Double Underscore in Python
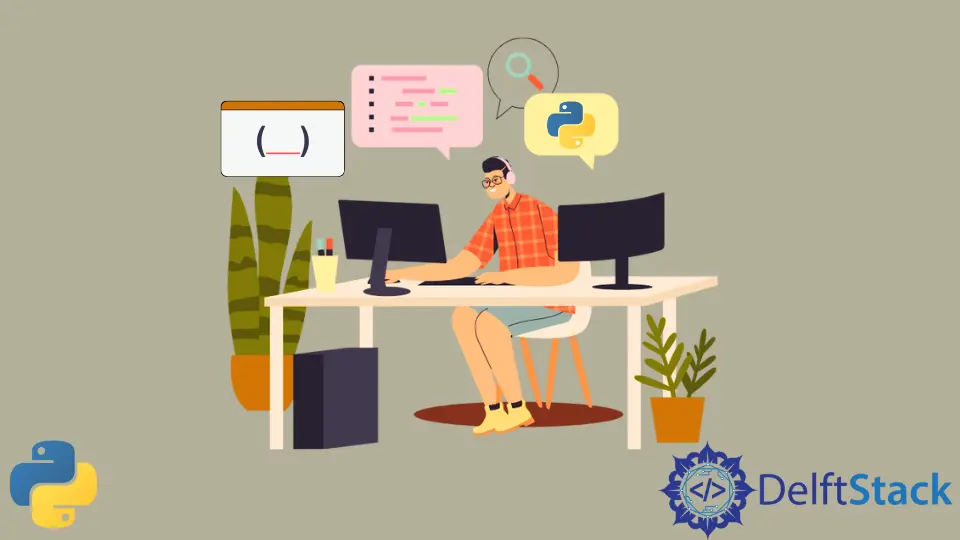
Both the underscore (_
) and the double underscore (__
) hold great significance in the world of Python programming and are excessively used by programmers for different purposes. Double underscores are pretty handy and are frequently encountered in Python code.
In this article, we will discuss the meaning of the double underscore in Python.
Use the Double-Leading Underscore in Python
When the double underscore leads a given identifier (__var
), the name mangling process occurs on that particular identifier.
Name mangling, in simple terms, is to basically rewrite the attribute name in order to prevent naming conflicts with the subclasses.
You can use the following code to explain the double leading underscore in Python.
class E1:
def __init__(self):
self.bar = 11
self._baz = 23
self.__foo = 23
x = E1()
print(dir(x))
Output:
['_E1__foo', '__doc__', '__init__', '__module__', '_baz', 'bar']
Explanation
- In the code above, we take a class and compare the single underscore, double underscore, and normal elements.
- The elements
foo
,bar
,baz
are simple keywords used as a placeholder here for the values that happen to change on conditions passed to the program or new information received by the program. - The
dir()
function is used here to provide a list of valid attributes of the given object passed as an argument in the function.
In the code above, we notice that the bar
and _baz
variable looks to be unchanged, while the __foo
variable has been changed to _E1__foo
. This is the process of name tangling occurring on the variable, and it is done to safeguard the variable against getting overridden in subclasses.
This process of name mangling of the variables with leading double underscore is totally transparent to the programmer. Name mangling interacts with and changes everything that leads with a double underscore, including functions.
Use Double Leading and Trailing Underscore in Python
When a variable is surrounded by double underscore on both the leading and trailing sides, the process of name mangling is not applied to it.
Variables clustered by the double underscore as both the prefix and the postfix are ignored by the Python interpreter.
You can use the following code to explain the double leading and trailing underscore in Python.
class A:
def __init__(self):
self.__foo__ = 10
print(A().__foo__)
Output:
10
In the code above, the foo
variable with a double underscore as prefix and postfix is ignored by the interpreter, and the name mangling does not occur. The value of this function is returned as the output, which proves that the variable exists in the object attribute list.
Some unique names, like init
or call
that contains both the leading and the trailing double underscore, are reserved in the Python language for special use.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn