How to Sleep Milliseconds in Python
- Understanding the Time Module
- Using the Sleep Function
- Implementing Millisecond Sleep in a Loop
- Using Sleep with Asynchronous Programming
- Conclusion
- FAQ
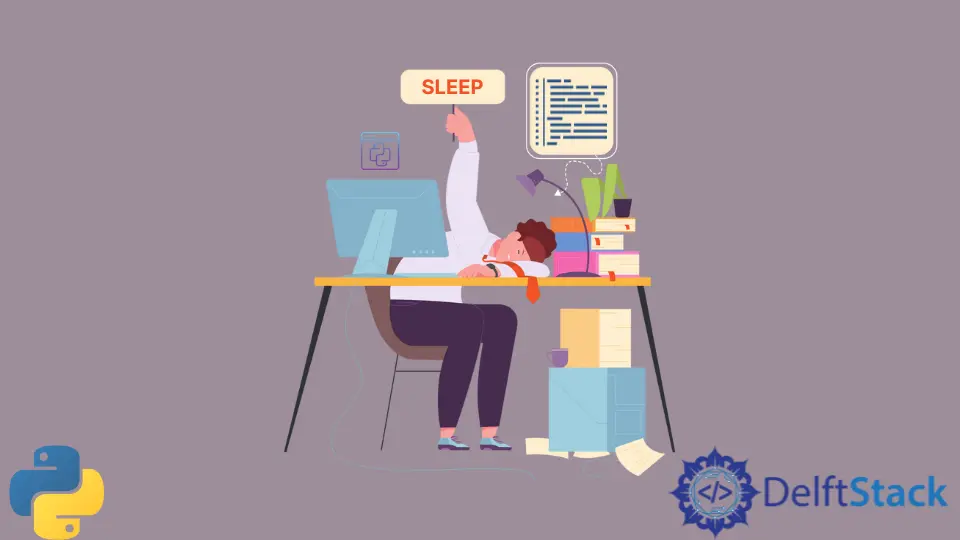
In the world of programming, there are times when you need your program to take a breather. Whether it’s to manage resource usage, create a delay between actions, or simply to synchronize tasks, pausing execution can be crucial. In Python, this is often achieved using the time
module, which provides a straightforward way to make your code sleep for a specified duration.
In this tutorial, we’ll explore how to pause your Python programs for milliseconds, allowing for precise control over execution timing. Whether you’re building a game, automating a task, or just trying to control the flow of your script, knowing how to sleep for milliseconds can enhance your coding toolkit.
Understanding the Time Module
Before diving into the actual code, it’s essential to understand the time
module, which is part of Python’s standard library. This module provides various time-related functions, and one of its most useful features is the ability to pause execution. The sleep()
function allows you to specify the duration of the pause in seconds. To achieve millisecond precision, you can simply use a decimal value.
Let’s look at how to implement this in Python.
Using the Sleep Function
The simplest method to pause execution in Python is by using the sleep()
function from the time
module. This function takes a single argument: the number of seconds to sleep. To sleep for milliseconds, you can provide a decimal value.
Here’s how you can do it:
import time
print("Sleeping for 0.5 seconds...")
time.sleep(0.5)
print("Awake now!")
Output:
Sleeping for 0.5 seconds...
Awake now!
In this example, we import the time
module and use time.sleep(0.5)
to pause the program for half a second. The sleep()
function is versatile, allowing you to specify any duration, including milliseconds. By passing 0.5
, we effectively tell the program to wait for 500 milliseconds. This is particularly useful in scenarios like animations or timed events where precise timing is crucial.
Implementing Millisecond Sleep in a Loop
Sometimes, you may want to pause execution within a loop, especially in applications like games or simulations where timing is essential. By using time.sleep()
in a loop, you can control how frequently certain actions occur.
Here’s an example:
import time
for i in range(5):
print(f"Iteration {i + 1}")
time.sleep(0.1) # Sleep for 100 milliseconds
Output:
Iteration 1
Iteration 2
Iteration 3
Iteration 4
Iteration 5
In this code snippet, we loop five times, printing the iteration number each time. The time.sleep(0.1)
call pauses execution for 100 milliseconds between iterations. This method is handy for creating delays in processes that need to run at specific intervals, such as updating a user interface or managing event timings.
Using Sleep with Asynchronous Programming
In modern Python applications, especially those involving I/O operations or web services, asynchronous programming is becoming increasingly popular. The asyncio
library allows for concurrent code execution, and you can also implement sleeping in an asynchronous context.
Here’s how you can use asyncio.sleep()
for millisecond pauses:
import asyncio
async def main():
print("Sleeping for 0.2 seconds...")
await asyncio.sleep(0.2)
print("Awake now!")
asyncio.run(main())
Output:
Sleeping for 0.2 seconds...
Awake now!
In this example, we define an asynchronous function main()
that uses await asyncio.sleep(0.2)
to pause for 200 milliseconds. This allows other tasks to run while waiting, making it efficient for applications that require non-blocking behavior. Using asyncio.sleep()
is particularly advantageous in scenarios involving multiple concurrent tasks, such as web scraping or handling multiple user requests.
Conclusion
In conclusion, knowing how to pause your Python programs for milliseconds can significantly enhance your coding capabilities. Whether you are using the traditional time.sleep()
method or exploring asynchronous programming with asyncio.sleep()
, these techniques provide flexibility and control over your program’s execution flow. Mastering these methods will not only improve the functionality of your applications but also make your code more efficient and responsive. So the next time you need to introduce a delay, remember these simple yet effective techniques.
FAQ
-
How precise is the sleep function in Python?
The precision of the sleep function can be affected by the operating system and system load, but it generally provides reliable timing for most applications. -
Can I use sleep in a multi-threaded environment?
Yes, you can use sleep in multi-threaded applications, but be cautious of how it interacts with thread scheduling. -
What is the difference between time.sleep and asyncio.sleep?
time.sleep
blocks the entire thread, whileasyncio.sleep
allows other tasks to run concurrently, making it suitable for asynchronous programming. -
Is there a maximum sleep duration in Python?
The maximum duration for sleep is limited by the underlying operating system, but in practice, you can sleep for very long durations. -
Can I use sleep with other libraries in Python?
Yes, sleep can be used in conjunction with various libraries, especially those that involve timing, animations, or user interactions.