How to Solve The AttributeError: __Enter__ in Python
-
the
AttributeError: __enter__
in Python -
Other Possible Causes and Fixes of
AttributeError: __enter__
in Python - Conclusion
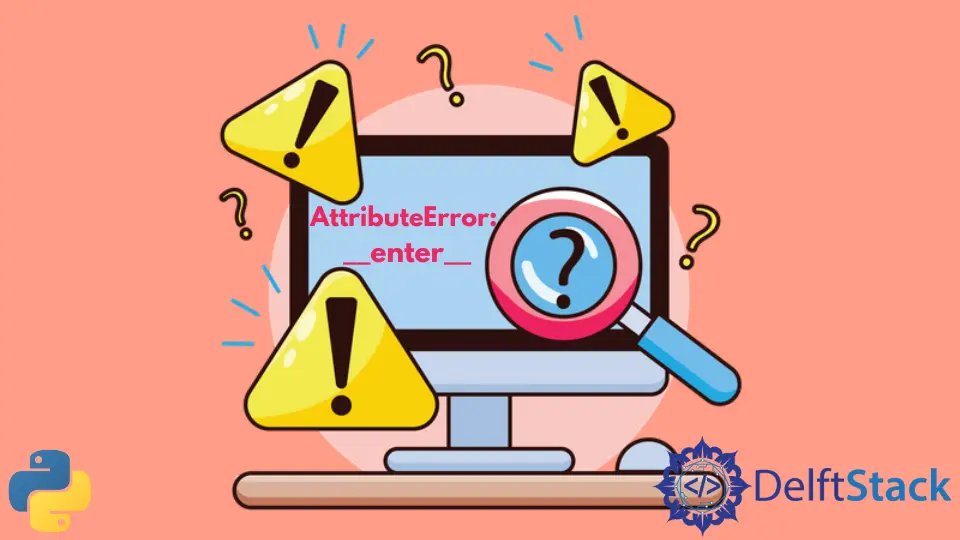
This error is caused by the fact that enter
is an attribute of a metaclass rather than built-in, which means it is not a valid attribute of an instance of the type.
In this article, we will discuss what this error is and how to fix it.
the AttributeError: __enter__
in Python
An AttributeError: __enter__
is a common Python error that indicates that a Python object has failed to instantiate with the expected class. The error usually occurs when a class hasn’t been imported correctly.
Also, it can occur if the user has forgotten to call the parent class in the class definition. This error will happen if the user fails to call the parent class when defining a child class.
How the AttributeError: __enter__
Occurs in Python
AttributeError: __enter__
is one of the most confusing error messages you can see in your Python program. It’s not a very clear error message and has many possible causes.
For instance, you can get it if you’re trying to use an object that doesn’t exist or if you’re trying to call a function that doesn’t exist on an object. It’s incredibly frustrating because the error message doesn’t tell you what object you tried to use or what function you tried to call.
It’s not a very helpful error message. The best way to know what is causing this error message is to use the debugger to track down where the error is happening.
Let’s say you want to use the object visitor
of a class DelfStack
inside a with
statement, then the object visitor
must have __exit__
and __enter__
; otherwise, this will produce an error. The __enter__
will be executed first, and the __exit__
will be executed at the end.
Let’s understand it through an example:
class DelfStack:
def __enter__(self):
print("Keep looking for solutions at DELFSTACK")
return "This is __Enter__, it will be executed first"
def __exit__(self, exc_type, exc_val, exc_tb):
print("This is __Exit__, this will be executed at the end")
visitor = DelfStack()
with visitor as obj:
print(obj)
Output:
Keep looking for solutions at DELFSTACK
This is __Enter__, it will be executed first
This is __Exit__, this will be executed at the end
But if we remove the __enter__
, the program will crash and throw an error.
class DelfStack:
# __enter is missing, this will throw an error
def __exit__(self, exc_type, exc_val, exc_tb):
print("This is __Exit__, this will be executed at the end")
visitor = DelfStack()
with visitor as obj:
print(obj)
Output:
----> 7 with visitor as obj:
8 print(obj)
AttributeError: __enter__
As you can see, the above program has thrown the error AttributeError: __enter__
because we are using the object with the with
statement, which demands the __enter__
and __exit__
. In this case, __enter__
is missing, which causes the AttributeError
.
Other Possible Causes and Fixes of AttributeError: __enter__
in Python
The AttributeError: __enter__
is usually caused by a Syntax Error in your Python code. It tells you that the Python interpreter has encountered a Syntax Error while trying to execute a Python statement.
The AttributeError: __enter__
can be due to any of the following reasons:
Compilation Error
In Python, an AttributeError
is a NameError
. When you encounter an AttributeError
, you’re trying to access an attribute on an object (through dot notation) that doesn’t have that attribute attached.
This is different from a TypeError
, which means you’re trying to call a function with the wrong type of arguments.
Syntax Error
The AttributeError: __enter__
error may occur when the programmer does not call the __enter__
method in a class. This gives an error because the program thinks the programmer is trying to call the method __enter__
, which is used in Python to call a class.
The __enter__
method is called when trying to enter a class. For example, if you want to enter a class named C
, you would type __enter__()
in C
.
However, Python thinks you are trying to call the __enter__
method. So, it returns the error AttributeError: __enter__
.
Runtime Error
The Runtime Error of AttributeError: __enter__
is one of the most common errors found while working with Python. This error usually occurs due to the failure of the exec
statement.
The AttributeError
occurs when a variable is not used correctly. This error is mainly generated if we have not used the variable after its declaration.
Import Error
AttributeError: __enter__
can’t set an attribute. In Python, you can use the import
statement’s simple form to define a module’s location; this will load the module into the interpreter.
You can then use the object in that module. Sometimes the error may be due to the Import Error.
Conclusion
AttributeError: __enter__
is a very annoying error that has bothered many users. If you run into this error, you can do a few things to resolve the issue.
First, you can try to import the package into the file you are trying to run. If you are running a Jupyter notebook, you can re-import the package you are having trouble with by using the drop-down menu next to the cell you are trying to run.
If you are getting an AttributeError: __enter__
when running a Python file, you may have forgotten to add the __enter__
method into your class.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python