How to Capture Exception Message in Python
-
Capture Exception Message in Python Using
logger.exception()
Method -
Capture Exception Message in Python Using
logger.error()
Method -
Capture Exception Message in Python Using the
print()
Method
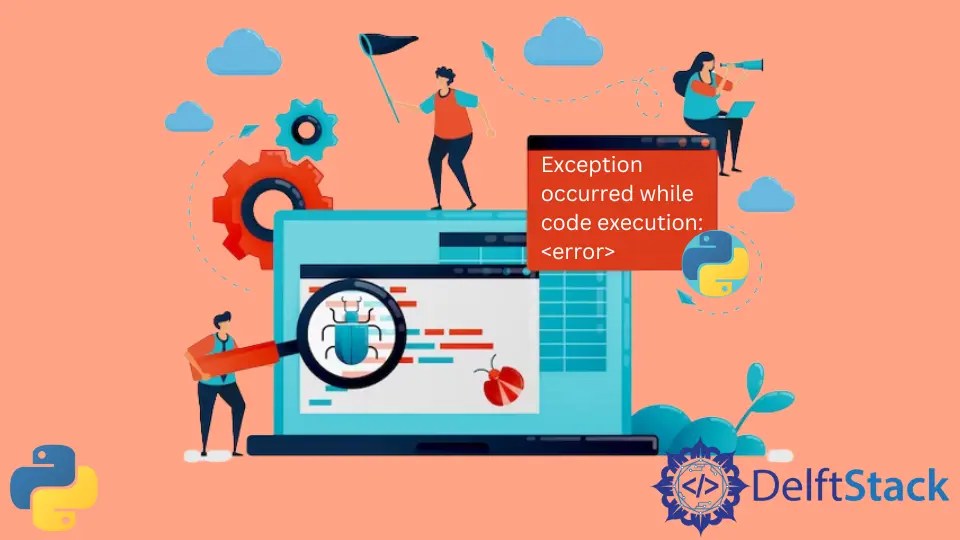
This tutorial will explain different ways to capture exception messages in Python. The exception handling is used to respond to the exceptions that occur during the execution of the program. It is important to handle exceptions; otherwise, a program will crash whenever some exception occurs.
The try ... except
statement handles exceptions in Python. But we also need to capture the details of exception that occurs during the code execution, so that it can be solved. The various methods that can be used to capture the exception messages in Python are explained below.
Capture Exception Message in Python Using logger.exception()
Method
The logger.exception()
method returns an error message and the log trace, which includes the details like the code line number at which the exception has occurred. The logger.exception()
method must be placed within except
statement; otherwise, it will not work correctly in any other place.
The below code example demonstrates the proper use of the logger.exception()
method with try ... except
statement to capture the exception message in Python.
import logging
logger = logging.getLogger()
try:
x = 1 / 0
except Exception as e:
logger.exception("Exception occurred while code execution: " + str(e))
Output:
Exception occurred while code execution: division by zero
Traceback (most recent call last):
File "<ipython-input-27-912703271615>", line 5, in <module>
x = 1/0
ZeroDivisionError: division by zero
Capture Exception Message in Python Using logger.error()
Method
The logger.error()
method returns the error message only whenever exceptions occur within the try
block. The code example of how the logger.error()
method can capture exception messages in Python is given below.
import logging
logger = logging.getLogger()
try:
x = 1 / 0
except Exception as e:
logger.error("Exception occurred while code execution: " + str(e))
Output:
Exception occurred while code execution: division by zero
As we can notice in the above example, the str(e)
method only gets the exception message from the exception e
object and not the exception type.
The repr(e)
method can be used to get the exception type along the exception message. The below code example demonstrate the use and output of the repr(e)
method:
import logging
logger = logging.getLogger()
try:
x = 1 / 0
except Exception as e:
logger.error("Exception occurred while code execution: " + repr(e))
Output:
Exception occurred while code execution: ZeroDivisionError('division by zero',)
Capture Exception Message in Python Using the print()
Method
We can also use the print()
method to print the exception message. The example code below demonstrates how to capture and print an exception message in Python using the print()
method.
Example code:
try:
x = 1 / 0
except Exception as e:
print("Exception occurred while code execution: " + repr(e))
Output:
Exception occurred while code execution: ZeroDivisionError('division by zero',)