How to Calculate Cube Root in Python
-
Python Get Cube Root Using the Exponent Symbol
**
-
Python Get Cube Root Using the
pow()
Function -
Python Get Cube Root Using the
cbrt()
Function of the NumPy Library -
Python Get Cube Root Using the
math.sqrt
Function - Python Get Cube Root Using Binary Search
- Python Get Cube Root Using Exponentiation Operator and Logarithm
-
Python Get Cube Root Using the
reduce()
Function - Conclusion
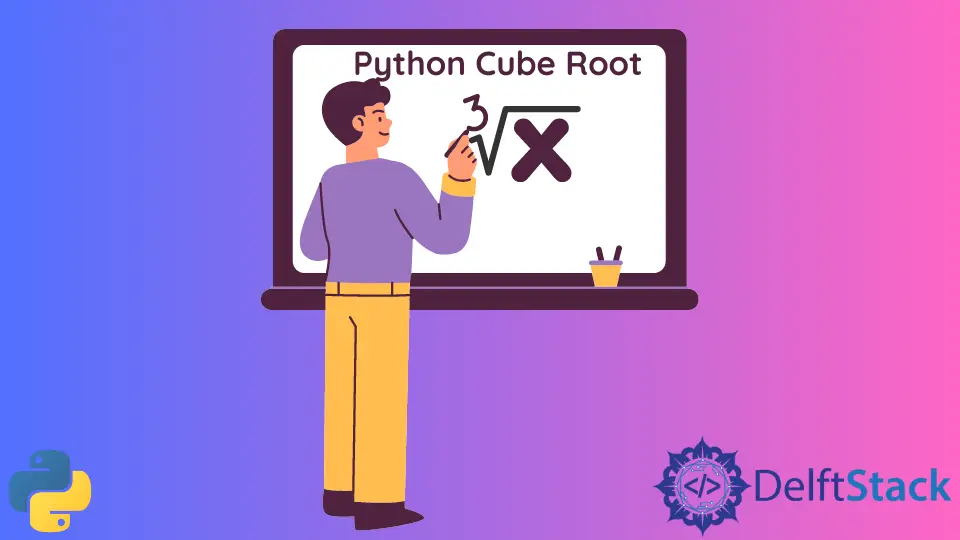
Calculating the cube root of an integer or a float variable in Python can be achieved through various methods, each with its unique approach and advantages.
In this tutorial, we will explore multiple techniques, including using the exponent symbol (**
), the pow()
function, the cbrt()
function from the NumPy library, the math.sqrt
function, binary search, exponentiation with logarithms, and the reduce()
function.
Python Get Cube Root Using the Exponent Symbol **
One straightforward approach to calculate the cube root of an integer or a float variable in Python is by using the exponent symbol **
.
The exponent symbol **
is an operator that raises a number to a specified power. To compute the cube root, we can leverage this symbol by setting the power to 1/3
.
The syntax is as follows:
result = number ** (1 / 3)
Here, number
is the integer or float variable for which we want to find the cube root. The expression 1/3
indicates the cube root operation.
It’s worth noting that using this method may lead to incorrect results for negative numbers. For instance, the cube root of -27
should be -3
, but Python might return a complex number.
To address this, we can simply ignore the negative sign before calculating the cube root.
Let’s implement the cube root calculation using the exponent symbol in Python. The following code demonstrates this method:
def get_cuberoot(x):
if x < 0:
x = abs(x)
cube_root = x ** (1 / 3) * (-1)
else:
cube_root = x ** (1 / 3)
return cube_root
print(get_cuberoot(64))
print(round(get_cuberoot(64)))
print(get_cuberoot(-27))
In the code, we define a function get_cuberoot(x)
that takes a number x
as its parameter. Inside the function, we check if the input x
is negative.
If so, we take the absolute value (abs(x)
) to eliminate the negative sign, calculate the cube root using x ** (1/3)
, and then multiply the result by -1
to reintroduce the negative sign.
For positive numbers, the cube root is computed directly. The function returns the final cube root value.
The provided test cases showcase the function’s usage, with outputs for both positive and negative numbers.
After executing the above code, the output will be:
3.9999999999999996
4
-3.0
Python Get Cube Root Using the pow()
Function
An alternative method to compute the cube root of an integer or a float variable involves using the pow()
function. This versatile function takes a number as its first argument and the exponent or power as its second argument, returning the result of raising the number to the specified power.
The pow()
function has a straightforward syntax, taking the form:
result = pow(number, 1 / 3)
Here, number
represents the integer or float variable for which we want to find the cube root, and 1/3
signifies the cube root operation. Unlike the previous method, the pow()
function simplifies the code by directly accepting the exponent as its second argument.
However, similar to the exponent symbol method, the pow()
function may yield incorrect results for negative numbers. To address this, we can handle negative numbers separately by taking the absolute value and adjusting the sign.
Let’s implement the cube root calculation using the pow()
function in Python. The following code demonstrates this method:
def get_cuberoot(x):
if x < 0:
x = abs(x)
cube_root = pow(x, 1 / 3) * (-1)
else:
cube_root = pow(x, 1 / 3)
return cube_root
print(get_cuberoot(64))
print(round(get_cuberoot(64)))
print(get_cuberoot(-27))
In the code, we define a function get_cuberoot(x)
that takes a number x
as its parameter. Within the function, we check if the input x
is negative.
If so, we take the absolute value (abs(x)
) to eliminate the negative sign, calculate the cube root using pow(x, 1/3)
, and then multiply the result by -1
to reintroduce the negative sign.
For positive numbers, the cube root is computed directly using pow(x, 1/3)
. The function returns the final cube root value.
The provided test cases showcase the function’s usage, offering outputs for both positive and negative numbers.
After executing the above code, the output will be:
3.9999999999999996
4
-3.0
As seen in the output, the pow()
function behaves similarly to the exponent symbol method, accurately calculating the cube root for positive numbers while returning complex numbers for negative ones. Rounding the result may be necessary to address this discrepancy.
Python Get Cube Root Using the cbrt()
Function of the NumPy Library
For a seamless and accurate method to calculate the cube root of an integer or a float variable in Python, we can use the cbrt()
function from the NumPy library. NumPy is a powerful library for numerical computing, and its cbrt()
function simplifies cube root calculations without the complexities encountered in some native Python methods.
The syntax is straightforward:
result = np.cbrt(number)
Here, number
is the integer or float variable for which we want to find the cube root. Unlike the previous methods, the cbrt()
function handles negative numbers seamlessly, providing accurate results without requiring special handling.
It’s important to note that you need to have NumPy installed to use the cbrt()
function. You can install it using:
pip install numpy
Let’s implement the cube root calculation using the cbrt()
function from the NumPy library in Python. The following code demonstrates this method:
import numpy as np
print(np.cbrt(64))
print(np.cbrt(-64))
print(np.cbrt(9.4))
In this code, we start by importing the NumPy library using import numpy as np
. The test cases then showcase the simplicity of using the cbrt()
function.
For positive numbers like 64
and 9.4
, the function accurately calculates the cube root. Remarkably, when dealing with negative numbers such as -27
, the cbrt()
function handles them seamlessly, providing precise results without any additional code to address negativity.
After executing the above code, the output will be:
4.0
-4.0
2.11045429449015
As seen in the output, the cbrt()
function delivers accurate cube root results for both positive and negative numbers, making it a convenient and reliable choice for such calculations in Python.
Python Get Cube Root Using the math.sqrt
Function
In Python, the math.sqrt
function, traditionally used for square roots, can also be used to calculate cube roots by raising a number to the power of 1/3
. While not as intuitive as some other methods, this approach provides an alternative for those who prefer using the math
module.
The syntax is as follows:
import math
result = math.pow(number, 1 / 3)
Here, number
represents the integer or float variable for which we want to find the cube root. The math.pow
function is employed to calculate the desired power, and the result is the cube root of the given number.
Let’s implement the cube root calculation using the math.sqrt
function in Python. The following code demonstrates this method:
import math
def get_cuberoot(x):
cube_root = math.pow(x, 1 / 3)
return cube_root
print(get_cuberoot(64))
print(get_cuberoot(27))
print(get_cuberoot(9.4))
In this code, we begin by importing the math
module using import math
. The function get_cuberoot(x)
is then defined to calculate the cube root of a given number.
Inside the function, the math.pow
function is used to raise x
to the power of 1/3
, resulting in the cube root. The function returns this cube root value.
The test cases showcase the usage of the get_cuberoot
function for various numbers, including positive and negative integers, as well as a float.
After executing the above code, the output will be:
3.9999999999999996
3.0
2.11045429449015
As seen in the output, the math.sqrt
function, when used with the appropriate power, accurately calculates cube roots.
However, similar to other methods, it returns complex numbers for negative inputs. Rounding may be necessary for precise results in certain cases.
Python Get Cube Root Using Binary Search
We can employ a more unconventional yet efficient method to calculate the cube root of an integer or a float variable using binary search. While not as straightforward as some built-in functions, binary search allows us to approximate the cube root with precision.
Binary search involves iteratively narrowing down a range of values until the desired result is reached. To use binary search for cube root calculation, we can define a range based on the input number and iteratively adjust it until the result is within an acceptable tolerance.
Let’s implement the cube root calculation using binary search in Python. The following code demonstrates this method:
def get_cuberoot(x, epsilon=1e-6):
low, high = 0, abs(x)
guess = (low + high) / 2
while abs(guess**3 - abs(x)) > epsilon:
if guess**3 < abs(x):
low = guess
else:
high = guess
guess = (low + high) / 2
return guess if x > 0 else -guess
print(get_cuberoot(64))
print(get_cuberoot(-27))
print(get_cuberoot(9.4))
In this code, we define a function get_cuberoot(x, epsilon=1e-6)
that takes a number x
and an optional tolerance epsilon
as parameters. We initialize the range (low
and high
) based on the absolute value of the input.
The guess
is set initially to the midpoint of the range. The while
loop continues until the cube of the guess is within the specified tolerance of the absolute value of x
.
Within the loop, we adjust the range based on whether the cube of the guess is less or greater than the absolute value of x
. The final result is returned with the appropriate sign for negative numbers.
After executing the above code, the output will be:
3.999999761581421
-3.000000238418579
2.1104540824890137
The binary search method accurately approximates the cube root for both positive and negative numbers. The result may not be as precise as some other methods, but it provides a viable option, especially when simplicity and efficiency are priorities.
Python Get Cube Root Using Exponentiation Operator and Logarithm
An alternative method to calculate the cube root of an integer or a float variable involves using the exponentiation operation in conjunction with logarithmic functions. By applying the math.exp(math.log(n)/3)
formula, we can efficiently obtain the cube root of a number.
The math.exp(math.log(n)/3)
formula uses the properties of logarithms and exponentiation to find the cube root.
The math.log(n)
function calculates the natural logarithm of the number, and then dividing it by 3
represents the cube root, and math.exp
raises the result to the power of e
, effectively computing the cube root.
Let’s implement the cube root calculation using the exponentiation operator and logarithm in Python.
import math
def get_cuberoot(x):
return math.exp(math.log(x) / 3)
print(round(get_cuberoot(64)))
print(round(get_cuberoot(27)))
In this code, we start by importing the math
module using import math
. The function get_cuberoot(x)
takes a number x
as its parameter and returns the cube root.
In the function, we use math.log(x)
to calculate the natural logarithm of the number and then divide it by 3
, which represents the cube root. The result is then obtained by applying math.exp
to the logarithmic value.
After executing the above code, the output will be:
4
3
As seen in the output, the method accurately calculates the cube root for positive numbers.
However, note that this approach returns a complex number for negative numbers, similar to the behavior observed in previous methods. Also, rounding the result may be necessary for precise results.
Python Get Cube Root Using the reduce()
Function
In Python, we can also use the reduce()
function from the functools
module to calculate the cube root of an integer or a float variable. While reduce()
is typically associated with iterables and cumulative operations, it can be creatively utilized for cube root approximation.
The reduce()
function is often used for cumulative operations on iterables. To adapt it for cube root calculation, we need to define a function that represents the iterative process.
This function should take two arguments, combine them, and then the result is used in the next iteration. By defining a suitable function, we can leverage reduce()
to approximate the cube root.
Let’s implement the cube root calculation using reduce()
in Python.
from functools import reduce
def cube_root_approximation(x, iterations=100, epsilon=1e-6):
approximations = [x] * iterations
def update_approximation(current, _):
return (2 * current + x / current**2) / 3
result = reduce(update_approximation, approximations, x)
return result if x > 0 else -result
print(cube_root_approximation(64))
print(cube_root_approximation(-27))
print(cube_root_approximation(9.4))
In this code, we first import the reduce()
function from the functools
module using from functools import reduce
.
Here, the main function takes a number, x
, and optional parameters for the number of iterations and tolerance.
cube_root_approximation(x, iterations=100, epsilon=1e-6)
This function initializes a list of approximations with the input value x
. The update_approximation
function defines the iterative process for the reduce()
function, which updates the approximation using the formula (2 * current + x / current**2) / 3
.
The final result is returned with the appropriate sign for negative numbers.
After executing the above code, the output will be:
4.0
3.0
2.11045429449015
As seen in the output, the reduce()
function, when creatively utilized for cube root approximation, accurately calculates the cube root for both positive and negative numbers. While this method may not be as intuitive as some others, it showcases the versatility of Python’s functional programming features.
Conclusion
In this article, we’ve explored multiple methods for calculating the cube root in Python. The choice of method depends on factors such as simplicity, accuracy, and efficiency.
Whether using built-in operators, library functions, or creative mathematical approaches, these methods showcase the versatility of Python for numerical computations. Consider the characteristics of your data and the desired precision when selecting the appropriate method for your specific use case.