How to Check if Directory Exists using Python
- Method 1: Using os.path.exists()
- Method 2: Using os.path.isdir()
- Method 3: Using pathlib
- Conclusion
- FAQ
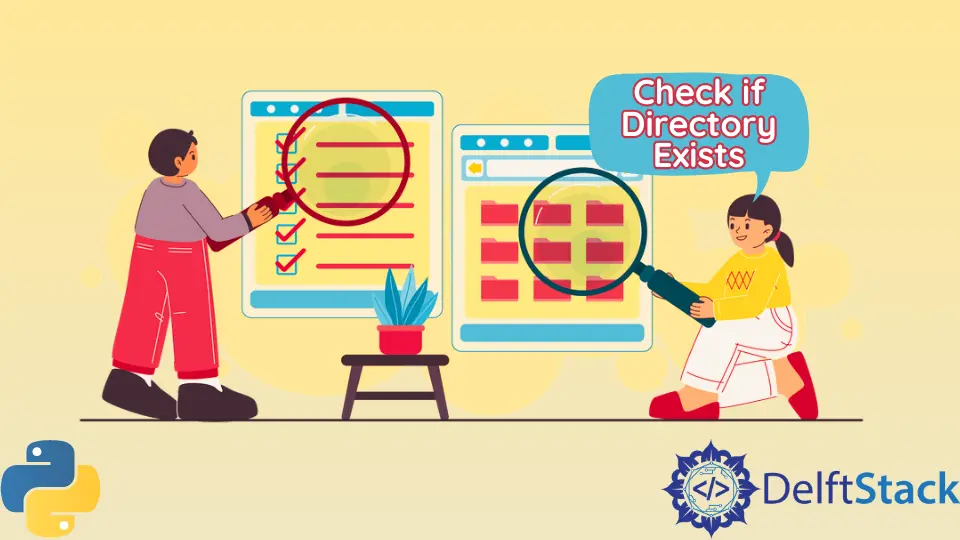
When working with file systems in Python, one of the common tasks you might encounter is checking whether a specific directory exists. This can be crucial for various applications, such as ensuring that your program has the necessary environment to run or preventing errors when trying to access files.
In this tutorial, we will explore different methods to check for the existence of a directory in Python. Whether you are a beginner or an experienced developer, understanding these techniques will enhance your coding skills and help you write more robust applications. Let’s dive into the various methods to check if a directory exists using Python.
Method 1: Using os.path.exists()
One of the simplest ways to check if a directory exists in Python is by using the os
module’s path.exists()
function. This method is straightforward and effective, making it a popular choice among developers. The os
module provides a portable way of using operating system-dependent functionality, and path.exists()
checks if a specified path exists, whether it’s a file or a directory.
Here’s how to use it:
import os
directory = "example_directory"
if os.path.exists(directory):
print(f"The directory '{directory}' exists.")
else:
print(f"The directory '{directory}' does not exist.")
Output:
The directory 'example_directory' does not exist.
In this code snippet, we first import the os
module. We then define the variable directory
, which holds the name of the directory we want to check. The os.path.exists(directory)
function checks if the specified path exists. If it does, we print a message confirming its existence; otherwise, we inform the user that the directory does not exist. This method is simple and works well for most use cases.
Method 2: Using os.path.isdir()
Another effective method to check if a directory exists is by using the os.path.isdir()
function. This function specifically checks whether the given path is a directory. Unlike os.path.exists()
, which returns True
for both files and directories, os.path.isdir()
will only return True
if the path points to a directory.
Here’s an example of how to use os.path.isdir()
:
import os
directory = "example_directory"
if os.path.isdir(directory):
print(f"The directory '{directory}' exists.")
else:
print(f"The directory '{directory}' does not exist.")
Output:
The directory 'example_directory' does not exist.
In this example, we again import the os
module and define our directory variable. The key difference here is that we use os.path.isdir(directory)
to check if the specified path is indeed a directory. If it is, we confirm its existence; if not, we indicate that it does not exist. This method is particularly useful when you want to differentiate between files and directories.
Method 3: Using pathlib
With the introduction of Python 3.4, the pathlib
module provides an object-oriented approach to handling filesystem paths. This module simplifies path manipulations and offers a more modern way to check for directory existence. The Path
class in pathlib
has a method called exists()
that can be used to check if a directory exists.
Here’s how you can use pathlib
to check for a directory:
from pathlib import Path
directory = Path("example_directory")
if directory.exists():
print(f"The directory '{directory}' exists.")
else:
print(f"The directory '{directory}' does not exist.")
Output:
The directory 'example_directory' does not exist.
In this snippet, we first import the Path
class from the pathlib
module. We create a Path
object for our directory. By calling directory.exists()
, we can easily check if the directory exists. This approach is more intuitive and readable, especially for those who prefer an object-oriented style. The pathlib
module is becoming increasingly popular among Python developers for its simplicity and elegance.
Conclusion
Checking whether a directory exists in Python is a fundamental skill that can save you from potential errors in your applications. In this tutorial, we explored three effective methods: using os.path.exists()
, os.path.isdir()
, and pathlib
. Each method has its advantages, and the choice often depends on your specific needs and coding style. By mastering these techniques, you can ensure that your Python applications handle file system interactions more gracefully. Happy coding!
FAQ
-
How do I create a directory in Python if it doesn’t exist?
You can useos.makedirs()
orPath.mkdir()
from thepathlib
module to create a directory if it doesn’t exist. -
Can I check for multiple directories at once?
Yes, you can loop through a list of directory names and apply any of the methods discussed to check each one. -
Which method is preferred for checking directory existence?
The choice often depends on personal preference, butpathlib
is generally favored for its modern and object-oriented approach. -
Does the existence check work for files as well?
Yes, bothos.path.exists()
andpathlib.Path.exists()
can check for both files and directories. -
What happens if the directory name contains special characters?
Python handles special characters in directory names, but ensure that the path is correctly formatted according to your operating system’s conventions.
Related Article - Python Directory
- How to Get Home Directory in Python
- How to List All Files in Directory and Subdirectories in Python
- How to Fix the No Such File in Directory Error in Python
- How to Get Directory From Path in Python
- How to Execute a Command on Each File in a Folder in Python
- How to Count the Number of Files in a Directory in Python