How to Convert Epoch to Datetime in Python
-
Use the
time
Module to Convert Epoch to Datetime in Python -
Use the
datetime
Module to Convert Epoch to Datetime in Python
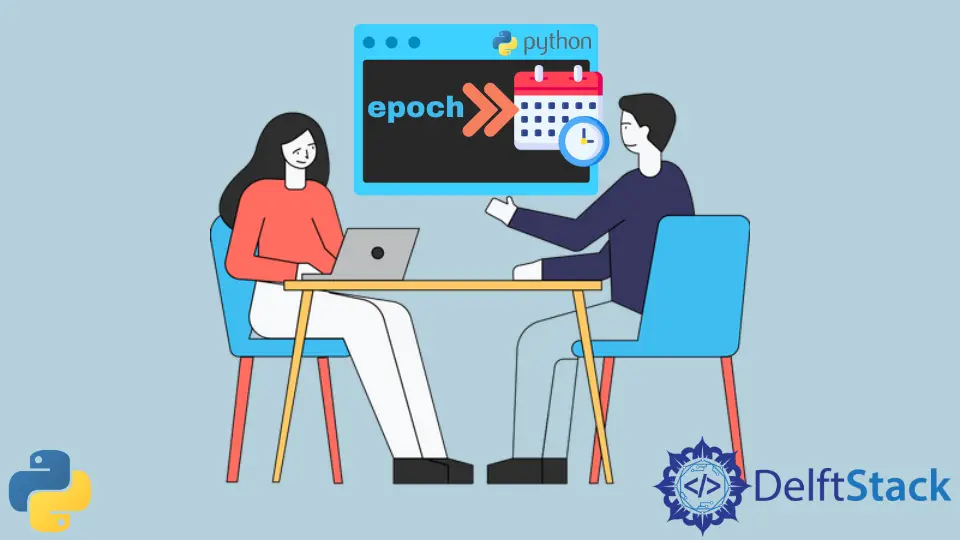
This tutorial will introduce how to convert epoch seconds to the datetime format in Python.
Epoch is a term for the number of seconds that have elapsed since the epoch date - January 1, 1970. The year 1970 is the release date of UNIX OS. Epoch time is also alternatively called as UNIX timestamp.
For example, the epoch for the date November 25, 2020 (00:00:00) would be 1606262400
, which is the number of seconds that have elapsed since 1970/01/01 until that date.
Use the time
Module to Convert Epoch to Datetime in Python
The Python time
module provides convenient time-related functions. The function provides a lot of support for computations related to UNIX timestamps.
For instance, the function time.localtime()
accepts epoch seconds as an argument and generates a time value sequence consisting of 9 time sequences.
Let’s use a random epoch time for this example.
import time
epoch_time = 1554723620
time_val = time.localtime(epoch_time)
print("Date in time_struct:", time_val)
Output:
Date in time_struct: time.struct_time(tm_year=2019, tm_mon=4, tm_mday=8, tm_hour=19, tm_min=40, tm_sec=20, tm_wday=0, tm_yday=98, tm_isdst=0)
localtime()
outputs a tuple struct_time()
with the details of the epoch time containing the exact year, month, day, and other datetime details that the epoch time contains.
The time
module has another function time.strftime()
to convert the tuple into a datetime format.
The function accepts 2 arguments, the first argument is a string containing the specified format for conversion, and the second argument is the struct_time()
tuple.
Now, convert the same epoch time example above into a readable datetime format.
import time
epoch_time = 1554723620
time_formatted = time.strftime("%Y-%m-%d %H:%M:%S", time.localtime(epoch_time))
print("Formatted Date:", time_formatted)
Output:
Formatted Date: 2019-04-08 19:40:20
Apart from this format, another generic date format would be the full name of the month and including the day of the week. Let’s try that out.
import time
epoch_time = 1554723620
time_formatted = time.strftime("%A, %B %-d, %Y %H:%M:%S", time.localtime(epoch_time))
print("Formatted Date:", time_formatted)
Formatted Date: Monday, April 8, 2019 19:40:20
Another useful format in strftime()
that only needs a single character is %c
, which is the generic date and time representation for Locale.
time_formatted = time.strftime("%c", time.localtime(epoch_time))
print("Formatted Date:", time_formatted)
Output:
Formatted Date: Mon Apr 8 19:40:20 2019
Use the datetime
Module to Convert Epoch to Datetime in Python
datetime
is another module in Python that supports time-related functions. The difference is the time
module focuses on Unix timestamps while datetime
supports a wide range of date and time functions while still supporting many of the operations that the time
module has.
datetime
has the function fromtimestamp()
which accepts epoch seconds and a datetime
object.
Let’s try out a different epoch second example for this module.
datetime
is intentionally repeated twice. Within the datetime
module is a datetime
object with the function fromtimestamp()
. date
and time
are also objects within the datetime
module.import datetime
epoch_time = 561219765
time_val = datetime.datetime.fromtimestamp(epoch_time)
print("Date:", time_val)
If datetime.datetime
is exclusively the object that’s being used within the datetime
module, then import it from the module to avoid the code looking redundant.
from datetime import datetime
Output:
Date: 1987-10-14 22:22:45
To convert the datetime into another format, datetime()
also has the utility function strftime()
which provides the same functionality as time.strftime()
.
import datetime
epoch_time = 561219765
time_formatted = datetime.datetime.fromtimestamp(epoch_time).strftime(
"%A, %B %-d, %Y %H:%M:%S"
)
print("Formatted Date:", time_formatted)
Output:
Formatted Date: Wednesday, October 14, 1987 22:22:45
In summary, these are the two main ways to convert epoch to datetime format in Python. Both the time
and datetime
modules provide functions that can be conveniently used for datetime conversion.
Both modules have the function strftime()
, which is a convenient way to format dates.
Here’s a reference to get familiar with datetime formats in Python to be able to customize date formatting using strftime()
.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn