How to Solve Algebraic Equations Using Python
-
Solve Algebraic Equations in One Variable Using the
solve()
Method From theSymPy
Package -
Solve Algebraic Equations in Multiple Variables Using the
SymPy
Package - Solving Algebraic Equations in Two Multiple Variables
- Solving Algebraic Equations in Three Multiple Variables
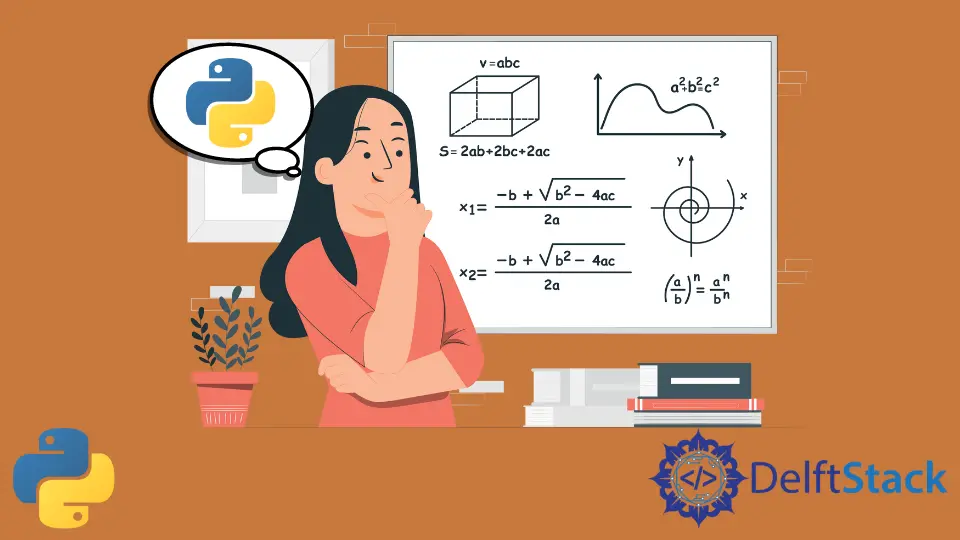
Python has a library for symbolic mathematics, namely, SymPy
. This library contains utilities for solving complex mathematical problems and concepts such as matrices, calculus, geometry, discrete mathematics, integrals, cryptography, algebra, etc.
We can use this library to solve algebraic equations. This article will show how to use SymPy
to solve algebraic equations in Python.
Following are some ways using which we can install the SymPy
module on our machines.
Using the pip
Package Manager to Install Sympy
Use the following command to install the SymPy
package using pip
.
pip install sympy
or
pip3 install sympy
Using Anaconda to Install Sympy
Anaconda is a free Python distribution that includes the SymPy
library in its environment by default. One can update the existing version using the following command.
conda update sympy
To get a detailed overview of the methods discussed above and some other available methods to install the SymPy
library, refer to the official documentation here.
Solve Algebraic Equations in One Variable Using the solve()
Method From the SymPy
Package
The SymPy
library has a solve()
function that can solve algebraic equations. This function accepts the following main arguments.
f
: An algebraic equation.symbols
: The variables for which the equation has to be solved.dict
: A boolean flag for returning a list of solutions mappings.set
: A boolean flag for a list of symbols and set of tuples of solutions.check
: A boolean flag for testing the obtained solutions in the algebraic expression.minimal
: A boolean flag for fast and minimal testing of the solution.
Now that we are done with some brief theory, let us learn how to use this solve()
method to solve algebraic equations with the help of some examples. Refer to the following code for the same.
from sympy.solvers import solve
from sympy import Symbol
x = Symbol("x")
print(solve(x ** 2 - 1, x))
print(solve(x ** 3 + x ** 2 + x + 1, x))
print(solve(x ** 3 - 0 * x ** 2 + 4 * x - 5, x))
Output:
[-1, 1]
[-1, -i, i]
[1, -1/2 - √19i / 2, -1/2 + √19i / 2]
Solve Algebraic Equations in Multiple Variables Using the SymPy
Package
To solve algebraic equations in multiple variables, we need multiple equations.
For example, we need at least two such equations if we have to solve algebraic equations in two variables. In solving algebraic equations in three variables, we need at least three such equations.
And solving such equations, we need three utilities, namely, symbols
, Eq
, solve
, from the SymPy
module.
The symbols
function transforms strings of variables into instances of the Symbol
class.
The Eq
class represents an equal relationship between two objects, specifically expressions. For example, if we have an equation x + y = 3
, then x + y
and 3
are the two objects or expressions. For x + y - 5 = x + 6
, x + y - 5
and x + 6
are the two objects.
And, the solve()
method uses the above two to solve the equations.
Let’s see how we can use these utilities to solve algebraic equations in two and three variables with the help of some relevant examples.
Solving Algebraic Equations in Two Multiple Variables
To understand how to solve algebraic equations in two values using the utilities discussed above, we will consider the following two examples.
Example 1:
x + y = 5
x - y = 5
Example 2:
2 * x + 4 * y = 10
4 * x + 2 * y = 30
Refer to the following Python code for the first example.
from sympy import symbols, Eq, solve
x, y = symbols("x y")
equation_1 = Eq((x + y), 5)
equation_2 = Eq((x - y), 5)
print("Equation 1:", equation_1)
print("Equation 2:", equation_2)
solution = solve((equation_1, equation_2), (x, y))
print("Solution:", solution)
Output:
Equation 1: Eq(x + y, 5)
Equation 2: Eq(x - y, 5)
Solution: {x: 5, y: 0}
Refer to the following Python code for the second example.
from sympy import symbols, Eq, solve
x, y = symbols("x y")
equation_1 = Eq((2 * x + 4 * y), 10)
equation_2 = Eq((4 * x + 2 * y), 30)
print("Equation 1:", equation_1)
print("Equation 2:", equation_2)
solution = solve((equation_1, equation_2), (x, y))
print("Solution:", solution)
Output:
Equation 1: Eq(2*x + 4*y, 10)
Equation 2: Eq(4*x + 2*y, 30)
Solution: {x: 25/3, y: -5/3}
Solving Algebraic Equations in Three Multiple Variables
To understand how to solve algebraic equations in three values using the utilities discussed above, we will consider the following two examples.
Example 1:
x + y + z = 5
x - y + z = 5
x + y - z = 5
Example 2:
2 * x - 4 * y + 6 * z = 10
4 * x + 2 * y + 6 * z = 30
4 * x + 2 * y - 10 * z = 50
Refer to the following Python code for the first example.
from sympy import symbols, Eq, solve
x, y, z = symbols("x y z")
equation_1 = Eq((x + y + z), 5)
equation_2 = Eq((x - y + z), 5)
equation_3 = Eq((x + y - z), 5)
print("Equation 1:", equation_1)
print("Equation 2:", equation_2)
print("Equation 3:", equation_3)
solution = solve((equation_1, equation_2, equation_3), (x, y, z))
print("Solution:", solution)
Output:
Equation 1: Eq(x + y + z, 5)
Equation 2: Eq(x - y + z, 5)
Equation 3: Eq(x + y - z, 5)
Solution: {x: 5, z: 0, y: 0}
Refer to the following Python code for the second example.
from sympy import symbols, Eq, solve
x, y, z = symbols("x y z")
equation_1 = Eq((2 * x - 4 * y + 6 * z), 10)
equation_2 = Eq((4 * x + 2 * y + 6 * z), 30)
equation_3 = Eq((4 * x + 2 * y - 10 * z), 50)
print("Equation 1:", equation_1)
print("Equation 2:", equation_2)
print("Equation 3:", equation_3)
solution = solve((equation_1, equation_2, equation_3), (x, y, z))
print("Solution:", solution)
Output:
Equation 1: Eq(2*x - 4*y + 6*z, 10)
Equation 2: Eq(4*x + 2*y + 6*z, 30)
Equation 3: Eq(4*x + 2*y - 10*z, 50)
Solution: {x: 37/4, z: -5/4, y: 1/4}