How to Add Days to a Date in Python
-
Use
datetime.timedelta()
to Add Days to a Date in Python -
Use
timedelta
indatetime
Module to Add Days to the Current Date - Use Pandas Module to Add Days to a Date in Python
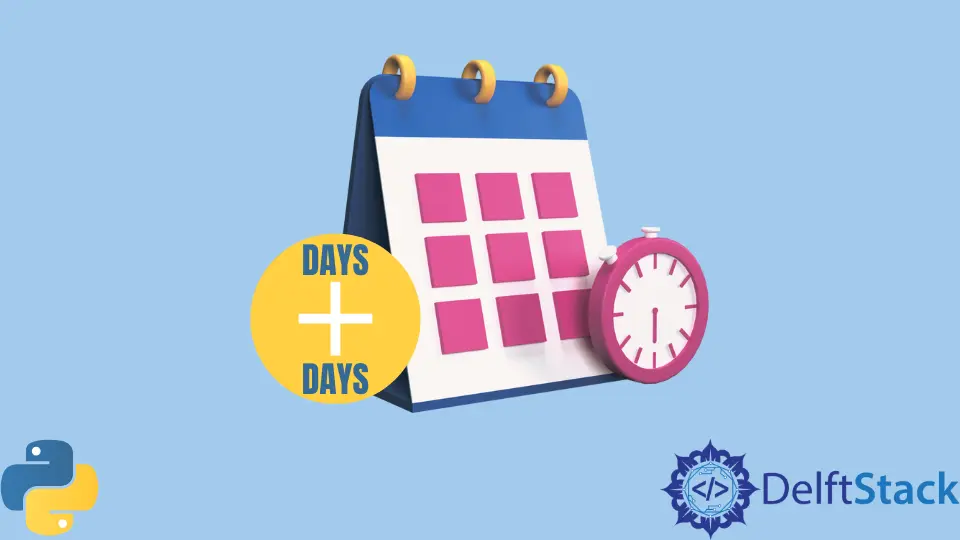
The tutorial explains how to add days to a date in Python.
Use datetime.timedelta()
to Add Days to a Date in Python
In Python, datetime
module provides a datetime.timedelta()
method. It is used for performing arithmetic operations on date and time values. It takes a number of days (and optionally, other time units such as hours, minutes, seconds, etc.) as its argument and returns a new date or time object.
The datetime.strptime()
method of datetime()
module takes the start date as its input and returns the same date in the format of datetime.datetime
.
It takes two arguments:
- The date-time string to be parsed.
- A format string that specifies the expected format of the input string.
An example code is given as:
import datetime
curr_date = "12/6/20"
curr_date_temp = datetime.datetime.strptime(curr_date, "%m/%d/%y")
new_date = curr_date_temp + datetime.timedelta(days=5)
print(new_date)
Output:
2020-12-11 00:00:00
Explanation
- Parsing the Current Date:
curr_date_temp = datetime.datetime.strptime(curr_date, "%m/%d/%y")
- Here,
datetime.datetime.strptime()
is used to parse thecurr_date
string according to the specified format ("%m/%d/%y"). - It creates a
datetime.datetime
object (curr_date_temp
) representing the same date.
- Calculating the New Date:
new_date = curr_date_temp + datetime.timedelta(days=5)
- The code adds 5 days to the
curr_date_temp
usingdatetime.timedelta(days=5)
. This creates a newdatetime.datetime
object (new_date
) that is 5 days ahead of the original date.
- Printing the New Date:
print(new_date)
- Finally, the code prints the
new_date
to the console.
When you run the code, it prints the new date in the format YYYY-MM-DD HH:MM:SS
. It is the default format.
This output represents the date and time 5 days after the original date, as calculated by adding a datetime.timedelta
of 5 days to the curr_date_temp
.
Use timedelta
in datetime
Module to Add Days to the Current Date
The Python datetime
has the timedelta
method itself besides the timedelta
from its submodule datetime
. The timedelta()
method takes the number of days to be added as its argument and returns them in date format. The date
module also has a today()
method, which returns the current date.
A basic example of this approach is given as:
from datetime import timedelta, date
Date_required = date.today() + timedelta(days=5)
print(Date_required)
Output:
2023-10-19
Explanation:
- Python’s
date
module provides thetoday()
method to get the current data.current_date = date.today()
Now, `current_date` holds the current date in the `datetime.date` format.
- Adding Days to the Current Date
With the current date in hand, we can use thetimedelta
class to add or subtract days, as well as other time units, to or from it. In this example, we’ll add 5 days to the current date:# Define the number of days to add days_to_add = 5 # Perform the date calculation Date_required = current_date + timedelta(days=days_to_add)
In this code snippet:
- We define `days_to_add` to specify the number of days we want to add to the current date. You can change this value to add a different number of days.
- We use the `+` operator to add the `timedelta` representing `days_to_add` to the `current_date`. This results in a new `datetime.date` object, `Date_required`, which is 5 days ahead of the current date.
Use datetime.now()
to Get the Current Date
You can also use dateime.now()
to get the current date and time as a datetime
object.
current_date = datetime.datetime.now()
The complete code to use dateime.now()
to add days to datetime is below.
from datetime import timedelta, datetime
Date_required = datetime.now() + timedelta(days=5)
print(Date_required)
Output:
2023-10-24 09:59:31.463865
Difference Between datetime.now()
and datetime.today()
Methods
In Python’s datetime
module, both datetime.now()
and datetime.today()
methods are used to obtain the current date and time. However, there is a subtle difference between them:
datetime.now()
:datetime.now()
returns the current date and time and It is more versatile as it allows you to pass a timezone object as an argument, giving you the current date and time in the specified timezone.- It includes information about the time zone offset from UTC (Coordinated Universal Time) if you pass the timezone object to it.
datetime.today()
:datetime.today()
also returns the current date and time, but does not allow you to specify a timezone. It is essentially equivalent to callingdatetime.now()
with no arguments.- It assumes that the current time is in the local time zone, but it does not provide details about the time zone itself.
Use Pandas Module to Add Days to a Date in Python
Pandas module provides a to_datetime()
method that takes a date as its argument and converts it into a pandas._libs.tslibs.timestamps.Timestamp
object. Pandas will do the smart converting if the format of the date string is not specified.
The to_datetime()
method in Pandas is a versatile function that allows you to convert date strings or objects into Pandas Timestamps, which are datetime objects optimized for efficient manipulation. This method can handle various date formats and performs smart conversion if the format is not explicitly specified.
The DateOffset()
method takes the keyword arguments like days
, months
and etc. It returns a pandas.tseries.offsets.DateOffset
object.
An example code is given below:
import pandas as pd
initial_date = "12/5/2019"
req_date = pd.to_datetime(initial_date) + pd.DateOffset(days=3)
print(req_date)
Output:
2019-12-08 00:00:00
In the code snippet above:
- We have an initial date string,
12/5/2019
, which we want to convert. - We use
pd.to_datetime(initial_date)
to convert the date string into a Pandas Timestamp. Pandas automatically detects the format of the input date string and converts it accordingly. - The resulting
req_date
variable holds a Pandas Timestamp object representing the converted date.
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn