Python 中的异常消息
Muhammad Waiz Khan
2023年1月30日
Python
Python Exception
-
使用
logger.exception()
方法捕获 Python 中的异常消息 -
在 Python 中使用
logger.error()
方法捕获异常消息 -
在 Python 中使用
print()
方法捕获异常消息
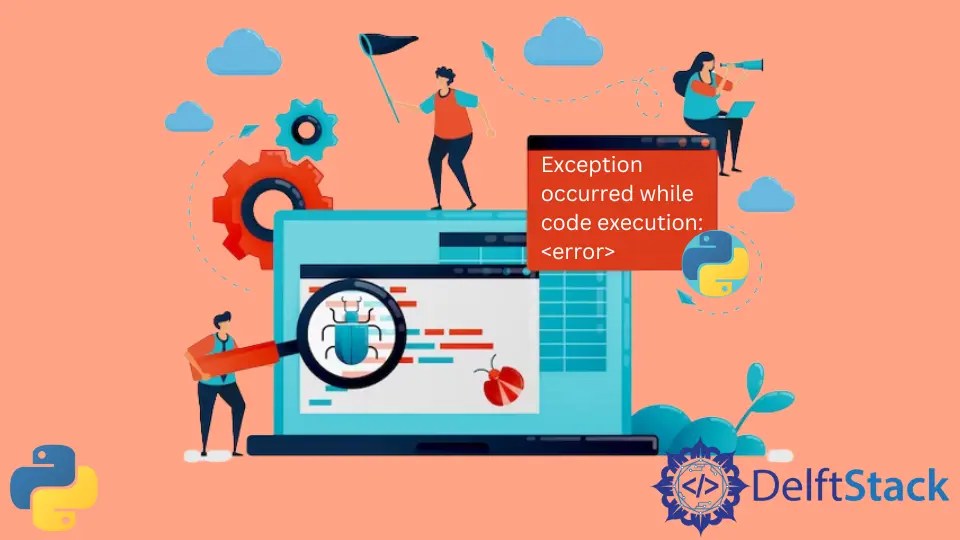
本教程将讲解在 Python 中捕获异常消息的不同方法。异常处理用于响应程序执行过程中出现的异常。处理异常是很重要的,否则每当出现一些异常时,程序就会崩溃。
try ... except
语句在 Python 中处理异常。但是我们还需要捕捉代码执行过程中发生的异常细节,以便解决。下面解释了在 Python 中可以用来捕获异常消息的各种方法。
使用 logger.exception()
方法捕获 Python 中的异常消息
logger.exception()
方法返回一个错误信息和日志跟踪,其中包括发生异常的代码行号等细节。logger.exception()
方法必须放在 except
语句中,否则在其他地方将无法正常工作。
下面的代码示例演示了正确使用 logger.exception()
方法和 try ... except
语句来捕获 Python 中的异常信息。
import logging
logger = logging.getLogger()
try:
x = 1 / 0
except Exception as e:
logger.exception("Exception occurred while code execution: " + str(e))
输出:
Exception occurred while code execution: division by zero
Traceback (most recent call last):
File "<ipython-input-27-912703271615>", line 5, in <module>
x = 1/0
ZeroDivisionError: division by zero
在 Python 中使用 logger.error()
方法捕获异常消息
logger.error()
方法只在 try
块内发生异常时返回错误信息。下面给出了 Python 中 logger.error()
方法如何捕获异常信息的代码示例。
import logging
logger = logging.getLogger()
try:
x = 1 / 0
except Exception as e:
logger.error("Exception occurred while code execution: " + str(e))
输出:
Exception occurred while code execution: division by zero
在上面的例子中,我们可以注意到,str(e)
方法只从异常 e
对象中获取异常消息,而不是异常类型。
repr(e)
方法可以用来获取异常消息的同时获取异常类型。下面的代码示例演示了 repr(e)
方法的使用和输出。
import logging
logger = logging.getLogger()
try:
x = 1 / 0
except Exception as e:
logger.error("Exception occurred while code execution: " + repr(e))
输出:
Exception occurred while code execution: ZeroDivisionError('division by zero',)
在 Python 中使用 print()
方法捕获异常消息
我们也可以使用 print()
方法来打印异常消息。下面的示例代码演示了如何在 Python 中使用 print()
方法捕获和打印异常消息。
示例代码:
try:
x = 1 / 0
except Exception as e:
print("Exception occurred while code execution: " + repr(e))
输出:
Exception occurred while code execution: ZeroDivisionError('division by zero',)
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe