How to Print an Exception in Python
-
Print an Exception Using
try-except-finally
Blocks in Python -
Print Exception Using the
traceback
Module in Python
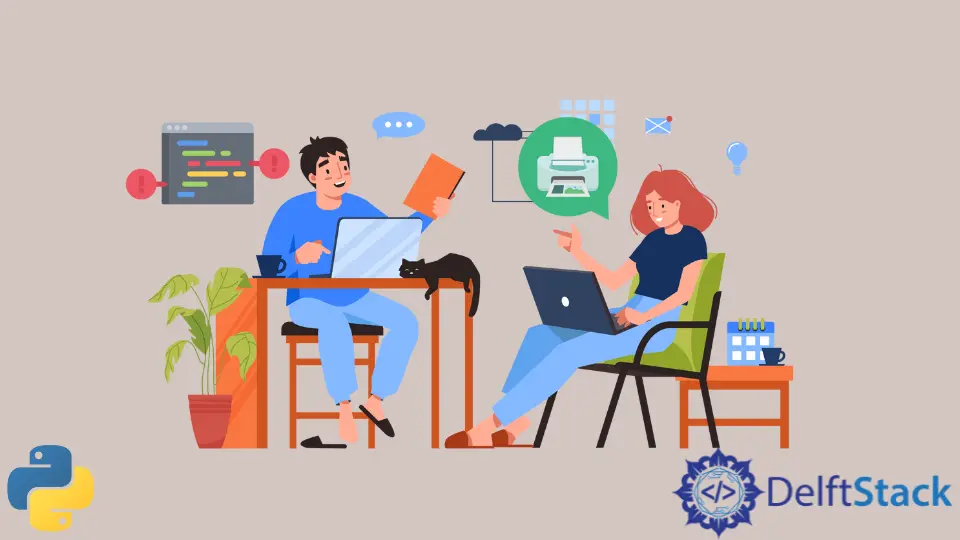
In Python, an Exception is an error. There are many errors or Exceptions in Python, such as TypeError
, SyntaxError
, KeyError
, AttributeError
, etc. We use try-except-finally
in Python to handle these Exceptions because, without these blocks, these exceptions would halt the program’s execution. try-except-finally
blocks in Python can be used to print these exceptions without stopping the program’s execution.
Print an Exception Using try-except-finally
Blocks in Python
Consider the following code snippet.
dictionary = {
"hello": "world",
}
number = 25
try:
number = number + dictionary["hello"]
print(number)
except Exception as e:
print(repr(e))
Output:
TypeError("unsupported operand type(s) for +: 'int' and 'str'",)
In the above code, we first initialize a dictionary with hello
as a key pointing to the string value world
, and a variable number
. Then inside the try
block, we are trying to access the string value stored in the dictionary
and adding it to the number
variable.
This statement is both practically and conceptually wrong because it is impossible to add a string
to an integer
. So the except
block catches this error and prints the Exception
object associated with this exception in the console.
Print Exception Using the traceback
Module in Python
Python has a built-in module, traceback
, for printing and formatting exceptions. And it makes it easy to print the whole exception in the console.
In Python, you can manually raise exceptions using the raise
keyword. In the following code snippet, we’ll use the raise
keyword to raise exceptions inside the try
block.
import traceback
try:
raise KeyError
except Exception as e:
traceback.print_exc()
Output:
Traceback (most recent call last):
File ".\main.py", line 4, in <module>
raise KeyError
KeyError
In the code above, we raise a KeyError
exception and use the print_exc()
function from the module traceback
to print the exception. This function prints the information about the exception and is a shorthand for traceback.print_exception(*sys.exc_info(), limit, file, chain)
.
To learn more about print_exception()
function refer to the official docs here