Inline if...else Statement in Python
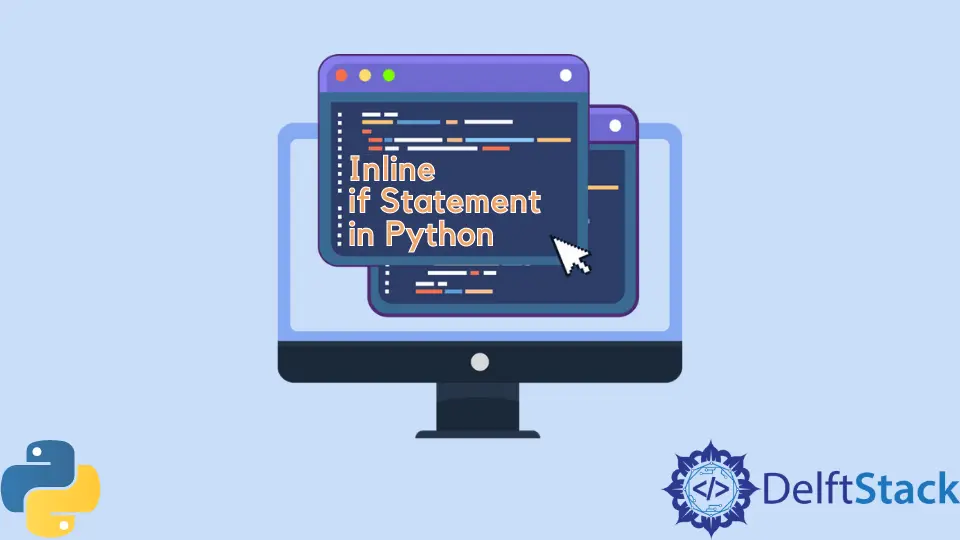
In Python, the inline if…else statement, also known as the conditional expression, is a powerful tool that allows developers to write concise and readable code. This feature enables you to evaluate a condition and return one of two values based on the truthiness of that condition—all in a single line. Not only does this enhance code readability, but it also streamlines your coding process, making it easier to manage complex logic.
In this tutorial, we will dive into the inline if…else statement, explore its syntax, and provide practical examples to illustrate its use. Whether you’re a beginner or an experienced programmer, mastering this feature will undoubtedly enhance your Python skills.
Understanding the Syntax of Inline if…else
The inline if…else statement in Python follows a simple syntax:
value_if_true if condition else value_if_false
In this structure, condition
is evaluated first. If it’s true, value_if_true
is returned; otherwise, value_if_false
is returned. This compact form can replace traditional multi-line if…else statements, making your code cleaner and more efficient.
Let’s see a basic example to clarify this concept.
age = 18
status = "Adult" if age >= 18 else "Minor"
print(status)
Output:
Adult
In this example, we check if the age
is greater than or equal to 18. If true, the variable status
is assigned the value “Adult”; if false, it gets “Minor”. The inline if…else statement allows us to achieve this in a single line, enhancing both readability and efficiency.
Practical Examples of Inline if…else
Now that we understand the basic syntax, let’s explore some practical examples of using inline if…else statements in different scenarios. These examples will help you appreciate the versatility and utility of this feature in Python programming.
Example 1: Assigning Values Based on Conditions
One common use of inline if…else statements is to assign values based on certain conditions. Here’s a simple case where we determine whether a number is positive, negative, or zero.
number = -5
result = "Positive" if number > 0 else "Negative" if number < 0 else "Zero"
print(result)
Output:
Negative
In this example, we check if number
is greater than zero. If it is, result
is assigned “Positive”. If not, we check if it’s less than zero to assign “Negative”, and if neither condition is met, we assign “Zero”. This compact form allows you to handle multiple conditions without cluttering your code.
Example 2: Conditional Function Calls
Inline if…else statements can also be useful when deciding which function to call based on a condition. Let’s consider a scenario where we want to greet users differently based on their logged-in status.
is_logged_in = True
greeting = "Welcome back!" if is_logged_in else "Please log in."
print(greeting)
Output:
Welcome back!
In this example, we check the is_logged_in
variable. If it’s true, the greeting will be “Welcome back!”, otherwise, it will prompt the user to log in. This demonstrates how inline if…else can streamline decisions in your code.
Example 3: Simplifying List Comprehensions
Another powerful application of inline if…else is within list comprehensions. This allows you to create lists in a more concise manner. For instance, let’s say we want to create a list of labels based on a list of scores.
scores = [85, 42, 67, 90]
labels = ["Pass" if score >= 50 else "Fail" for score in scores]
print(labels)
Output:
['Pass', 'Fail', 'Pass', 'Pass']
In this example, we iterate through each score in the scores
list. Using an inline if…else statement, we determine whether each score results in a “Pass” or “Fail” label. This method not only makes the code cleaner but also more efficient.
Conclusion
The inline if…else statement in Python is a valuable feature that enhances code readability and efficiency. By allowing you to condense conditional logic into a single line, it streamlines your coding process and helps you maintain cleaner code. Whether you’re assigning values based on conditions, deciding between function calls, or simplifying list comprehensions, mastering this feature will undoubtedly elevate your Python programming skills. Embrace the inline if…else statement in your coding practices, and watch your code become more elegant and efficient.
FAQ
-
What is an inline if…else statement in Python?
An inline if…else statement is a concise way to write conditional expressions in a single line, allowing you to return one of two values based on a condition. -
How does the syntax of inline if…else work?
The syntax is:value_if_true if condition else value_if_false
. If the condition is true, the first value is returned; otherwise, the second value is returned. -
Can I use inline if…else in list comprehensions?
Yes, inline if…else statements can be used in list comprehensions to create lists based on conditions, making your code cleaner and more efficient. -
Are there any limitations to using inline if…else?
While inline if…else statements are great for simple conditions, they can reduce readability for complex logic. It’s best to use them judiciously. -
How can inline if…else improve code readability?
By condensing conditional logic into a single line, inline if…else statements reduce clutter and make it easier to understand the flow of your code at a glance.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn