How to Calculate Time Elapsed in Python
-
Calculate Elapsed Time of a Function With
time()
Function oftime
Module in Python -
Calculate Elapsed Time of a Function With
perf_counter()
Function oftime
Module in Python -
Calculate Elapsed Time of a Function With
process_time()
Function oftime
Module in Python
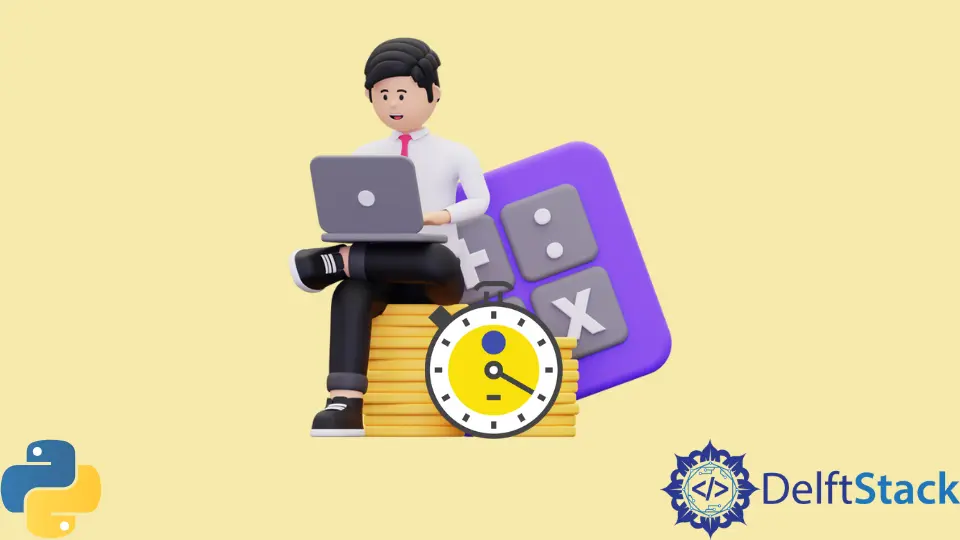
In this tutorial, we will discuss methods to calculate the execution time of a program in Python.
The time
module is a built-in module that contains many time-related functions. Several methods inside the time module can be used to calculate the execution time of a program in Python. These methods are discussed below.
Calculate Elapsed Time of a Function With time()
Function of time
Module in Python
The time()
function gives us the current time in seconds. It returns a float value that contains the current time in seconds. The following code example shows us how we can calculate the execution time of a function with the time()
function in Python.
import time
start = time.time()
print("The time used to execute this is given below")
end = time.time()
print(end - start)
Output:
The time used to execute this is given below
0.00011444091796875
In the above code, we first initialize the start
variable that contains the starting time using the time()
function and then initialize the end
variable after the print()
statement using the time()
function. We then calculate the total execution time by subtracting the start
from the end
.
Calculate Elapsed Time of a Function With perf_counter()
Function of time
Module in Python
The perf_counter()
function gives the most accurate measure of the system time. The perf_counter()
function returns system-wide time and takes the sleep time into account. The perf_counter()
function can also be used to calculate the execution time of a function. The following code example shows us how we can calculate the execution time of a function with the perf_counter()
function in Python.
import time
start = time.perf_counter()
print("This time is being calculated")
end = time.perf_counter()
print(end - start)
Output:
This time is being calculated
0.00013678300001629395
In the above code, we first initialize the start
variable that contains the starting time using the perf_counter()
function and then initialize the end
variable after the print()
statement using the perf_counter()
function. We then calculate the total execution time by subtracting the start
from the end
.
Calculate Elapsed Time of a Function With process_time()
Function of time
Module in Python
The perf_counter()
function is affected by other programs running in the background on the machine. It also counts the sleep time. So, it is not ideal for measuring the execution time of a program.
The best practice for using the perf_counter()
function is to run it several times, and then the average time would give a reasonably accurate estimate of the execution time.
Another approach would be to use the process_time()
function. The process_time()
function is specifically designed to estimate the execution time of a program. It is not affected by other programs running in the background on the machine. It also does not count the sleep time.
The process_time()
function returns a float value that contains the sum of the system and the user CPU time of the program. The following code example shows us how we can calculate the execution time of a function with the process_time()
function in Python.
import time
start = time.process_time()
print("This time is being calculated")
end = time.process_time()
print(end - start)
Output:
This time is being calculated
0.000991254000000108
In the above code, we first initialize the start
variable that contains the starting time using the process_time()
function and then initialize the end
variable after the print()
statement using the process_time()
function. We then calculate the total execution time by subtracting the start
from the end
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn