How to Get Absolute Path in Python
-
Use
abspath()
to Get the Absolute Path in Python -
Use the Module
pathlib
to Get the Absolute Path in Python
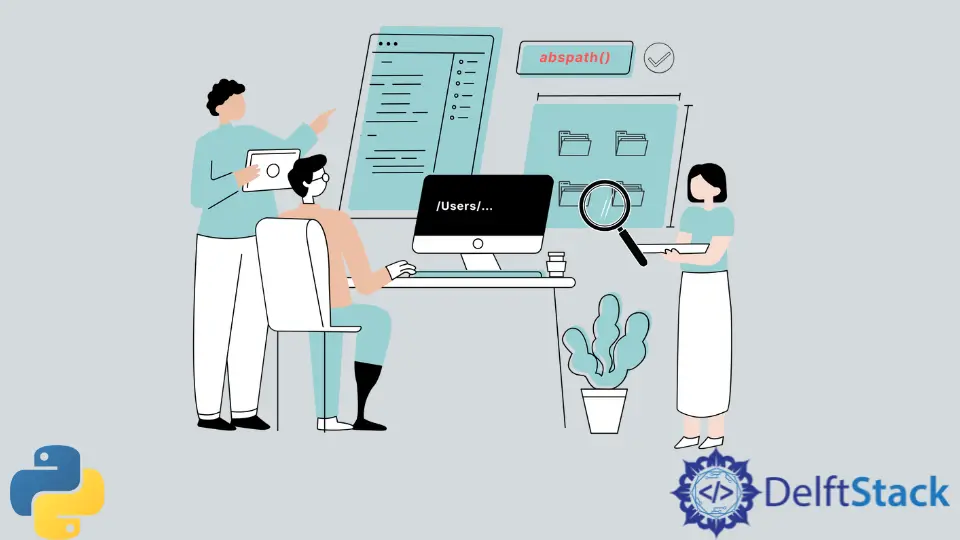
This tutorial will demonstrate how to get the absolute path of a file or a folder in Python.
Use abspath()
to Get the Absolute Path in Python
Under the Python module os
are useful utility functions and properties that manipulate and access file paths under the os.path
property. To get the absolute path using this module, call path.abspath()
with the given path to get the absolute path.
import os
simp_path = "demo/which_path.docx"
abs_path = os.path.abspath(simp_path)
print(abs_path)
The output of the abspath()
function will return a string value of the absolute path relative to the current working directory.
Output:
/Users/user/python/demo/which_path.docx
Use the Module pathlib
to Get the Absolute Path in Python
The Python module pathlib
offers similar functions as os.path
and contains classes representing file paths with their corresponding properties and functions used for path manipulation and access.
To get the absolute path using pathlib
, import the Path
class from the pathlib
module and use the Path.absolute()
function of that class to determine the absolute path of a given file or folder.
from pathlib import Path
fpath = Path("sample2.py").absolute()
print(fpath)
Setting the absolute path as a parameter is also supported and will print it straightforwardly instead of appending the root folders and making it redundant.
from pathlib import Path
fpath = Path("/Users/user/python/sample2.py").absolute()
print(fpath)
Both instances will produce the same output:
/Users/user/python/sample2.py
In summary, there are two easy ways to get the absolute path of a file or a folder in Python under the modules os
and pathlib
. Performance-wise, both solutions are relatively fast, and it’s only a matter of preference as to which solution the developer wants to use.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn