How to Wait 5 Seconds in Python
-
Use the
time.sleep()
Function to Wait 5 Seconds in Python -
Use the
threading
Library With theEvent
Class to Wait 5 Seconds in Python -
Use the
threading
Library With theTimer()
Function to Wait 5 Seconds in Python -
Use
asyncio.sleep()
for Asynchronous Code to Wait 5 Seconds in Python -
Use the
sched
Module to Wait 5 Seconds in Python - Conclusion
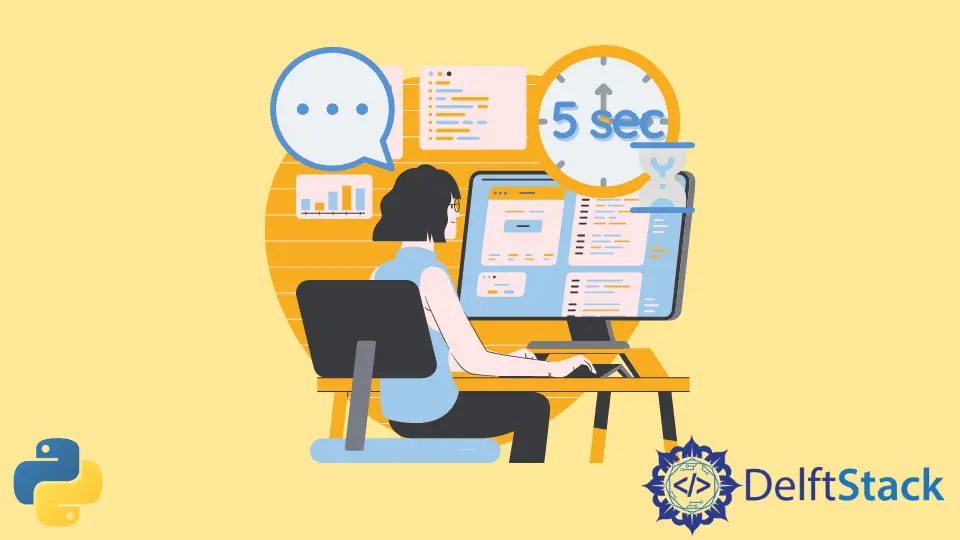
Introducing a delay in Python is a common requirement, whether for creating interactive applications, managing concurrency, or working with asynchronous code. In this article, we’ll explore various methods to wait for 5 seconds in Python, covering different scenarios and use cases.
Use the time.sleep()
Function to Wait 5 Seconds in Python
The time.sleep()
function is part of the time
module in Python. It enables you to suspend the execution of your program for a specified number of seconds.
This can be particularly useful in scenarios where you need to introduce delays between certain actions, create timers, or simulate real-time processes.
The basic syntax for using time.sleep()
is as follows:
import time
time.sleep(seconds)
Here, seconds
represents the duration of the pause in seconds. It can be a floating-point number for sub-second precision.
Let’s walk through a simple example where we want to print a message, wait for 5 seconds, and then print another message:
import time
print("Hello, waiting for 5 seconds...")
time.sleep(5)
print("Done waiting! Now back to work.")
Output:
Hello, waiting for 5 seconds...
Done waiting! Now back to work.
In this example, we begin by importing the time
module. We then print an initial message using print()
.
The time.sleep(5)
line introduces a 5-second delay, pausing the execution of the program. After the delay, the program proceeds to print the second message.
This illustrates how time.sleep()
can be used to introduce controlled pauses in a script, which is useful for various applications such as managing timing in simulations or coordinating actions in a program.
Handle Interruptions With Signal Handling
While time.sleep()
is a straightforward way to introduce delays, it’s important to note that it can be interrupted by signals, such as keyboard interrupts Ctrl+C).
If your script is intended to run continuously, you might want to consider handling interruptions gracefully to ensure a smooth user experience.
import time
import signal
import sys
def graceful_exit(signum, frame):
print("\nReceived interrupt signal. Exiting gracefully.")
sys.exit(0)
signal.signal(signal.SIGINT, graceful_exit)
print("Hello, waiting for 5 seconds...")
time.sleep(5)
print("Done waiting! Now back to work.")
In this modified example, we introduce a signal handler graceful_exit
to handle interruptions caused by the SIGINT
signal (Ctrl+C). The signal.signal(signal.SIGINT, graceful_exit)
line registers this handler.
When the program receives an interrupt signal, it gracefully exits by printing a message and calling sys.exit(0)
. This ensures that interruptions are handled in a user-friendly manner, preventing abrupt terminations and allowing the program to clean up before exiting.
Output:
Hello, waiting for 5 seconds...
Done waiting! Now back to work.
Additionally, if you interrupt the script during the 5-second wait (by pressing Ctrl+C), the output will be:
Hello, waiting for 5 seconds...
^C
Received interrupt signal. Exiting gracefully.
Use the threading
Library With the Event
Class to Wait 5 Seconds in Python
In Python, the threading
library offers a versatile approach to introduce delays and manage concurrency. One method involves using the Event
class from this library to create a synchronization point.
The threading.Event
class provides a simple mechanism for threads to communicate and synchronize their activities. It is particularly useful for scenarios where one thread needs to wait for a specific event to occur before proceeding.
By utilizing its wait()
method, we can effectively pause the program execution for a specified time.
Here’s the syntax:
event = threading.Event()
event.wait(seconds)
In this context, seconds
represents the duration for which the program will be paused.
Let’s proceed with a complete working example:
import threading
event = threading.Event()
print("Before Delay")
event.wait(5)
print("After delay")
In this example, we start by importing the threading
module. We then create an instance of the Event
class with event = threading.Event()
.
The print("Before Delay")
statement marks the point before the delay. We then invoke event.wait(5)
, instructing the program to wait for 5 seconds.
Finally, the program resumes execution with the print("After delay")
statement.
Output:
Before Delay
After delay
Use the threading
Library With the Timer()
Function to Wait 5 Seconds in Python
The threading
library in Python provides another method to introduce delays using the Timer()
function. Unlike the Event
class, Timer()
allows us to create a timer that executes a function after a specified duration.
The Timer()
function takes two arguments: the time delay and the function to be executed. Here’s the syntax:
t = Timer(seconds, fun)
t.start()
In this context, seconds
represents the delay duration, and fun
is the function to be executed after the specified time.
Let’s proceed with a complete working example:
from threading import Timer
def fun():
print("After Delay")
print("Before Delay")
t = Timer(5, fun)
t.start()
In this example, we first import the Timer
class from the threading
module. We then define a function fun()
that will be executed after the delay.
The print("Before Delay")
statement marks the starting point, and we create a Timer
object t
with a 5-second delay and the function fun
. The timer is initiated with t.start()
, and after 5 seconds, the fun()
function is executed.
When you run this Python script, you’ll observe the following output:
Before Delay
After Delay
Use asyncio.sleep()
for Asynchronous Code to Wait 5 Seconds in Python
In Python, when dealing with asynchronous code, the asyncio
library plays a significant role. To introduce a delay in such scenarios, we can use the asyncio.sleep()
function. This method is particularly useful when working with asynchronous tasks and coroutines.
The asyncio.sleep()
function allows asynchronous tasks to pause their execution for a specified duration. The syntax is as follows:
asyncio.sleep(seconds)
Here, seconds
represents the duration for which the asynchronous code will be paused.
Let’s proceed with a complete working example:
import asyncio
async def main():
print("Before Delay")
await asyncio.sleep(5)
print("After Delay")
asyncio.run(main())
In this example, we begin by importing the asyncio
module. We define an asynchronous function main()
that contains the asynchronous code to execute. The print("Before Delay")
statement marks the point before the delay.
We then use await asyncio.sleep(5)
to pause the asynchronous execution for 5 seconds. Finally, the program resumes with print("After Delay")
.
When you run this Python script, you’ll observe the following output:
Before Delay
After Delay
Note that the above example works for Python 3.7 and below. This is because the execution of such functions was altered in further versions.
Use the sched
Module to Wait 5 Seconds in Python
Introducing a delay in Python can also be accomplished using the sched
module. This module provides a general-purpose event scheduler, allowing us to schedule the execution of functions with a specified delay.
The sched
module operates by maintaining a queue of events, each associated with a specific time. The syntax involves creating a scheduler instance, scheduling an event, and running the scheduler.
Here’s a simplified representation:
# Create a scheduler instance
scheduler = sched.scheduler(time.time, time.sleep)
# Schedule an event after a specified delay
scheduler.enter(seconds, priority, function, argument)
# Run the scheduler
scheduler.run()
Here, seconds
represents the delay duration, priority
indicates the event’s priority, function
is the function to execute, and argument
is an optional argument to pass to the function.
Let’s delve into a complete working example:
import sched
import time
def delayed_function():
print("After Delay")
scheduler = sched.scheduler(time.time, time.sleep)
print("Before Delay")
scheduler.enter(5, 1, delayed_function, ())
scheduler.run()
In this example, we start by importing the sched
and time
modules. We define a function delayed_function()
containing the code to execute after the delay.
The print("Before Delay")
statement marks the point before the delay. We then create a scheduler instance using sched.scheduler(time.time, time.sleep)
.
Next, we schedule the delayed_function
to be executed after a 5-second delay using scheduler.enter(5, 1, delayed_function, ())
. The parameters indicate the delay duration, priority, the function to execute, and any optional arguments.
Finally, we run the scheduler with scheduler.run()
.
Output:
Before Delay
After Delay
Conclusion
This article has equipped you with diverse strategies to introduce a 5-second delay in Python, catering to various scenarios. From the time.sleep()
function for synchronous code, the asyncio.sleep()
function in asynchronous environments, the threading
library, and the sched
module, you now have a toolbox of techniques at your disposal.
Whether you are developing interactive applications, managing concurrent threads, or dealing with asynchronous tasks, the methods presented here provide valuable options to suit your programming needs. Consider the specific requirements of your project and the nature of your code to choose the most fitting approach.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn