Python Datetime.timedelta() Method
-
Syntax of Python
datetime.timedelta()
Method -
Example Codes: Working With the
datetime.timedelta()
Method -
Example Codes: Find Time Difference Using the
datetime.timedelta()
Method -
Example Codes: Enter Negative Values in the
datetime.timedelta()
Method -
Example Codes: Add Days and Time in a DateTime Object Using the
datetime.timedelta()
Method
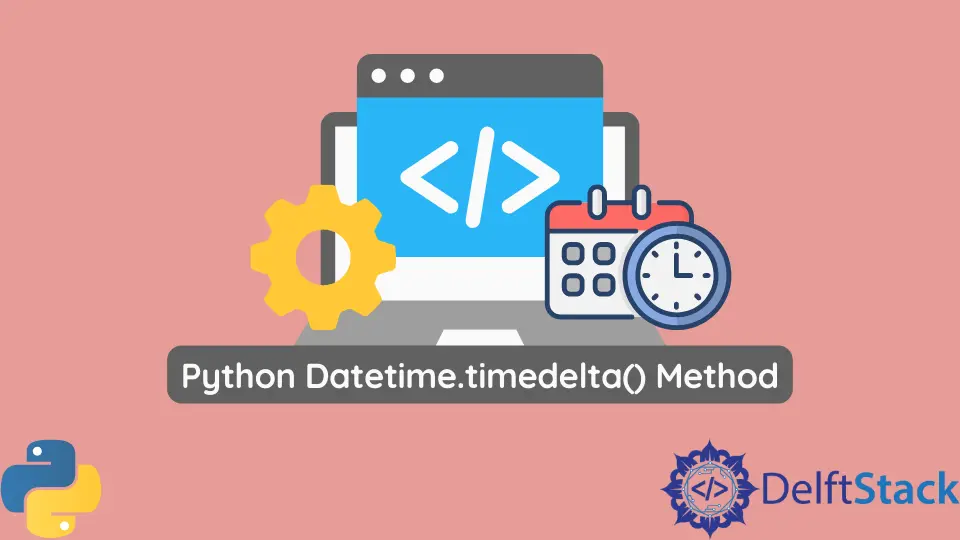
Python datetime.timedelta()
method is an efficient way of calculating the duration between two dates and times. A timedelta
object can do mathematical operations, e.g., addition, subtraction, multiplication, division, etc.
Syntax of Python datetime.timedelta()
Method
datetime.timedelta()
datetime.timedelta(days, seconds, microseconds, milliseconds, minutes, hours, weeks)
Parameters
days |
Optional argument, default value is 0. |
seconds |
Optional argument, default value is 0. |
microseconds |
Optional argument, default value is 0. |
milliseconds |
Optional argument, default value is 0. A millisecond is always converted to 1000 microseconds. |
minutes |
Optional argument, default value is 0. A minute is always converted to 60 seconds. |
hours |
Optional argument, default value is 0. An hour is always converted to 3600 seconds. |
weeks |
Optional argument, default value is 0. A week is always converted to 7 days. |
Return
The return type of this method is a timedelta
object.
Example Codes: Working With the datetime.timedelta()
Method
import datetime
print(
datetime.timedelta(
days=20,
seconds=40,
microseconds=8,
milliseconds=9,
minutes=30,
hours=7,
weeks=6,
)
)
Output:
62 days, 7:30:40.009008
Note that the arguments can be in integers or floats. The values may be either positive or negative.
Example Codes: Find Time Difference Using the datetime.timedelta()
Method
import datetime
print("Today is ", datetime.datetime.now())
print(
"One year from now, the date will be "
+ str(datetime.datetime.now() + datetime.timedelta(days=365))
)
Output:
Today is 2022-09-05 11:20:24.346875
One year from now, the date will be 2023-09-05 11:20:24.346932
An OverflowError
or OSError
exception may occur if argument values lie outside the indicated range.
Example Codes: Enter Negative Values in the datetime.timedelta()
Method
import datetime
time = datetime.datetime.now()
print(time + datetime.timedelta(days=-3))
Output:
2022-09-02 11:25:02.151686
The above code can easily be used to find a date and time in the past.
Example Codes: Add Days and Time in a DateTime Object Using the datetime.timedelta()
Method
import datetime
time = datetime.datetime.now()
print(time)
print(time + datetime.timedelta(days=20, seconds=40, milliseconds=9, hours=7, weeks=6))
Output:
2022-09-05 11:28:26.239365
2022-11-06 18:29:06.248365
The above code can easily be used to find a date and time in the future.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn