Line Continuation in Python
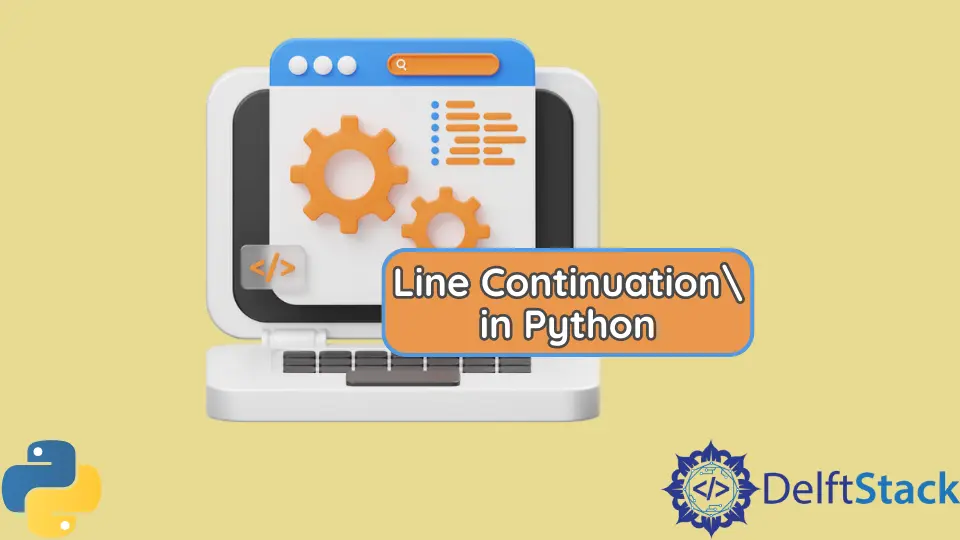
When it comes to writing code in Python, clarity and readability are paramount. One of the common challenges developers face is managing long lines of code. Fortunately, Python offers two primary methods for line continuation that can help maintain the neatness of your scripts. Understanding these techniques not only enhances your coding style but also makes it easier to debug and collaborate with others.
In this article, we will delve into these two methods, providing clear examples and explanations to ensure you grasp the concepts fully. Whether you’re a beginner or an experienced programmer, mastering line continuation in Python will undoubtedly improve your coding efficiency.
Method 1: Implicit Line Continuation
The first method of line continuation in Python is implicit line continuation. This technique allows you to split long lines of code into multiple lines without using any special characters. You can take advantage of parentheses, brackets, or braces to achieve this. Here’s how it works:
result = (1 + 2 + 3 +
4 + 5 + 6 +
7 + 8 + 9)
print(result)
Output:
45
In this example, the expression inside the parentheses is broken into multiple lines for better readability. Python recognizes that the line is not complete until the closing parenthesis is reached. This method is particularly useful for long mathematical expressions or when dealing with lists and dictionaries. By using implicit line continuation, you can keep your code clean and organized without sacrificing functionality.
Another advantage of this method is that it helps in maintaining the flow of logic in your code. When you use parentheses, brackets, or braces, it’s clear to anyone reading your code that the lines are part of the same statement. This makes collaboration easier, as others can quickly understand the structure and intent of your code.
Method 2: Explicit Line Continuation
The second method for line continuation in Python is explicit line continuation. This method requires the use of a backslash (\
) at the end of a line to indicate that the statement continues on the next line. Here’s an example:
total = 1 + 2 + 3 + \
4 + 5 + 6 + \
7 + 8 + 9
print(total)
Output:
45
In this case, the backslash tells Python that the line of code is not finished yet. While this method is straightforward, it’s essential to use it judiciously. The backslash can sometimes make the code look cluttered, especially if you have multiple lines of continuation. However, it does serve its purpose effectively when you want to break a long line without using parentheses or brackets.
One of the key points to remember when using explicit line continuation is that there should be no characters or spaces after the backslash. Any stray characters will lead to syntax errors, which can be frustrating for developers. Overall, while explicit line continuation may not be as visually appealing as implicit continuation, it remains a valuable tool in your coding arsenal.
Conclusion
In summary, mastering line continuation in Python is essential for writing clean and readable code. By utilizing implicit line continuation with parentheses, brackets, or braces, you can break long lines seamlessly. Alternatively, explicit line continuation with a backslash provides a straightforward way to manage lengthy statements. Both methods have their advantages, and knowing when to use each can significantly improve your coding style. So, whether you’re working on a personal project or collaborating with a team, these techniques will help you maintain clarity and efficiency in your Python scripts.
FAQ
-
What is line continuation in Python?
Line continuation in Python refers to methods that allow you to split long lines of code into multiple lines for better readability. -
What are the two main methods of line continuation?
The two main methods are implicit line continuation using parentheses, brackets, or braces, and explicit line continuation using a backslash. -
When should I use implicit line continuation?
Use implicit line continuation when you have long mathematical expressions or data structures, as it keeps your code clean and organized. -
What are the drawbacks of explicit line continuation?
The main drawback is that it can make the code look cluttered, and any stray characters after the backslash will lead to syntax errors. -
Can I use both methods in the same code?
Yes, you can use both implicit and explicit line continuation methods in the same code, depending on your preference and the context.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn