Float Division in Python
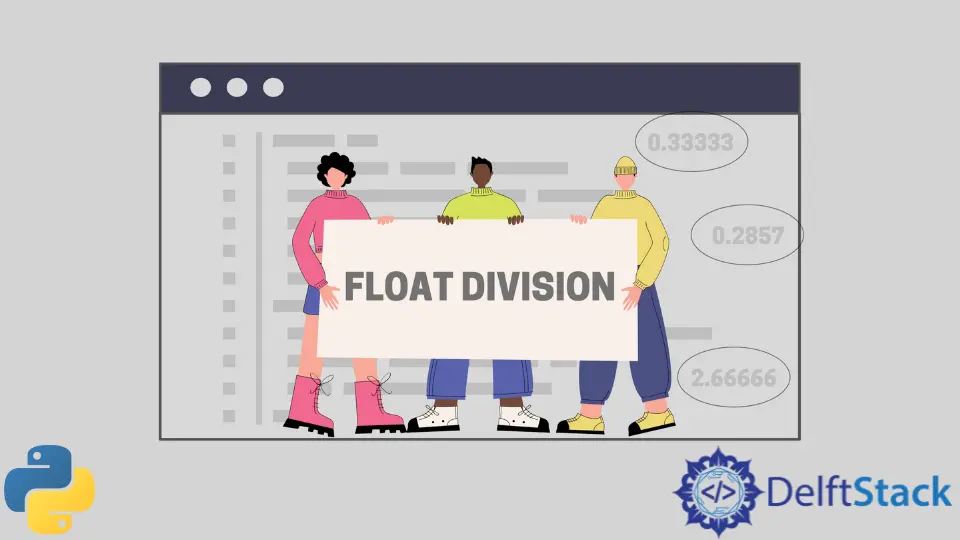
Float division refers to a floating-point approximation of the result of a division or, mathematically speaking, the quotient. In comparison, integer division refers to an integer approximation of the quotient. Essentially, the floating part is completely removed from the result.
In statically-typed programming languages such as C
, C++
, Go
, Scala
, and Java
, floating division depends on the data type of the variables and the numerical values. Whereas, in the case of dynamically-typed programming languages such as Python
, Groovy
, PHP
, Lua
, and JavaScript
, it depends on the numerical values (since variables don’t have a fixed data type and can be reused for a different type of values).
As stated above, Python is a dynamically-typed programming language. In this article, we will learn to perform float division in Python with the help of relevant examples.
Different Ways to Perform Float Division in Python
Essentially, Python has two ways to perform float division, and we will try to learn them through some examples. Note that the examples provided will try to cover most of the possible cases.
Using Default Division
In Python, the division performed by the division operation (/
) is, by default, float division. To achieve integer division, one can use the //
operator. Refer to the following code for some examples.
print(1 / 3)
print(2 / 7)
print(8 / 3)
print(9 / 4)
print(11 / 10)
print(121.0 / 8.0)
print(8 / 121)
print(10 / 11)
print(4.0 / 9)
print(3 / 8.0)
Output:
0.3333333333333333
0.2857142857142857
2.6666666666666665
2.25
1.1
15.125
0.06611570247933884
0.9090909090909091
0.4444444444444444
0.375
Using Float Conversion
In Python and all the other programming languages, division of a float number (float/int
) or division by a float number (int/float
) or division of a float number by a float number (float/float
), yields a floating-point result or quotient. Note that the same concept applies to the double
datatype.
In Python, we can convert an integer or a string representing a number, both integer and float number, to a floating-point number with the help of the float()
function. Let us look at some examples to understand how we can perform float division with the help of float conversion.
print(float(1) / 3) # float / int
print(float("2") / 7) # float / int
print(8 / float(3)) # int / float
print(9 / float("4")) # int / float
print(float(11) / float(10)) # float / float
print(float("121") / float("8")) # float / float
print(float("8.0") / float("121.0")) # float / float
print(float("10.00000") / 11) # float / int
print(float("4") / float(9)) # float / float
print(float(3) / float("8")) # float / float
Output:
0.3333333333333333
0.2857142857142857
2.6666666666666665
2.25
1.1
15.125
0.06611570247933884
0.9090909090909091
0.4444444444444444
0.375