在 Python 中获取文件的行数
-
在 Python 中使用
open()
和sum()
函数获取文件中的行数 -
在 Python 中使用
mmap.mmap()
方法获取文件中的行数 -
在 Python 中使用
file.read()
方法获取文件中的行数
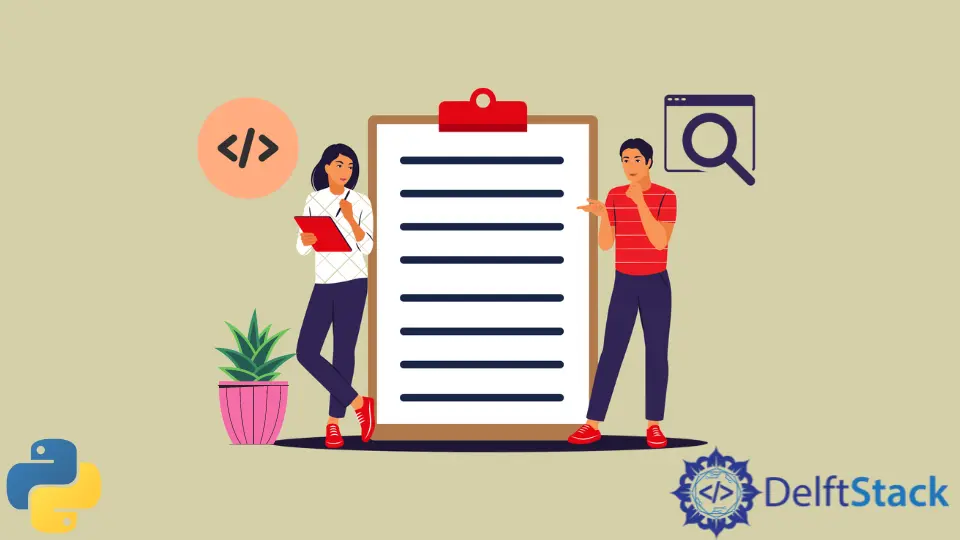
本教程将演示用 Python 获取文件中总行数的各种方法。为了获得文件的总行数,我们首先需要读取缓冲区中的文件数据,我们可以通过一次性加载完整的文件或者在文件大小较大的情况下分小块读取数据来实现。
我们将看看如何使用 Python 中的各种方法来实现这两种方法,下面将用示例代码来解释。
在 Python 中使用 open()
和 sum()
函数获取文件中的行数
获取文件行数的一个简单方法是通过遍历 open()
函数返回的文件对象的每一行。
open(file, mode)
函数以 file
作为输入,并返回一个文件对象作为输出。file
是一个类似路径的对象,可以是字符串或字节对象,包含文件路径。mode
代表我们要打开文件的模式,如读、写、追加模式等。
下面的示例代码演示了如何在 Python 中使用 for
循环来获取文件中的行数。
with open("myFolder/myfile.txt") as myfile:
total_lines = sum(1 for line in myfile)
print(total_lines)
在 Python 中使用 mmap.mmap()
方法获取文件中的行数
mmap.mmap(fileno, length)
方法从 fileno
指定的文件中映射 length
字节数,并返回 mmap
对象。如果 length
的值是 0
,映射的最大长度将等于文件大小。
我们可以使用 mmap.mmap()
方法返回的 mmap
对象,然后使用 mm.readline()
方法访问行,直到到达文件的末端。由于我们要加载完整的文件,我们将传递 0
作为 length
参数。
示例代码:
import mmap
with open("myFolder/myfile.txt", "r+") as myfile:
mm = mmap.mmap(myfile.fileno(), 0)
total_lines = 0
while mm.readline():
total_lines += 1
print(total_lines)
在 Python 中使用 file.read()
方法获取文件中的行数
如果文件的大小很大,我们需要一种快速的方法来读取小块的文件,我们可以使用 file.read()
方法将数据以字节数组的形式读取到一个指定大小的缓冲区中。
下面的示例代码演示了如何使用 file.read()
方法将文件数据读入缓冲区,然后遍历得到行数。
lines = 0
size = 1024 * 1024
with open(r"C:\test\file.txt", "r+") as myfile:
read_file = myfile.read
buffer = read_file(size)
while buffer:
lines += buffer.count("\n")
buffer = read_file(size)
if lines != 0:
lines += 1
print(lines)
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn