How to Convert Two Lists Into Dictionary in Python
-
Use
zip()
anddict()
to Convert Two Lists to Dictionary in Python - Use Dictionary Comprehension to Convert List to Dictionary in Python
-
Use the
for
Loop to Convert List to Dictionary in Python
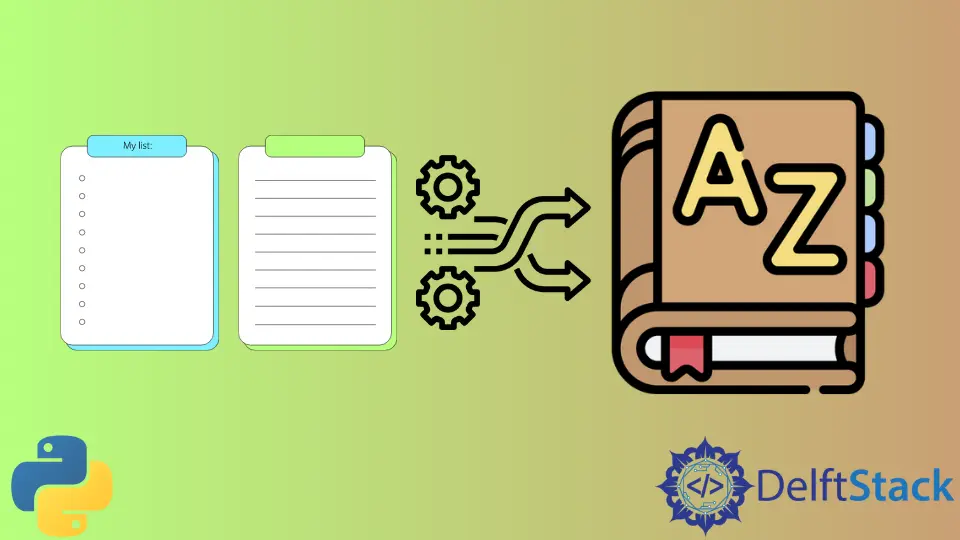
This tutorial will introduce how to convert two lists into a dictionary in Python wherein one list contains the dictionary’s keys, and the other contains the values.
Use zip()
and dict()
to Convert Two Lists to Dictionary in Python
Python has a built-in function called zip()
, which aggregates iterable data types into a tuple and returns it to the caller. The function dict()
creates a dictionary out of the given collection.
key_list = ["name", "age", "address"]
value_list = ["Johnny", "27", "New York"]
dict_from_list = dict(zip(key_list, value_list))
print(dict_from_list)
In this solution, converting lists to dictionaries is possible by using just a single line and two functions.
Output:
{'name': 'Johnny', 'age': '27', 'address': 'New York'}
Use Dictionary Comprehension to Convert List to Dictionary in Python
Dictionary comprehension is a way to shorten the initialization time of creating dictionaries in Python. Comprehension is a replacement of for loops, which requires multiple lines and variables. Comprehension does the job of initialization in a single line.
In this solution, the function zip()
will still provide values into the dictionary comprehension. The comprehension will return a key-value pair of each consecutive element within the return value of the function zip()
as a dictionary.
key_list = ["name", "age", "address"]
value_list = ["Johnny", "27", "New York"]
dict_from_list = {k: v for k, v in zip(key_list, value_list)}
print(dict_from_list)
Another way of converting lists to a dictionary is by utilizing the lists’ indices and using the range()
function to iterate over both lists and use comprehension to build a dictionary out of it.
It assumes that the length of the key list and the value list is the same. In this example, the key list length will be the basis for the range.
key_list = ["name", "age", "address"]
value_list = ["Johnny", "27", "New York"]
dict_from_list = {key_list[i]: value_list[i] for i in range(len(key_list))}
print(dict_from_list)
Both of these comprehension solutions will provide the same output as the previous example.
The only difference between these two solutions is that one uses the zip()
function to convert the list into a tuple. The other uses the range()
function to iterate both lists using the current index to form a dictionary.
Output:
{'name': 'Johnny', 'age': '27', 'address': 'New York'}
Use the for
Loop to Convert List to Dictionary in Python
The most straightforward way of achieving list to dictionary conversion is by looping it. Although this method is the most inefficient and lengthy, it is a good start, especially if you are a beginner and want to comprehend more about basic programming syntax.
Before looping, initialize an empty dictionary. Afterward, proceed to the conversion, which will require a nested loop; the outer loop will iterate the key list, while the inner loop iterates the value list.
key_list = ["name", "age", "address"]
value_list = ["Johnny", "27", "New York"]
dict_from_list = {}
for key in key_list:
for value in value_list:
dict_from_list[key] = value
value_list.remove(value)
break
print(dict_from_list)
Each time a key-value pair is formed, it removes the value element from the list so that the next key will be assigned the next value. After removal, break the inner loop to proceed to the next key.
Another solution using a loop is to use range()
to utilize the index to get the key and value pairs, just like what was done in the example with dictionary comprehension.
key_list = ["name", "age", "address"]
value_list = ["Johnny", "27", "New York"]
dict_from_list = {}
for i in range(len(key_list)):
dict_from_list[key_list[i]] = value_list[i]
print(dict_from_list)
Both solutions will produce the same output:
{'name': 'Johnny', 'age': '27', 'address': 'New York'}
In summary, the most efficient way to convert two lists into a dictionary is to use the built-in function zip()
to convert the two lists into a tuple and then convert the tuple into a dictionary using dict()
.
Using dictionary comprehension can also convert lists into a dictionary using either zip()
to convert the lists into tuple, or range()
and use the lists’ index to iterate and initialize a dictionary.
These two solutions are better solutions than the naive solution, which uses plain loops to convert the lists into a dictionary.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python