if not Statement in Python
- What is the If Not Statement?
- Using If Not with Boolean Values
- Combining If Not with Other Conditions
- Using If Not with Lists
- Conclusion
- FAQ
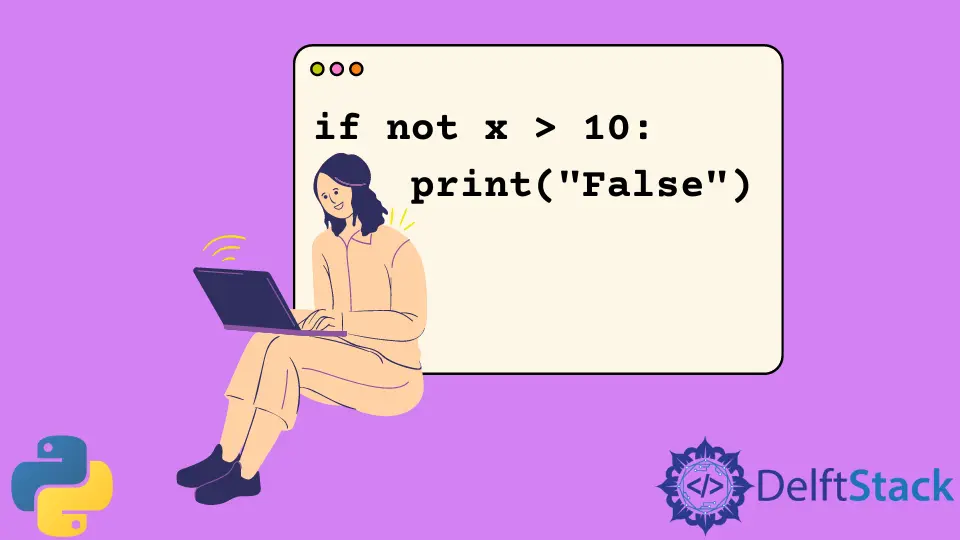
In Python programming, control flow is essential for creating dynamic and responsive applications. One of the fundamental components of control flow is the if
statement, which allows you to execute code based on certain conditions. However, sometimes you may want to reverse the logic of a condition, and that’s where the if not
statement comes into play.
This tutorial will explore the use of the if not
statement in Python, providing you with clear examples and explanations. Whether you’re a beginner looking to grasp the basics or an experienced developer wanting to refine your skills, this guide will help you understand how to effectively use if not
in your Python code.
What is the If Not Statement?
The if not
statement in Python is a way to check if a condition is false. Instead of writing a condition that evaluates to true, you can use if not
to execute code when the condition is false. This can lead to cleaner and more readable code, especially in complex logical structures.
Example of If Not Statement
Let’s take a simple example to illustrate how the if not
statement works. Suppose we want to check if a variable x
is not equal to 10. If x
is indeed not equal to 10, we will print a message indicating that.
x = 5
if not x == 10:
print("x is not equal to 10")
Output:
x is not equal to 10
In this example, x
is set to 5. The condition not x == 10
evaluates to true because 5 is indeed not equal to 10, so the message is printed. The if not
statement effectively reverses the logic of the equality check, making it straightforward to understand.
Using If Not with Boolean Values
Another common use case for the if not
statement is with boolean values. Let’s say we have a boolean variable that indicates whether a user is logged in or not. We can use the if not
statement to execute code when the user is not logged in.
Example of If Not with Boolean
Consider this example where we check if a user is logged in:
is_logged_in = False
if not is_logged_in:
print("User is not logged in")
Output:
User is not logged in
Here, the variable is_logged_in
is set to False
. The condition not is_logged_in
evaluates to true, triggering the print statement. This is a practical approach for handling user authentication in applications, allowing you to easily manage access based on the user’s login status.
Combining If Not with Other Conditions
The if not
statement can also be combined with other conditions to create more complex logic. For instance, you might want to check if a user is not logged in and if they are not an admin, to display a specific message.
Example of Combining Conditions
Let’s illustrate this with an example where we check both conditions:
is_logged_in = False
is_admin = False
if not is_logged_in and not is_admin:
print("Access denied. Please log in.")
Output:
Access denied. Please log in.
In this case, both conditions are checked using if not
. Since the user is neither logged in nor an admin, the message is printed. This allows for efficient handling of multiple conditions in a clean and readable way.
Using If Not with Lists
The if not
statement can also be useful when working with lists. For example, you might want to check if a list is empty before proceeding with an operation.
Example of If Not with Lists
Here’s how you can use if not
to check if a list is empty:
my_list = []
if not my_list:
print("The list is empty.")
Output:
The list is empty.
In this example, my_list
is an empty list. The condition not my_list
evaluates to true, leading to the print statement being executed. This is a great way to prevent errors that could arise from trying to access elements in an empty list.
Conclusion
The if not
statement is a powerful feature in Python that allows for more readable and efficient code. By using if not
, you can easily reverse conditions, manage boolean values, combine multiple checks, and handle lists effectively. Whether you’re developing applications or scripting simple tasks, mastering the if not
statement will enhance your programming skills. As you continue your Python journey, remember that clarity and simplicity in your code will always lead to better maintainability and understanding.
FAQ
-
What does the if not statement do in Python?
The if not statement checks if a condition is false and executes the associated code block if it is. -
Can I use if not with multiple conditions?
Yes, you can combine if not with other conditions using logical operators like and or or. -
How does if not work with lists?
If not can be used to check if a list is empty, allowing you to handle cases where you might try to access elements in an empty list. -
Is if not only for boolean values?
No, if not can be used with any expression that evaluates to a boolean value, including comparisons and lists. -
Can I nest if not statements in Python?
Yes, you can nest if not statements within each other to create more complex conditions.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn