clock() and time() Methods of the Time Module in Python
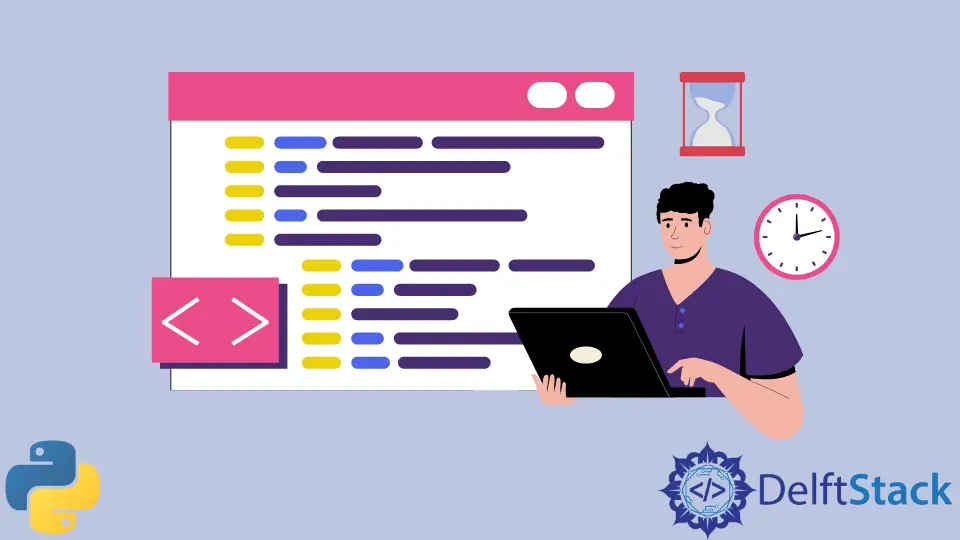
The time
module is a part of Python’s standard library.
This module contains time-related utilities. This module is used for various tasks such as calculating the execution time of a block of code, converting time from one unit to another such as hours to seconds and days to milliseconds, accessing the wall clock time.
The time
module has two methods, namely, clock()
and time()
, that are commonly used. This article will tackle the clock()
and time()
methods of Python’s time
module.
the clock()
and time()
Methods of the time
Module in Python
The clock()
method of the time
module returns the CPU time or real-time since the current process has started.
Unfortunately, this method is platform-dependent. This means that the clock()
method behaves differently for UNIX-based operating systems such as macOS, Linux, and Microsoft Windows.
For UNIX-based systems, this method returned the CPU time of the process as a floating-point and converted it in seconds. At the same time, Microsoft Windows returns the real-world time or the Wall Clock time elapsed in seconds since the first call to this method.
Because of this imbalance, the clock()
method was removed from the time
module in Python 3.8.
The time()
method returns the current time in seconds as a floating point. Unlike the clock()
method, the time()
method is platform-independent.
Let us know its usage with the help of an example. Refer to the following Python code for this.
import time
start = time.time()
time.sleep(5)
end = time.time()
print(end - start)
Output:
5.021177291870117
The Python code above first calls the time()
function and stores the timestamp in a variable.
Next, it sleeps or waits for 5
seconds using the sleep()
method. Then, it again calls the time()
function and stores the timestamp. Lastly, it prints the time difference to the console.
Note that for me, the time difference was 5.021177291870117
, which is very close to 5
. Keep in mind that little variations can be found in the calculations.
The sleeping action of 5
seconds is performed to represent a task that will take about 5
seconds to complete. It should be placed between the two time()
method calls to measure the execution time of a code block.
Refer to the following Python code for an example.
import time
start = time.time()
s = 0
for i in range(100000000):
s += i
print(s)
end = time.time()
print("Time Difference:", end - start)
Output:
4999999950000000
Time Difference: 14.171791315078735