mmap.mmap()
Method -
Get Number of Lines in a File in Python Using the
file.read()
Method
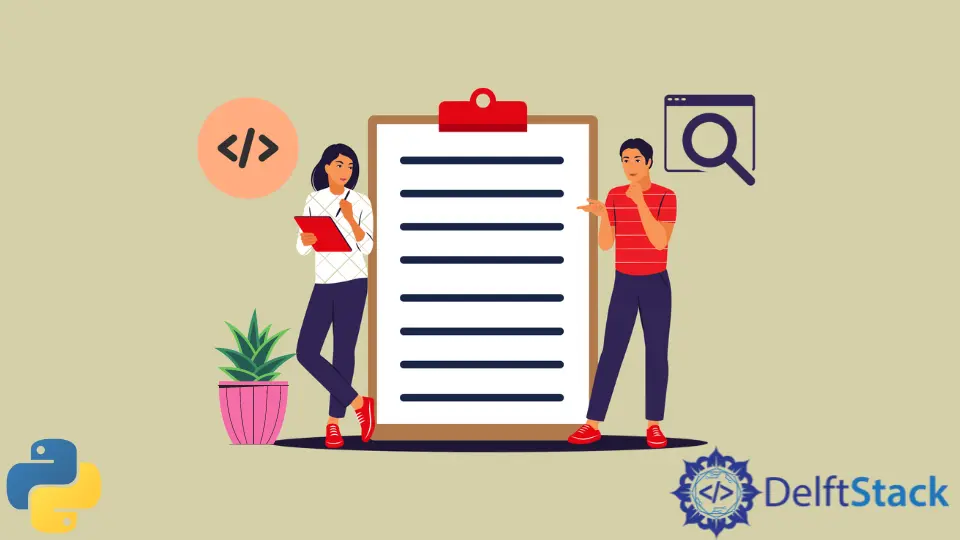
This tutorial will demonstrate various methods to get the total number of lines in a file in Python. To get the total number of lines of a file, we first need to read the data of the file in a buffer, and we can do so by either loading the complete file at once or read the data in small chunks if the file size is large.
We will look at how we can implement both approaches using various methods in Python, which are explained below with example code:
Get Number of Lines in a File in Python Using the open()
and sum()
Functions
A simple way to get the number of lines in a file is by iterating through each line of the file object returned by the open()
function.
The open(file, mode)
function takes file
as input and returns a file object as output. A file
is a path-like object that can be a string or bytes object and contains the file path. The mode
represents the mode we want to open the file, like read, write, append mode, etc.
The below example code demonstrates how to use the for
loop to get the number of lines in a file in Python.
with open("myFolder/myfile.txt") as myfile:
total_lines = sum(1 for line in myfile)
print(total_lines)
Get Number of Lines in a File in Python Using the mmap.mmap()
Method
The mmap.mmap(fileno, length)
method maps length
number of bytes from the file specified by the fileno
and returns a mmap
object. If the value of length
is 0
, the maximum length of the map will be equal to the file size.
We can use the mmap
object returned by the mmap.mmap()
method and then use the mm.readline()
method to access the lines until we reach the end of the file. As we want to load the complete file, we will pass 0
as the length
argument.
Example code:
import mmap
with open("myFolder/myfile.txt", "r+") as myfile:
mm = mmap.mmap(myfile.fileno(), 0)
total_lines = 0
while mm.readline():
total_lines += 1
print(total_lines)
Get Number of Lines in a File in Python Using the file.read()
Method
If the file size is huge and we need a fast way to read the file in small chunks, we can use the file.read()
method to read the data as a byte array into a buffer with the specified size.
The below example code demonstrates how to read the file data into a buffer using the file.read()
method and then iterate through it to get the number of lines:
lines = 0
size = 1024 * 1024
with open(r"C:\test\file.txt", "r+") as myfile:
read_file = myfile.read
buffer = read_file(size)
while buffer:
lines += buffer.count("\n")
buffer = read_file(size)
if lines != 0:
lines += 1
print(lines)
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn