How to Comment a Block of Code in Python
-
Add
#
Before Each Line to Comment Out Multiple Lines in Python - Use Triple Quotes for Multiline Comments in Python
-
Use
if False:
for Multiline Comments in Python - Use a String Variable for Multiline Comments in Python
- Use a Code Editor for Multiline Comments in Python
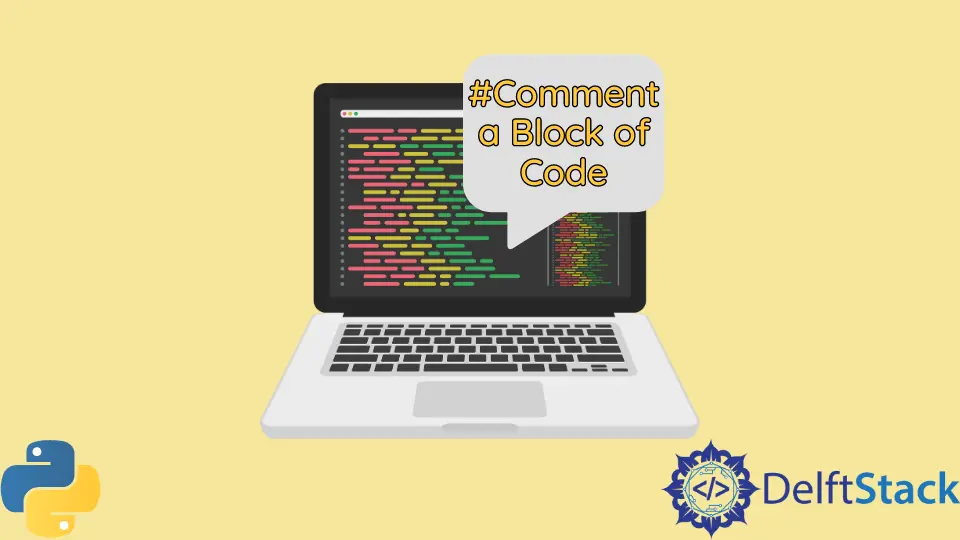
In this tutorial, we will discuss methods to comment out multiple lines of code in Python.
Add #
Before Each Line to Comment Out Multiple Lines in Python
The #
is used for single-line comments in Python. Unfortunately, there is no default method of commenting out multiple lines of code in Python. To comment out multiple code lines using the #
, we have to add a #
before each line. The following code example shows us how we can use #
to comment out multiple code lines in Python.
# Hello! this is a
# Multiple line comment
# print("This is a comment")
print("This is not a comment")
Output:
This is not a comment
In the above code, we commented out the first 3 lines of code by using #
before each line. This approach is ok for fewer lines, but this process can become very labor extensive if we have a huge number of lines.
Use Triple Quotes for Multiline Comments in Python
The Triple Quotes are used for code documentation and do not get executed during the execution of code. The following code example shows us how to use triple quotes to comment out multiple code lines in Python.
"""
Hello! this is a
Multiple line comment
print("This is a comment")
"""
print("This is not a comment")
Output:
This is not a comment
In the above code, we commented out the first 3 lines of code by putting them in triple quotes. The triple quotes reduce the effort drastically. But, triple quotes are designed to be used for code documentation and should not be used for commenting out code.
Use if False:
for Multiline Comments in Python
Another approach that can be used for commenting out multiple lines of code in Python is if False:
statement. Everything written in this block of code never gets executed because it is never False
in this context. We just have to write if False:
before the block of code we want to comment and then indent our code inside the if
statement. The following code example shows us how we can use if False:
to comment out multiple code lines in Python.
if False:
print("This is a comment")
print("This is another comment")
print("This is not a comment")
Output:
This is not a comment
In the above code, we commented out 2 lines of code using if False:
. The only problem with this approach is that it can only comment out a code block with proper syntax. The interpreter will still detect syntax errors in this block of code.
Use a String Variable for Multiline Comments in Python
A string variable can be used to store multiple lines of code in it. We can use triple quotes to store multiple lines of code in a string variable. The following code example shows us how we can use a string variable to comment out multiple code lines in Python.
comments = "This is a variable for commenting"
comments = """Hello! this is a
Multiple line comment
print("This is a comment")"""
print("This is not a comment")
Output:
This is not a comment
The same variable can be used multiple times to comment multiple blocks of code in Python.
Use a Code Editor for Multiline Comments in Python
We have discussed many approaches that can be used to comment out multiple lines of code in Python. But, unfortunately, none of the above approaches is an optimal solution. The best solution for this task would be to use the keyboard shortcuts of your desired code editor to comment out multiple lines of code in Python.
Visual Studio Code
In Visual Studio Code, select the block of code and use Ctrl+k, Ctrl+c to comment and Ctrl+k, Ctrl+u to uncomment.
Notepad++
In Notepad++, select the block of code and use Ctrl+k to comment.
PyCharm
In Pycharm IDE, select the block of code and use Ctrl+/ to comment and uncomment.
No matter which code editor you are using, it has a way to comment out multiple lines of code. All you have to do is search for the keyboard shortcut for commenting out multiple lines.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn