在 Python 中打印异常
Vaibhav Vaibhav
2023年1月30日
Python
Python Exception
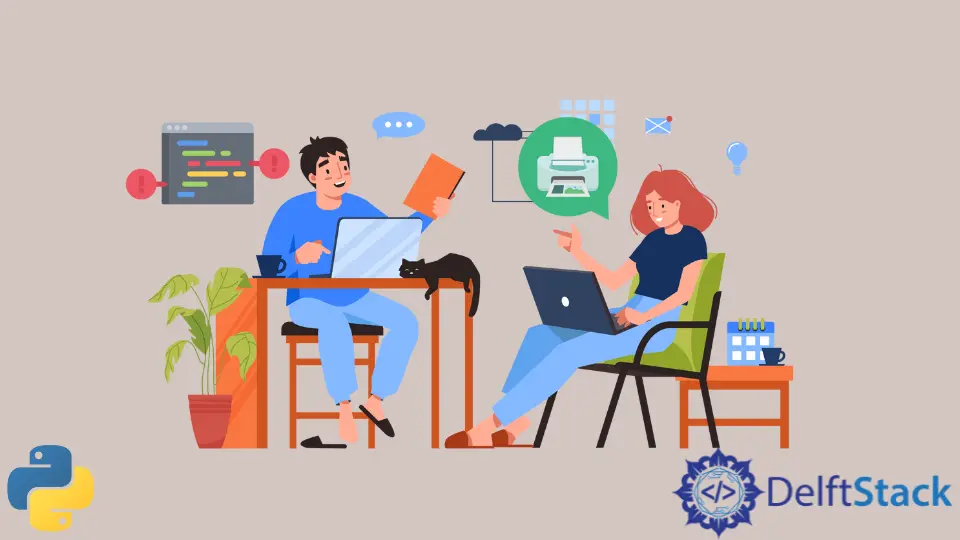
在 Python 中,异常是错误。Python 中存在许多错误或异常,例如 TypeError
、SyntaxError
、KeyError
和 AttributeError
等。我们在 Python 中使用 try-except-finally
来处理这些异常,因为没有这些块,这些异常将终止程序的执行。Python 中的 try-except-finally
块可用于打印这些异常,而无需停止程序的执行。
在 Python 中使用 try-except-finally
块打印异常
考虑以下代码片段。
dictionary = {
"hello": "world",
}
number = 25
try:
number = number + dictionary["hello"]
print(number)
except Exception as e:
print(repr(e))
输出:
TypeError("unsupported operand type(s) for +: 'int' and 'str'",)
在上面的代码中,我们首先用字典 hello
作为指向字符串值 world
的键和变量 number
的字典进行初始化。然后在 try
块中,我们尝试访问存储在字典
中的字符串值,并将其添加到 number
变量中。
该声明在实际上和概念上都是错误的,因为不可能在整数
中添加字符串
。因此,except
块会捕获此错误,并在控制台中输出与此异常关联的 Exception
对象。
在 Python 中使用 traceback
模块进行打印异常
Python 有一个内置模块 traceback
,用于打印和格式化异常。而且,它很容易在控制台中打印整个异常。
在 Python 中,你可以使用 raise
关键字手动引发异常。在以下代码段中,我们将使用 raise
关键字在 try
块内引发异常。
import traceback
try:
raise KeyError
except Exception as e:
traceback.print_exc()
输出:
Traceback (most recent call last):
File ".\main.py", line 4, in <module>
raise KeyError
KeyError
在上面的代码中,我们引发了一个 KeyError
异常,并使用了 traceback
模块中的 print_exc()
函数来打印该异常。该函数打印有关异常的信息,是 traceback.print_exception(*sys.ex_info(), limit, file, chain)
的简写。
要了解有关 print_exception()
函数的更多信息,请参考官方文档
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Vaibhav Vaibhav