How to Fix Python Error: Object Is Not Callable
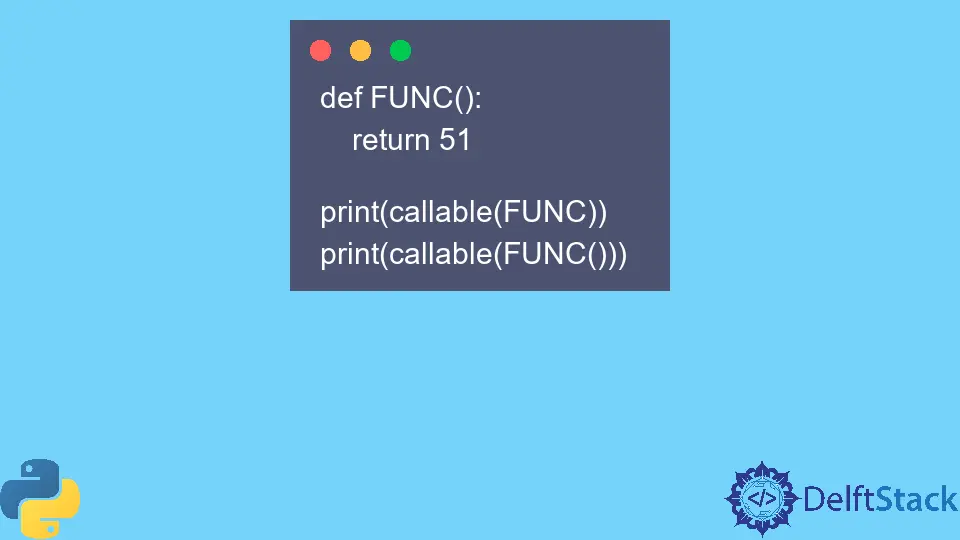
We will discuss the type error object is not callable
and see how we can fix it. We also discuss utilizing the callable function to check whether the objects are callable or not in Python.
Fix Python Error object is not callable
When we look at this error (object is not callable
), we probably need to understand what is happening within the Python script. For example, we have a few lines of code to demonstrate the problem and how we fix it.
Error Code:
v = 5
g = 9
int = 3
print(int(g / v * int))
Output:
TypeError: 'int' object is not callable
We have three variables v
, g,
and int
. We are experiencing this problem because we defined a variable called int
that is a function.
The int
is a Python built-in function which is why we can not use the int
as a variable name; therefore, when the Python script executes, it is looking to call the variable name int
. The int
kind of restricted name within Python, and as a result, it will show you this error when we use it as a variable.
We fixed the error by changing the variable name and running this script again.
Fixed Code:
v = 5
g = 9
d = 3
print(int(g / v * d))
Output:
5
Remember that when running any program with a function inside your Python script, you should never put that function as a variable name because you will face the same problem. The same problem happens with strings, floats, etc.
If you are getting this error, you first should check whether any of your variables calls a function name or not. We cannot call a variable that stores data type values like in the following code example.
Error Code:
x = "josh"
x()
Output:
TypeError: 'str' object is not callable
the callable()
Function in Python
If an object can be called like a function, the callable()
function returns True
and accepts one parameter, which can be any object.
For example, if we have a variable that is x
equals 42
, this variable refers to an integer number in memory. We can pass x
into the callable()
function and print the result immediately to see whether it returns a true
or a false
value.
When executing this, the return value is false
because the integer number 42
is not callable.
Example Code:
x = 42
print(callable(x))
Output:
False
Let’s check what happens if the callable()
function calls itself; we type callable inside the callable()
function and print this. We will see the result is True
, and we can also pass the print
function, which is also obviously callable.
print(callable(callable))
print(callable(print))
Output:
True
True
Suppose we define our custom objects, not built-in objects, where they are callable or not. Let’s create a function called FUNC()
that returns something.
We pass this function name into the callable()
function and print the output, and this is callable. If we call the return value using FUNC()
, that will be an integer and pass it into the callable()
function. The output will return False
, which is not callable because we can not call an integer that we already look at this.
Example:
def FUNC():
return 51
print(callable(FUNC))
print(callable(FUNC()))
Output:
True
False
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python