Order of Operations in Python
- What is Operator Precedence?
- Basic Arithmetic Operators
- Using Parentheses for Clarity
- Comparison and Logical Operators
- Chaining Comparisons
- Conclusion
- FAQ
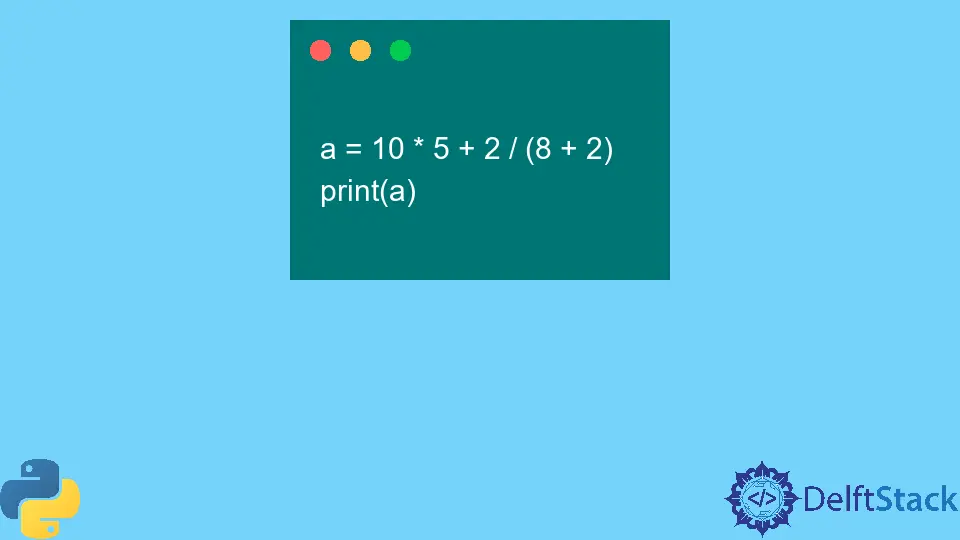
Understanding the order of operations in Python is crucial for anyone looking to write effective and efficient code. The order in which Python evaluates expressions can significantly affect your program’s behavior and output.
This tutorial will guide you through the fundamentals of operator precedence and how various operators interact with one another. Whether you’re a beginner or have some experience, grasping these concepts will empower you to write better code and debug more effectively. Let’s dive in and explore how different operators are executed in Python.
What is Operator Precedence?
In Python, operator precedence determines the order in which operations are performed in an expression. When you have multiple operators in a single expression, Python evaluates them based on their predefined precedence levels. Higher precedence operators are executed before lower precedence ones. For instance, multiplication and division have higher precedence than addition and subtraction.
To illustrate this, consider the following expression:
result = 3 + 4 * 2
Here, the multiplication operator (*) is executed before the addition operator (+). Thus, Python calculates:
Output:
result = 3 + (4 * 2) = 3 + 8 = 11
In this case, understanding operator precedence is essential for predicting the output accurately. If you are ever in doubt, using parentheses can clarify the order of operations, making your code more readable.
Basic Arithmetic Operators
Python supports several basic arithmetic operators, including addition (+), subtraction (-), multiplication (*), division (/), and modulus (%). Each of these operators has its own precedence level, which dictates the order of evaluation in expressions.
Let’s look at a simple example that combines these operators:
a = 10
b = 5
c = 2
result = a + b * c - a / b
In this code, the multiplication and division will be performed before addition and subtraction due to their higher precedence. The calculation will proceed as follows:
Output:
result = 10 + (5 * 2) - (10 / 5)
result = 10 + 10 - 2
result = 18
By following the order of operations, we see that the multiplication and division are executed first, leading to the final result of 18. This example highlights the importance of understanding how Python evaluates arithmetic expressions, particularly when combining multiple operators.
Using Parentheses for Clarity
While operator precedence is essential, using parentheses can help clarify your intentions and ensure that expressions are evaluated in the desired order. Parentheses can override the default precedence rules, allowing you to dictate the order in which operations are performed.
Consider the following example:
result = (a + b) * c - a / b
Here, the addition operation inside the parentheses is executed first:
Output:
result = (10 + 5) * 2 - (10 / 5)
result = 15 * 2 - 2
result = 30 - 2
result = 28
As you can see, the use of parentheses changed the outcome of the expression significantly. Instead of evaluating based on the default precedence, Python prioritized the addition, resulting in a final value of 28. This demonstrates how parentheses can be a powerful tool for controlling the flow of operations in your code.
Comparison and Logical Operators
In addition to arithmetic operators, Python also provides comparison and logical operators. Comparison operators (like ==, !=, >, <, >=, and <=) allow you to compare values, while logical operators (and, or, not) enable you to combine conditional statements. Understanding how these operators interact with one another is essential for writing effective conditionals and loops.
Let’s look at an example that combines comparison and logical operators:
a = 10
b = 5
c = 15
result = (a > b) and (c > a)
In this case, the comparisons are evaluated first due to their higher precedence:
Output:
result = (10 > 5) and (15 > 10)
result = True and True
result = True
The final output is True
since both comparisons are valid. This example illustrates how comparison operators take precedence over logical operators, allowing you to build complex conditional statements effectively.
Chaining Comparisons
Python allows you to chain comparisons, which can make your code more concise and readable. When chaining, Python evaluates the comparisons from left to right, applying the same precedence rules. This feature can be particularly useful in scenarios where you want to compare multiple values simultaneously.
Here’s an example of chaining comparisons:
a = 10
b = 5
c = 15
result = a > b > c
In this case, Python evaluates the expression as follows:
Output:
result = (10 > 5) > 15
However, since the first comparison evaluates to True
, Python interprets it as 1 > 15
, which is False
.
Output:
result = False
Chaining comparisons can simplify your code by reducing the number of logical operators required. However, it’s crucial to remember how Python evaluates these expressions to avoid unexpected results.
Conclusion
Understanding the order of operations in Python is fundamental for anyone looking to write effective code. By grasping operator precedence, using parentheses for clarity, and knowing how to work with comparison and logical operators, you can create more efficient and readable code. This knowledge not only helps you avoid bugs but also enhances your overall programming skills. As you continue to practice, you’ll find that mastering these concepts opens up new possibilities in your coding journey.
FAQ
-
What is operator precedence in Python?
Operator precedence in Python determines the order in which operators are evaluated in an expression. -
How can I change the order of operations in Python?
You can change the order of operations by using parentheses to group expressions as needed. -
Are there any operators that have the same precedence?
Yes, some operators, such as addition and subtraction, have the same precedence and are evaluated from left to right. -
What happens if I don’t use parentheses in complex expressions?
If you don’t use parentheses, Python will evaluate the expression based on its precedence rules, which may lead to unexpected results. -
Can I chain comparison operators in Python?
Yes, Python allows you to chain comparison operators, which can simplify your code while maintaining readability.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn