Python Array Value Error
-
Resolve the
ValueError: only 2 non-keyword arguments accepted
in Python -
Resolve the
ValueError: setting an array element with a sequence
in Python - Matched Default Data-Type of Array and Values
- Conclusion
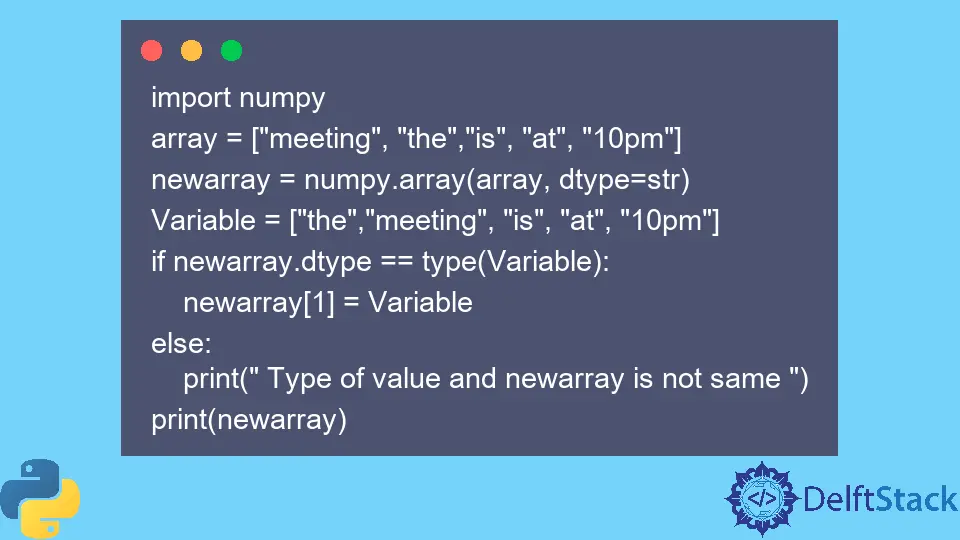
A Value Error
in Python happens when a function gets an argument of the right type, but the value of the type is incorrect. Another error occurs when the NumPy array has more than one element, which causes the error.
This article focuses on resolving specific ValueError
instances related to arrays in Python. We will address the only 2 non-keyword arguments accepted
error and the setting an array element with a sequence
error. Additionally, we’ll explore the concept of matching the default data type of an array with the provided values.
Resolve the ValueError: only 2 non-keyword arguments accepted
in Python
In the below example, the NumPy array is 2-dimensional, but afterward, we mixed in a single-dimensional array as well. The np.array()
function expects a single iterable (like a list) as its first argument.
Python recognizes this as an inhomogeneous shape, indicating that the array’s structure fluctuates, and hence Python raises a value error
.
Code Example:
import numpy as np
print(np.array([1, "English"], [2, "Spanish"], [3, "German"], dtype=object))
The code, import numpy as np
, imports the NumPy library and provides an alias (np
) for convenience.
Next, np.array([1, "English"], [2, "Spanish"], [3, "German"], dtype=object)
, creates a NumPy array. The outer square brackets []
represent a list, and the inner lists contain elements that can have different data types due to the dtype=object
parameter. This allows the array to hold a mix of integers and strings.
Lastly, print(data)
prints the resulting array to the console.
Output:
TypeError: array() takes from 1 to 2 positional arguments but 3 were given
The array should have identical elements to solve this error by creating the array with the same dimensions.
Code Example:
import numpy as np
print(np.array([[1, "English"], [2, "Spanish"], [3, "German"]], dtype=object))
In this corrected code, There are outer square brackets []
added which represent a list of lists, and the inner lists contain elements that can have different data types due to the dtype=object
parameter.
This allows the array to hold a mix of integers and strings.
Output:
[[1 'English']
[2 'Spanish']
[3 'German']]
The array above can hold a mix of different data types (integers and strings) because of the specified dtype=object
.
Keep in mind that using dtype=object
can have implications for performance, and in many cases, it’s preferable to work with homogeneous data types whenever possible.
Resolve the ValueError: setting an array element with a sequence
in Python
The ValueError: setting an array element with a sequence
occurs when you’re trying to assign a nested sequence (like a list containing another list) to an element of a NumPy array, and it violates the requirement of homogeneous data types within the array.
In the example below, the code is creating a NumPy array np_array
with a nested list [8, [10, 12]]
, which leads to the error.
Code Example:
import numpy
arrayeven = [2, 4, 6, [8, [10, 12]]]
np_array = numpy.array(arrayeven, dtype=int)
The code snippet is specifying dtype=int
, which means it is trying to create a NumPy array with integers.
However, due to the nested structure of arrayeven
, we will encounter the ValueError: setting an array element with a sequence
.
Output:
ValueError: setting an array element with a sequence
To resolve this, you should make sure that the elements of the NumPy array have consistent data types.
We can also use a data type that supports all data types (a common data type) to solve this error. In the following example, we’ve used dtype=object
instead of dtype=int
.
Code Example:
import numpy
arrayeven = [2, 4, 6, [8, [10, 12]]]
np_array = numpy.array(arrayeven, dtype=object)
print(np_array)
The arrayeven = [2, 4, 6, [8, [10, 12]]]
defines a Python list named arrayeven
. This list contains a mix of integers and nested lists.
Next, np_array = numpy.array(arrayeven, dtype=object)
uses the numpy.array
function to create a NumPy array from the Python list arrayeven
.
The dtype=object
parameter allows the NumPy array to contain elements of any Python object, effectively making it a heterogeneous array. This is useful when dealing with mixed data types or nested structures.
Output:
[2 4 6 list([8, [10, 12]])]
In this output, the NumPy array can hold integers as well as Python lists (which are nested within the original list).
The dtype=object
allows for this mixed data type behavior. Keep in mind that using dtype=object
can have performance implications, and in many cases, it’s preferable to work with homogeneous data types for better efficiency.
Matched Default Data-Type of Array and Values
In the below example, the error occurs due to the assignment of an array as an element of an array that accepts string data. This is related to mismatched data types when trying to assign a list to an element of a NumPy array.
Code Example:
import numpy
array = ["meeting", "the", "is", "at", "10pm"]
newarray = numpy.array(array, dtype=str)
newarray[1] = ["the", "meeting", "is", "at", "10pm"]
print(newarray)
The code begins by creating a NumPy array, newarray
, from a list of strings (array
).
The dtype=str
parameter specifies that the array elements should have a string data type. However, an error occurs when attempting to assign a list to the second element of newarray
, causing a mismatched data type.
The error prevents the successful execution of the code, and the attempt to print the resulting NumPy array using print(newarray)
is unsuccessful due to the encountered error.
Output:
ValueError: setting an array element with a sequence
We can solve this error by matching the data types of the value and the array. We may correct this issue and assign the value as an array element.
Code Example:
import numpy
array = ["meeting", "the", "is", "at", "10pm"]
newarray = numpy.array(array, dtype=str)
Variable = ["the", "meeting", "is", "at", "10pm"]
if newarray.dtype == type(Variable):
newarray[1] = Variable
else:
print(" Type of value and newarray is not same ")
print(newarray)
The code above involves creating a NumPy array, newarray
, from a list of strings, specifying a string data type.
A variable, Variable
, is defined as a list with the same elements. The code checks for data type compatibility between newarray
and Variable
.
If the data types match, the code correctly assigns the list to the second element of newarray
; otherwise, it prints an error message.
Finally, the code prints the resulting NumPy array, showing the modified array or the original array if there is a type mismatch.
Output:
Type of value and newarray is not same
['meeting' 'the' 'is' 'at' '10pm']
Using the library NumPy, we sometimes encounter Value Errors
. It happens when a function might pass the right argument type, but the value of that type is incorrect.
We can fix the Array Value Error
using the abovementioned methods.
Conclusion
This article addresses common ValueError
instances related to NumPy arrays in Python, focusing on specific error scenarios.
It covers the resolution of errors like only 2 non-keyword arguments accepted
and setting an array element with a sequence
. Additionally, the concept of matching the default data type of an array with the provided values is explored.
Practical examples demonstrate error cases and provide corrected code to ensure proper handling.
In conclusion, this article emphasizes the importance of understanding and resolving ValueError
instances when working with NumPy arrays in Python.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python